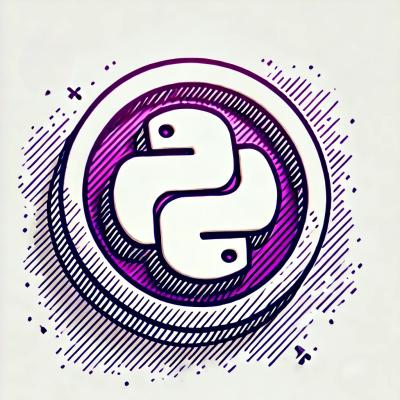
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The typanion npm package is a comprehensive solution for runtime input validation in JavaScript and TypeScript applications. It allows developers to ensure that the data their applications process meets certain criteria, thereby preventing unexpected errors and improving data integrity. Typanion offers a flexible and expressive API for defining validation rules, making it suitable for a wide range of use cases.
Basic Type Validation
This feature allows for basic type validation, such as checking if a value is a number. The code sample demonstrates how to use the `isNumber` function to validate numeric values.
{"const {isNumber} = require('typanion');
const validate = isNumber();
console.log(validate(42)); // {ok: true, errors: []}
console.log(validate('hello')); // {ok: false, errors: [...]}"}
Complex Object Validation
This feature enables complex object validation, allowing developers to validate nested objects and apply multiple validation rules. The code sample shows how to validate a user object with both `name` and `email` fields.
{"const {isObject, isString, applyCascade} = require('typanion');
const validateUser = isObject({
name: isString(),
email: applyCascade(isString(), [isEmail()])
});
console.log(validateUser({name: 'John Doe', email: 'john@example.com'})); // {ok: true, errors: []}"}
Custom Validation Rules
Typanion allows for the creation of custom validation rules. This feature is particularly useful for domain-specific validations. The code sample illustrates how to define and use a custom validator to check if a person is an adult.
{"const {createValidator} = require('typanion');
const isAdult = createValidator({
test: (value) => value >= 18,
message: (value) => `${value} is not an adult`
});
console.log(isAdult(21)); // {ok: true, errors: []}
console.log(isAdult(16)); // {ok: false, errors: [...]}"}
Joi is a powerful schema description language and data validator for JavaScript. It offers a similar range of functionalities for validating data structures but with a slightly different API design. Compared to typanion, Joi might be considered more feature-rich but also more complex for simple use cases.
Yup is a lean JavaScript schema builder for value parsing and validation. It integrates well with form libraries and is often used in front-end applications. While it provides similar validation capabilities, Yup focuses more on simplicity and ease of use compared to the more flexible and comprehensive approach of typanion.
Validator is a library of string validators and sanitizers. Unlike typanion, which offers a wide range of validation types and custom validation logic, Validator focuses primarily on string validation, making it more specialized but less versatile for different data types.
Static and runtime type assertion library with no dependencies
yarn add typanion
Compared to yup, Typanion has a better inference support for TypeScript + supports isOneOf
. Its functional API makes it very easy to tree shake, which is another bonus (although the library isn't very large in itself).
First define a schema using the builtin operators:
import * as t from 'typanion';
const isMovie = t.isObject({
title: t.isString(),
description: t.isString(),
});
Then just call the schema to validate any unknown
value:
const userData = JSON.parse(input);
if (isMovie(userData)) {
console.log(userData.title);
}
Passing a second parameter allows you to retrieve detailed errors:
const userData = JSON.parse(input);
const errors: string[] = [];
if (!isMovie(userData, {errors})) {
console.log(errors);
}
You can also apply coercion over the user input:
const userData = JSON.parse(input);
const coercions: Coercion[] = [];
if (isMovie(userData, {coercions})) {
// Coercions aren't flushed by default
for (const [p, op] of coercions) op();
// All relevant fields have now been coerced
// ...
}
You can derive the type from the schema and use it in other functions:
import * as t from 'typanion';
const isMovie = t.isObject({
title: t.isString(),
description: t.isString(),
});
type Movie = t.InferType<typeof isMovie>;
// Then just use your alias:
const printMovie = (movie: Movie) => {
// ...
};
Schemas can be stored in multiple variables if needed:
import * as t from 'typanion';
const isActor = t.isObject({
name: t.isString();
});
const isMovie = t.isObject({
title: t.isString(),
description: t.isString(),
actors: t.isArray(isActor),
});
isArray(values)
will ensure that the values are arrays whose values all match the specified schema.
isBoolean()
will ensure that the values are all booleans. Prefer isLiteral
if you wish to specifically check for one of true
or false
. This predicate supports coercion.
isDate()
will ensure that the values are proper Date
instances. This predicate supports coercion via either ISO8601, or raw numbers (in which case they're interpreted as the number of seconds since epoch, not milliseconds).
isDict(values, {keys?})
will ensure that the values are all a standard JavaScript objects containing an arbitrary number of fields whose values all match the given schema. The keys
option can be used to apply a schema on the keys as well (this will always have to be strings, so you'll likely want to use applyCascade(isString(), [...])
to define the pattern).
isLiteral(value)
will ensure that the values are strictly equal to the specified expected value. It's an handy tool that you can combine with oneOf
and object
to parse structures similar to Redux actions, etc.
isNumber()
will ensure that the values are all numbers. This predicate supports coercion.
isObject(props, {extra?})
will ensure that the values are plain old objects whose properties match the given shape. Extraneous properties will be aggregated and validated against the optional extra
schema.
isString()
will ensure that the values are all regular strings.
isUnknown()
will accept whatever is the input without validating it, but without refining the type inference either. Note that by default isUnknown
will forbid undefined
and null
, but this can be switched off by explicitly allowing them via isOptional
and isNullable
.
isInstanceOf(constructor)
will ensure that the values are instances of a given constructor.
applyCascade(spec, [specA, specB, ...])
will ensure that the values all match spec
and, if they do, run the followup validations as well. Since those followups will not contribute to the inference (only the lead schema will), you'll typically want to put here anything that's a logical validation, rather than a typed one (cf the Cascading Predicates section).
isOneOf([specA, specB])
will ensure that the values all match any of the provided schema. As a result, the inferred type is the union of all candidates. The predicate supports an option, exclusive
, which ensures that only one variant matches.
isOptional(spec)
will add undefined
as an allowed value for the given specification.
isNullable(spec)
will add null
as an allowed value for the given specification.
Cascading predicate don't contribute to refining the value type, but are handful to assert that the value itself follows a particular pattern. You would compose them using applyCascade
(cf the Examples section).
hasExactLength
will ensure that the values all have a length
property exactly equal to the specified value.
hasMaxLength
will ensure that the values all have a length
property at most equal to the specified value.
hasMinLength
will ensure that the values all have a length
property at least equal to the specified value.
hasUniqueItems
will ensure that the values only have unique items (map
will transform before comparing).
isAtLeast
will ensure that the values compare positively with the specified value.
isAtMost
will ensure that the values compare positively with the specified value.
isBase64
will ensure that the values are valid base 64 data.
isHexColor
will ensure that the values are hexadecimal colors (alpha
will allow an additional channel).
isInExclusiveRange
will ensure that the values compare positively with the specified value.
isInInclusiveRange
will ensure that the values compare positively with the specified value.
isInteger
will ensure that the values are round safe integers (unsafe
will allow unsafe ones).
isJSON
will ensure that the values are valid JSON, and optionally match them against a nested schema.
isLowerCase
will ensure that the values only contain lowercase characters.
isNegative
will ensure that the values are at most 0.
isPositive
will ensure that the values are at least 0.
isISO8601
will ensure that the values are dates following the ISO 8601 standard.
isUpperCase
will ensure that the values only contain uppercase characters.
isUUID4
will ensure that the values are valid UUID 4 strings.
matchesRegExp
will ensure that the values all match the given regular expression.
Validate that an unknown value is a port protocol:
const isPort = t.applyCascade(t.isNumber(), [
t.isInteger(),
t.isInInclusiveRange(1, 65535),
]);
isPort(42000);
Validate that a value contains a specific few fields, regardless of the others:
const isDiv = t.isObject({
tagName: t.literal(`DIV`),
}, {
extra: t.isUnknown(),
});
isDiv({tagName: `div`, appendChild: () => {}});
Validate that a specific field is a specific value, and that others are all numbers:
const isModel = t.isObject({
uid: t.String(),
}, {
extra: t.isDict(t.isNumber()),
});
isModel({uid: `foo`, valA: 12, valB: 24});
Copyright © 2020 Mael Nison
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Simple runtime TypeScript validator library
The npm package typanion receives a total of 646,754 weekly downloads. As such, typanion popularity was classified as popular.
We found that typanion demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.