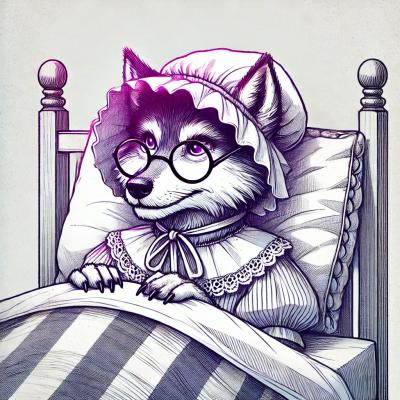
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The ulid npm package is a library for generating Universally Unique Lexicographically Sortable Identifiers (ULIDs). ULIDs are a type of unique identifier that are lexicographically sortable, making them useful for databases and other systems where ordering by time is important.
Generate a ULID
This feature allows you to generate a new ULID. The generated ULID is a 26-character string that is lexicographically sortable and unique.
const { ulid } = require('ulid');
const id = ulid();
console.log(id);
Monotonic ULID
This feature allows you to generate ULIDs in a monotonic manner, ensuring that they are always increasing even if generated in the same millisecond.
const { monotonicFactory } = require('ulid');
const ulid = monotonicFactory();
const id1 = ulid();
const id2 = ulid();
console.log(id1, id2);
The uuid package is a popular library for generating UUIDs (Universally Unique Identifiers). Unlike ULIDs, UUIDs are not lexicographically sortable but are widely used for their simplicity and uniqueness. UUIDs are typically used in distributed systems where uniqueness is critical.
The nanoid package is a library for generating unique IDs with a focus on performance and small size. Nanoid IDs are not lexicographically sortable but are very fast to generate and have a smaller footprint compared to ULIDs.
The shortid package is a library for generating short, unique, non-sequential IDs. While shortid IDs are not lexicographically sortable, they are useful for cases where a shorter ID is preferred and uniqueness is still required.
UUID can be suboptimal for many uses-cases because:
Instead, herein is proposed ULID:
npm install --save ulid
TypeScript, ES6+, Babel, Webpack, Rollup, etc.. environments
import { ulid } from 'ulid'
ulid() // 01ARZ3NDEKTSV4RRFFQ69G5FAV
CommonJS environments
const ULID = require('ulid')
ULID.ulid()
AMD (RequireJS) environments
define(['ULID'] , function (ULID) {
ULID.ulid()
});
Browser
<script src="/path/to/ulid.js"></script>
<script>
ULID.ulid()
</script>
To generate a ULID, simply run the function!
import { ulid } from 'ulid'
ulid() // 01ARZ3NDEKTSV4RRFFQ69G5FAV
You can also input a seed time which will consistently give you the same string for the time component. This is useful for migrating to ulid.
ulid(1469918176385) // 01ARYZ6S41TSV4RRFFQ69G5FAV
To generate monotonically increasing ULIDs, create a monotonic counter.
Note that the same seed time is being passed in for this example to demonstrate its behaviour when generating multiple ULIDs within the same millisecond
import { monotonicFactory } from 'ulid'
const ulid = monotonicFactory()
// Strict ordering for the same timestamp, by incrementing the least-significant random bit by 1
ulid(150000) // 000XAL6S41ACTAV9WEVGEMMVR8
ulid(150000) // 000XAL6S41ACTAV9WEVGEMMVR9
ulid(150000) // 000XAL6S41ACTAV9WEVGEMMVRA
ulid(150000) // 000XAL6S41ACTAV9WEVGEMMVRB
ulid(150000) // 000XAL6S41ACTAV9WEVGEMMVRC
// Even if a lower timestamp is passed (or generated), it will preserve sort order
ulid(100000) // 000XAL6S41ACTAV9WEVGEMMVRD
ulid
automatically detects a suitable (cryptographically-secure) PRNG. In the browser it will use crypto.getRandomValues
and on node it will use crypto.randomBytes
.
Math.random
By default, ulid
will not use Math.random
, because that is insecure. To allow the use of Math.random
, you'll have to use factory
and detectPrng
.
import { factory, detectPrng } from 'ulid'
const prng = detectPrng(true) // pass `true` to allow insecure
const ulid = factory(prng)
ulid() // 01BXAVRG61YJ5YSBRM51702F6M
To use your own pseudo-random number generator, import the factory, and pass it your generator function.
import { factory } from 'ulid'
import prng from 'somewhere'
const ulid = factory(prng)
ulid() // 01BXAVRG61YJ5YSBRM51702F6M
You can also pass in a prng
to the monotonicFactory
function.
import { monotonicFactory } from 'ulid'
import prng from 'somewhere'
const ulid = monotonicFactory(prng)
ulid() // 01BXAVRG61YJ5YSBRM51702F6M
Refer to ulid/spec
Refer to ulid/spec
npm test
npm run perf
ulid
336,331,131 op/s » encodeTime
102,041,736 op/s » encodeRandom
17,408 op/s » generate
Suites: 1
Benches: 3
Elapsed: 7,285.75 ms
FAQs
A universally-unique, lexicographically-sortable, identifier generator
The npm package ulid receives a total of 504,963 weekly downloads. As such, ulid popularity was classified as popular.
We found that ulid demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.