What is typechain?
TypeChain is a tool for generating TypeScript typings for Ethereum smart contracts. It helps developers interact with smart contracts in a type-safe manner, reducing runtime errors and improving the development experience.
What are typechain's main functionalities?
Generating TypeScript typings for smart contracts
This command generates TypeScript typings for smart contracts using the Ethers.js target. The generated typings are placed in the 'src/types' directory, and the input JSON files are located in the 'artifacts' directory.
npx typechain --target ethers-v5 --out-dir src/types './artifacts/**/*.json'
Using generated typings in a TypeScript project
This code demonstrates how to use the generated TypeScript typings in a TypeScript project. It imports the typings for a contract named 'MyContract', interacts with the contract using Ethers.js, and logs the result of calling a method on the contract.
import { MyContract } from './types/MyContract';
async function main() {
const myContract: MyContract = await ethers.getContractAt('MyContract', '0x123...');
const value = await myContract.myMethod();
console.log(value);
}
Other packages similar to typechain
ethers
Ethers.js is a library for interacting with the Ethereum blockchain and its ecosystem. While it does not generate TypeScript typings for smart contracts, it provides a comprehensive set of tools for interacting with Ethereum, including contract interaction, wallet management, and more. TypeChain can generate typings specifically for use with Ethers.js.
web3
Web3.js is a collection of libraries that allow you to interact with a local or remote Ethereum node using HTTP, IPC, or WebSocket. Similar to Ethers.js, it does not generate TypeScript typings for smart contracts but provides a wide range of functionalities for interacting with the Ethereum blockchain. TypeChain can also generate typings for use with Web3.js.
truffle
Truffle is a development environment, testing framework, and asset pipeline for Ethereum. It provides a suite of tools for developing smart contracts, including compilation, deployment, and testing. While Truffle does not generate TypeScript typings, it can be used in conjunction with TypeChain to provide a complete development workflow.
Typechain
🔌 Typescript bindings for Ethereum smartcontracts
Features ⚡
- static typing - you will never call not existing method again
- IDE support - works with any IDE supporting Typescript
- revamped API - native promises, safety checks and more!
- compatibility - under the hood it uses web3 so it's 100% compatible
Demo 🏎️
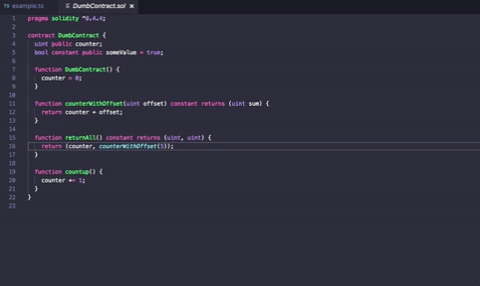
fullsize
Example repo
Getting started 📚
Motivation
Interacting with blockchain in Javascript is a pain. Web3 interface is sluggish and when you want to interact with a smartcontract already deployed it only gets worse. Often, you can't be sure what given method call will actually do without looking at ABI file. Typechain is here to solve these problems (as long as you use Typescript).
How does it work?
Typechain is just a code generator - provide ABI file and you will get Typescript class with flexible interface for interacting with blockchain.
In future we plan to leverage something like tsc plugin system to come up with much more elegant solution. You can keep track of Allow "Compiler Plugins" issue.
Step by step guide
Install typechain with yarn add --dev typechain
. Run typechain
(you might need to make sure that it's available in your path if you installed it only locally), it will automatically find all .abi
files in your project and generate Typescript classes based on them. We recommend git ignoring these generated files and making typechain part of your build process.
That's it! Now, just import contract bindings as any other file import MyAwesomeContract from './contracts/MyAwesomeContract'
and start interacting with it.
API
Let's take a look at typings generated for simple smartcontract:
interface ITxParams {
from?: string;
gas?: number | string | BigNumber;
gasPrice?: number | string | BigNumber;
}
declare class Contract {
public constructor(web3: any, address: string);
static createAndValidate(web3: any, address: string): Promise<Contract>;
public readonly someValue: Promise<boolean>;
public readonly counter: Promise<BigNumber>;
public returnAll(): Promise<[BigNumber, BigNumber]>;
public counterWithOffset(offset: BigNumber): Promise<BigNumber>;
public countupTx(params?: ITxParams): Promise<void>;
}
Roadmap 🛣️
- support for all solidity types
- transaction support
- improve generated code (autoformatting, more checks)