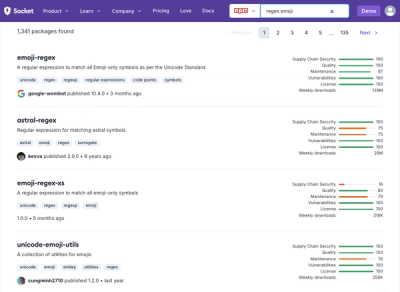
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
The uid2 npm package is a simple utility for generating unique IDs. It is often used in scenarios where you need to create unique tokens, session IDs, or any other form of unique identifier.
Generate Unique ID
This feature allows you to generate a unique ID of a specified length. In this example, a 32-character unique ID is generated.
const uid2 = require('uid2');
const id = uid2(32);
console.log(id);
The uuid package is a popular library for generating RFC4122 UUIDs (Universally Unique Identifiers). It offers more standardized and widely recognized unique ID generation compared to uid2.
The nanoid package is a small, secure, URL-friendly unique string ID generator. It is known for its performance and small size, making it a good alternative to uid2 for generating unique IDs.
The shortid package is used for generating short, non-sequential, URL-friendly unique IDs. It is useful when you need shorter IDs compared to those generated by uid2.
Generate unique ids. Pass in a length
and it returns a string
.
npm install uid2
Without a callback it is synchronous:
const uid = require('uid2');
const id = uid(10);
// id => "hbswt489ts"
With a callback it is asynchronous:
const uid = require('uid2');
uid(10, function (err, id) {
if (err) throw err;
// id => "hbswt489ts"
});
Imported via uid2/promises
it returns a Promise
:
const uid = require('uid2/promises');
async function foo() {
const id = await uid(10);
// id => "hbswt489ts"
}
MIT
FAQs
strong uid
The npm package uid2 receives a total of 860,158 weekly downloads. As such, uid2 popularity was classified as popular.
We found that uid2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.