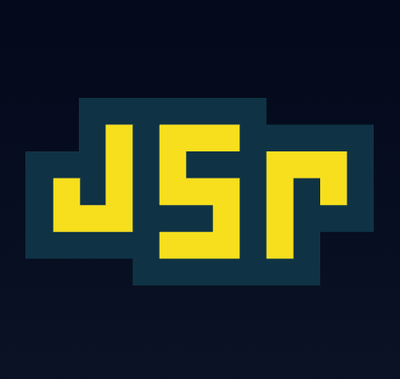
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Typeforce is a runtime type-checking library for JavaScript. It allows developers to enforce types and structures on their data, ensuring that the data conforms to expected formats. This can be particularly useful for validating function arguments, API responses, and more.
Basic Type Checking
Typeforce provides basic type checking for primitive types like strings, numbers, booleans, etc. The example demonstrates how to check if a value is a string.
const typeforce = require('typeforce');
// Define a type check for a string
const isString = typeforce.String;
// Validate a value
console.log(isString('Hello, World!')); // true
console.log(isString(123)); // throws an error
Custom Type Definitions
Typeforce allows you to define custom type checks using functions. The example shows how to create a custom type check for even numbers.
const typeforce = require('typeforce');
// Define a custom type check
const isEvenNumber = typeforce(function(value) {
return typeof value === 'number' && value % 2 === 0;
});
// Validate a value
console.log(isEvenNumber(4)); // true
console.log(isEvenNumber(5)); // throws an error
Object Structure Validation
Typeforce can validate the structure of objects, ensuring that they have the expected properties with the correct types. The example demonstrates how to validate an object representing a person.
const typeforce = require('typeforce');
// Define a type check for an object structure
const personType = {
name: typeforce.String,
age: typeforce.Number
};
// Validate an object
const person = { name: 'Alice', age: 30 };
console.log(typeforce(personType, person)); // true
const invalidPerson = { name: 'Bob', age: 'thirty' };
console.log(typeforce(personType, invalidPerson)); // throws an error
Array Type Checking
Typeforce can validate arrays, ensuring that all elements conform to a specified type. The example shows how to check if an array contains only numbers.
const typeforce = require('typeforce');
// Define a type check for an array of numbers
const isArrayofNumbers = typeforce(typeforce.ArrayOf(typeforce.Number));
// Validate an array
console.log(isArrayofNumbers([1, 2, 3])); // true
console.log(isArrayofNumbers([1, '2', 3])); // throws an error
Joi is a powerful schema description language and data validator for JavaScript. It allows you to create blueprints or schemas for JavaScript objects to ensure validation of key information. Compared to Typeforce, Joi offers a more extensive and flexible API for defining complex validation rules and is widely used in the Node.js community.
Yup is a JavaScript schema builder for value parsing and validation. It is similar to Joi but is often preferred for its simplicity and ease of use, especially in React applications. Yup provides a fluent API for object schema validation and is highly customizable.
Prop-types is a runtime type-checking library for React props. It allows you to specify the types of props that a component should receive, providing warnings in development mode if the props do not match the specified types. While it is more specialized for React, it offers similar type-checking capabilities as Typeforce.
Another biased type checking solution for Javascript.
Exception messages may change between patch versions, as often the patch will change some behaviour that was unexpected and naturally it results in a different error message.
var typeforce = require('typeforce')
var element = { prop: 'foo' }
var elementNumber = { prop: 2 }
var array = [element, element, elementNumber]
// supported primitives 'Array', 'Boolean', 'Buffer', 'Number', 'Object', 'String'
typeforce('Array', array)
typeforce('Number', array)
// TypeError: Expected Number, got Array
// array types
typeforce(['Object'], array)
typeforce(typeforce.arrayOf('Object'), array)
// supports recursive type templating
typeforce({ prop: 'Number' }, elementNumber)
// maybe types
typeforce('?Number', 2)
typeforce('?Number', null)
typeforce(typeforce.maybe(typeforce.Number), 2)
typeforce(typeforce.maybe(typeforce.Number), null)
// sum types
typeforce(typeforce.oneOf('String', 'Number'))
// value types
typeforce(typeforce.value(3.14), 3.14)
// custom types
function LongString (value, strict) {
if (!typeforce.String(value)) return false
if (value.length !== 32) return false
return true
}
typeforce(LongString, '00000000000000000000000000000000')
// => OK!
typeforce(LongString, 'not long enough')
// TypeError: Expected LongString, got String 'not long enough'
Protips:
// use precompiled primitives for high performance
typeforce(typeforce.Array, array)
// or just precompile a template
var type = {
foo: 'Number',
bar: '?String'
}
var fastType = typeforce.compile(type)
// fastType => typeforce.object({
// foo: typeforce.Number,
// bar: typeforce.maybe(typeforce.String)
// })
// use strictness for recursive types to enforce whitelisting properties
typeforce({
x: 'Number'
}, { x: 1 }, true)
// OK!
typeforce({
x: 'Number'
}, { x: 1, y: 2 }, true)
// TypeError: Unexpected property 'y' of type Number
WARNING: Be wary of using quacksLike
types which inherently rely on the MyType.name
property, if that property is mangled by say uglifyjs
you will have a bad time.
This library is free and open-source software released under the MIT license.
FAQs
Another biased type checking solution for Javascript
The npm package typeforce receives a total of 148,411 weekly downloads. As such, typeforce popularity was classified as popular.
We found that typeforce demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.