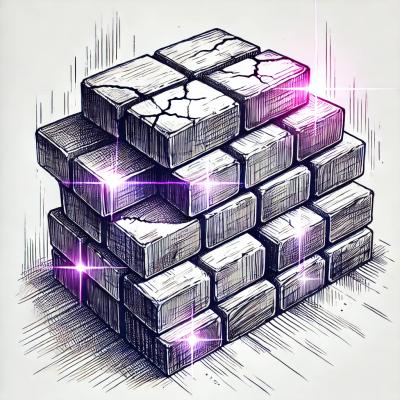
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
type-level-regexp
Advanced tools
TypeScript type-level RegExp parser and matcher implemented using template literals.
Examples in Playground
, test
🚧 🚧 Work In Progress, PRs and issues are welcome 🚧 🚧
type-level-regexp
dependency to your project# Using pnpm
pnpm i -D type-level-regexp
# Using npm
npm i -D type-level-regexp
createRegExp
function, pass in a RegExp string pattern to it creates a TypedRegExp
, passing this TypedRegExp
to String.match()
, String.matchAll()
or String.replace()
functions to get fully typed match result.match result will be fully typed if match against a literal stirng, or shows emumerated results if match against a dynamic string.
import { createRegExp, spreadRegExpIterator } from 'type-level-regexp'
/** string.match() */
const regExp = createRegExp('foO(?<g1>b[a-g]r)(?:BAz|(?<g2>qux))', ['i'])
const matchResult = 'prefix foobarbaz suffix'.match(regExp) // matching literal string
matchResult[0] // 'foobarbaz'
matchResult[1] // 'bar'
matchResult[3] // show type error `type '3' can't be used to index type 'RegExpMatchResult<...>`
matchResult.length // 3
matchResult.index // 7
matchResult.groups // { g1: "bar"; g2: undefined; }
/** string.replace() */
const regExp2 = createRegExp('(\\d{4})[-.](?<month>\\w{3,4})[-.](\\d{1,2})')
const replaceResult = '1991-Sept-15'.replace(regExp2, '$<month> $3, $1')
replaceResult // 'Sept 15, 1991'
/** string.matchAll() */
const regExp3 = createRegExp('c[a-z]{2}', ['g'])
const matchALlIterator = 'cat car caw cay caw cay'.matchAll(regExp3)
const spreadedResult = spreadRegExpIterator(matchALlIterator)
spreadedResult[2][0] // 'caw'
spreadedResult[3].index // 12
const InvalidRegExp = createRegExp('foo(bar')
// TypeScript error: Argument of type 'string' is not assignable to parameter of type 'RegExpSyntaxError<"Invalid regular expression, missing closing \`)\`">'
For TypeScript library authors, you can also import individual generic types to parse and match RegExp string at type-level and combine with your library's type-level features.
import { ParseRegExp, MatchRegExp } from 'type-level-regexp'
type MatchResult = MatchRegExp<'fooBAR42', 'Fo[a-z](Bar)\\d{2}', 'i'>
type Matched = MatchResult[0] // 'fooBAR42'
type First = MatchResult[1] // 'BAR'
type RegExpAST = ParseRegExp<'foo(?<g1>bar)'>
// [{
// type: "string";
// value: "foo";
// }, {
// type: "namedCapture";
// name: "g1";
// value: [{
// type: "string";
// value: "bar";
// }];
// }]
The main purpose of this project is to test and demonstrate the possibility and limitations of writing a RegExp parser/matcher in TypeScript's type-level. Note that this may not be practically useful, but rather an interesting showcase.
The idea for this project originated while I was working on improving the type hints of string.match and replace in magic-regexp (created by the most inspiring, resourceful, and kind Daniel Roe from Nuxt, definitely check it out if you are working with RegExp and TypeScript!).
As the complexity grows, I start working on this separated repo to increase development speed and try out different iterations. Hopefully, it can be ported back to magic-regexp, and even Gabriel Vergnaud's awesome hotscript when it's stable with good performance.
❤️ Testing, feedbacks and PRs are welcome!
createRegExp
function to create aTypedRegExp
that replace your original /regex_pattern/
regex object, which can be pass to String.match()
, String.matchAll()
and String.replace()
functions and gets fully typed result.RegExpSyntaxError
if the provided RegExp pattern is invalid.String
functions (.match
, matchAll
, .replace
...) for literal or dynamic typed string.String
functions matched exactly as runtime result.g
,i
) flags.spreadRegExpMatchArray
and spreadRegExpIterator
to get tuple type of match results and iterators.ParseRegExp
to parse and RegExp string to AST.MatchRegExp
to match giving string with a parsed RegExp.ResolvePermutation
to permutation all possible matching string of given RegExp if possible (due to TypeScript type-level limitation)string.replace()
string.matchAll()
with union of RegExp pattern remain as tupleTokens | Description | Support |
---|---|---|
. | Matches any single character. | ✅ |
* , *? | Matches zero or more occurrences (Greedy/Lazy). | ✅ |
+ , *? | Matches one or more occurrences (Greedy/Lazy). | ✅ |
? , ?? | Matches zero or one occurrence (Greedy/Lazy). | ✅ |
^ | Matches the start of a line. | ✅ |
$ | Matches the end of a line. | ✅ |
\s , \S | Matches any whitespace, non-whitespace character. | ✅ |
\d , \D | Matches any digit, non-digit character. | ✅ |
\w , \W | Matches any word, non-word character. | ✅ |
\b , \B | Matches a word-boundary, non-word-boundary. | ✅ |
[abc] | Matches any character in the set. | ✅ |
[^abc] | Matches any character not in the set. | ✅ |
() | Creates a capturing group. | ✅ |
(?:) | Creates a non-capturing group. | ✅ |
(?<name>) | Creates a named-capturing group. | ✅ |
| | Matches either the expression before or after the vertical bar. | ✅ |
{n} | Matches exactly n occurrences. | ✅ |
{n,} | Matches at least n occurrences. | ✅ |
{n,m} | Matches between n and m occurrences. | ✅ |
(?=) , (?!) | Positive/Negative lookahead. | ✅ |
(?<=) , (?<!) | Positive/Negative lookbehind. | ✅ |
Flags | Description | Support |
---|---|---|
g | Global matching (matches all occurrences). | ✅ |
i | Case-insensitive matching. | ✅ |
corepack enable
(use npm i -g corepack
for Node.js < 16.10)pnpm install
pnpm dev
Made with 🔥 and ❤️
Published under MIT License.
!-- Badges -->
FAQs
Type-Level RegExp parser, matcher and permutation resolver
The npm package type-level-regexp receives a total of 56,875 weekly downloads. As such, type-level-regexp popularity was classified as popular.
We found that type-level-regexp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.