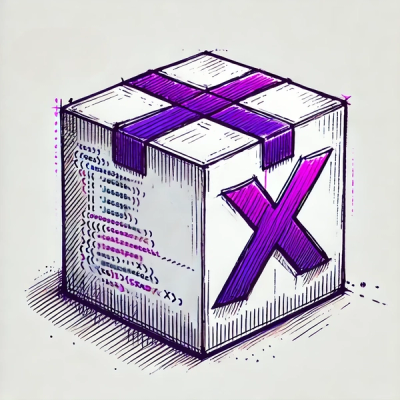
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
tweetnacl-util
Advanced tools
The tweetnacl-util npm package provides utility functions for encoding and decoding data to and from various formats, such as Base64 and UTF-8, which are commonly used in cryptographic operations. It is a companion to the tweetnacl package, which is a cryptographic library.
Encoding to Base64
This feature allows you to encode a UTF-8 string into a Base64 string. This is useful for encoding data that needs to be transmitted over media that are designed to deal with textual data.
const naclUtil = require('tweetnacl-util');
const message = 'Hello, World!';
const encodedMessage = naclUtil.encodeBase64(naclUtil.decodeUTF8(message));
console.log(encodedMessage);
Decoding from Base64
This feature allows you to decode a Base64 string back into a UTF-8 string. This is useful for decoding data that was encoded for safe transmission over text-based protocols.
const naclUtil = require('tweetnacl-util');
const encodedMessage = 'SGVsbG8sIFdvcmxkIQ==';
const decodedMessage = naclUtil.encodeUTF8(naclUtil.decodeBase64(encodedMessage));
console.log(decodedMessage);
Encoding to UTF-8
This feature allows you to encode a string into a UTF-8 byte array. This is useful for preparing data for cryptographic operations that require byte arrays.
const naclUtil = require('tweetnacl-util');
const message = 'Hello, World!';
const utf8Bytes = naclUtil.decodeUTF8(message);
console.log(utf8Bytes);
Decoding from UTF-8
This feature allows you to decode a UTF-8 byte array back into a string. This is useful for converting data back into a human-readable format after cryptographic operations.
const naclUtil = require('tweetnacl-util');
const utf8Bytes = new Uint8Array([72, 101, 108, 108, 111, 44, 32, 87, 111, 114, 108, 100, 33]);
const message = naclUtil.encodeUTF8(utf8Bytes);
console.log(message);
The base64-js package provides utilities for encoding and decoding data in Base64 format. It is similar to tweetnacl-util's Base64 encoding and decoding functionalities but does not include UTF-8 encoding and decoding.
The text-encoding package provides a polyfill for the TextEncoder and TextDecoder APIs, which are used for encoding and decoding text in various formats, including UTF-8. It offers similar functionality to tweetnacl-util's UTF-8 encoding and decoding features.
The buffer package provides a way to handle binary data in Node.js. It includes methods for encoding and decoding data in various formats, including Base64 and UTF-8. It offers a broader range of functionalities compared to tweetnacl-util.
String encoding utilities extracted from early versions of https://github.com/dchest/tweetnacl-js
Encoding/decoding functions in this package are correct, however their performance and wide compatibility with uncommon runtimes is not something that is considered important compared to the simplicity and size of implementation. For example, they don't work under React Native.
Instead of this package, I strongly recommend using my StableLib packages:
@stablelib/utf8 for UTF-8
encoding/decoding (note that the names of operations are reversed compared to
this package): npm install @stablelib/utf8
@stablelib/base64 for
constant-time Base64 encoding/decoding: npm install @stablelib/base64
Use a package manager:
$ bower install tweetnacl-util
NPM:
$ npm install tweetnacl-util
To make keep backward compatibility with code that used nacl.util
previously
included with TweetNaCl.js, just include it as usual:
<script src="nacl.min.js"></script>
<script src="nacl-util.min.js"></script>
<script>
// nacl.util functions are now available, e.g.:
// nacl.util.decodeUTF8
</script>
When using CommonJS:
var nacl = require('tweetnacl');
nacl.util = require('tweetnacl-util');
Decodes string and returns Uint8Array
of bytes.
Encodes Uint8Array
or Array
of bytes into string.
Decodes Base-64 encoded string and returns Uint8Array
of bytes.
Encodes Uint8Array
or Array
of bytes into string using Base-64 encoding.
FAQs
String encoding utilitlies extracted from TweetNaCl.js
The npm package tweetnacl-util receives a total of 742,841 weekly downloads. As such, tweetnacl-util popularity was classified as popular.
We found that tweetnacl-util demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.