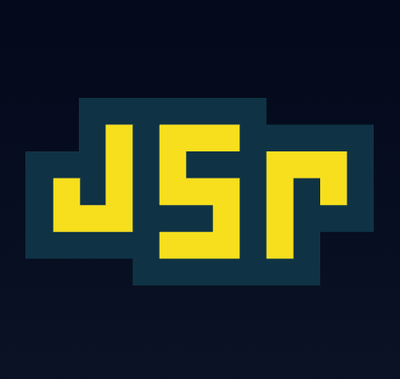
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
uint8arraylist
Advanced tools
Append and consume bytes using only no-copy operations
A class that lets you do operations over a list of Uint8Arrays without copying them.
import { Uint8ArrayList } from 'uint8arraylist'
const list = new Uint8ArrayList()
list.append(Uint8Array.from([0, 1, 2]))
list.append(Uint8Array.from([3, 4, 5]))
list.subarray()
// -> Uint8Array([0, 1, 2, 3, 4, 5])
list.consume(3)
list.subarray()
// -> Uint8Array([3, 4, 5])
// you can also iterate over the list
for (const buf of list) {
// ..do something with `buf`
}
list.subarray(0, 1)
// -> Uint8Array([0])
There are two ways to turn a Uint8ArrayList
into a Uint8Array
- .slice
and .subarray
and one way to turn a Uint8ArrayList
into a Uint8ArrayList
with different contents - .sublist
.
Slice follows the same semantics as Uint8Array.slice in that it creates a new Uint8Array
and copies bytes into it using an optional offset & length.
const list = new Uint8ArrayList()
list.append(Uint8Array.from([0, 1, 2]))
list.append(Uint8Array.from([3, 4, 5]))
list.slice(0, 1)
// -> Uint8Array([0])
Subarray attempts to follow the same semantics as Uint8Array.subarray with one important different - this is a no-copy operation, unless the requested bytes span two internal buffers in which case it is a copy operation.
const list = new Uint8ArrayList()
list.append(Uint8Array.from([0, 1, 2]))
list.append(Uint8Array.from([3, 4, 5]))
list.subarray(0, 1)
// -> Uint8Array([0]) - no-copy
list.subarray(2, 5)
// -> Uint8Array([2, 3, 4]) - copy
Sublist creates and returns a new Uint8ArrayList
that shares the underlying buffers with the original so is always a no-copy operation.
const list = new Uint8ArrayList()
list.append(Uint8Array.from([0, 1, 2]))
list.append(Uint8Array.from([3, 4, 5]))
list.sublist(0, 1)
// -> Uint8ArrayList([0]) - no-copy
list.sublist(2, 5)
// -> Uint8ArrayList([2], [3, 4]) - no-copy
Borrows liberally from bl but only uses native JS types.
$ npm i uint8arraylist
<script>
tagLoading this module through a script tag will make it's exports available as Uint8arraylist
in the global namespace.
<script src="https://unpkg.com/uint8arraylist/dist/index.min.js"></script>
Licensed under either of
Unless you explicitly state otherwise, any contribution intentionally submitted for inclusion in the work by you, as defined in the Apache-2.0 license, shall be dual licensed as above, without any additional terms or conditions.
FAQs
Append and consume bytes using only no-copy operations
We found that uint8arraylist demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.