What is typia?
Typia is a TypeScript library that provides runtime type checking, validation, and serialization/deserialization capabilities. It aims to enhance type safety and runtime validation for TypeScript applications.
What are typia's main functionalities?
Runtime Type Checking
This feature allows you to perform runtime type checking to ensure that a value matches a specified TypeScript type. The code sample demonstrates how to check if a value is a string at runtime.
const isString = typia.is<string>(value);
Validation
Typia provides validation capabilities to ensure that data conforms to a specified type. The code sample shows how to validate an object against a TypeScript type, returning a result that indicates whether the data is valid.
const validationResult = typia.validate<MyType>(data);
Serialization/Deserialization
Typia can serialize TypeScript objects to JSON strings and deserialize JSON strings back to TypeScript objects, ensuring type safety throughout the process. The code sample demonstrates both serialization and deserialization.
const jsonString = typia.stringify<MyType>(data); const dataObject = typia.parse<MyType>(jsonString);
Other packages similar to typia
io-ts
io-ts is a runtime type system for IO decoding/encoding in TypeScript. It provides similar functionality to typia in terms of runtime type checking and validation. However, io-ts uses a functional programming approach and is more focused on decoding and encoding data.
zod
Zod is a TypeScript-first schema declaration and validation library. It offers similar validation and type-checking capabilities as typia but with a more declarative API for defining schemas. Zod is known for its simplicity and ease of use.
class-validator
Class-validator is a library for validating TypeScript class objects. It provides decorators for defining validation rules directly in class definitions. While it focuses on class-based validation, typia offers a broader range of type-related utilities.
Typia
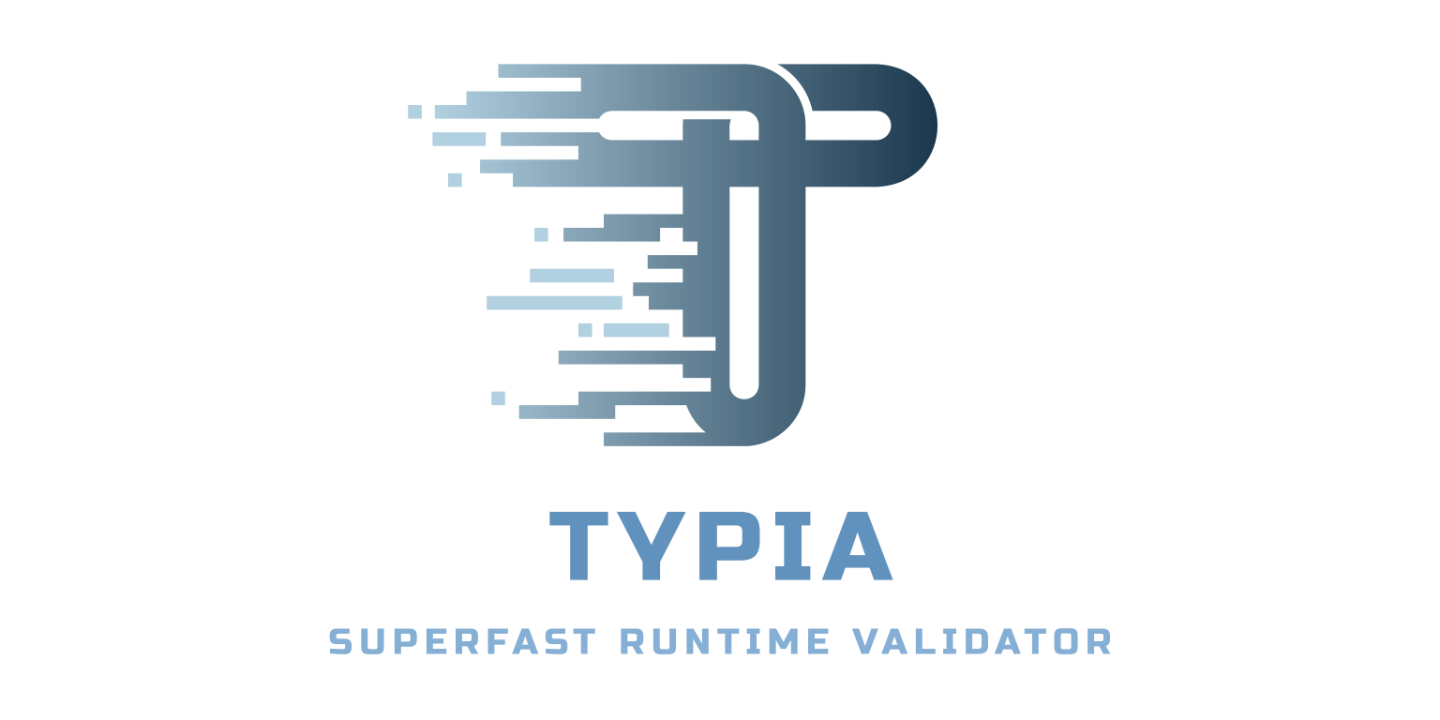

export function is<T>(input: unknown): input is T;
export function assert<T>(input: unknown): T;
export function assertGuard<T>(input: unknown): asserts input is T;
export function validate<T>(input: unknown): IValidation<T>;
export namespace json {
export function application<T>(): IJsonApplication;
export function assertParse<T>(input: string): T;
export function assertStringify<T>(input: T): string;
}
export namespace llm {
export function application<App>(): ILlmApplication;
export function schema<T>(): ILlmSchema;
}
export namespace protobuf {
export function message<T>(): string;
export function assertDecode<T>(buffer: Uint8Array): T;
export function assertEncode<T>(input: T): Uint8Array;
}
export function random<T>(g?: Partial<IRandomGenerator>): T;
Typia is a transformer library supporting below features:
- Super-fast Runtime Validators
- Enhanced JSON functions
- LLM function calling application composer
- Protocol Buffer encoder and decoder
- Random data generator
[!NOTE]
- Only one line required, with pure TypeScript type
- Runtime validator is 20,000x faster than
class-validator
- JSON serialization is 200x faster than
class-transformer
Thanks for your support.
Your donation encourages typia
development.
Also, typia
is re-distributing half of donations to core contributors of typia
.

Playground
You can experience how typia works by playground website:
Guide Documents
Check out the document in the website:
🏠 Home
📖 Features
🔗 Appendix