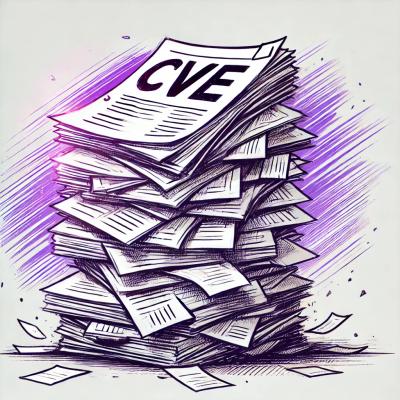
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
unisx
is a tiny (~512 bytes gzipped) React Native utility for Unistyles that simplifies the process of conditional styling. It allows you to apply a set of Unistyle styles based on certain conditions and component states, such as pressed
or hovered
by utilizings Unistyles dynamic functions feature. Unisx is loosely inspired by the clsx utility that conditionally concatenates classNames into a string, however this utility's syntax differs and returns an array of styles.
To add unisx
to your project, run the following command:
npm install unisx --save
# or
yarn add unisx
Import the unisx
function into your component:
import { unisx } from "unisx";
Define styles using Unistyles (see Unistyles docs)
const stylesheet = createStyleSheet((theme) => ({
defaultStyle: {
height: 50,
justifyContent: "center",
alignItems: "center",
borderRadius: 12,
backgroundColor: theme.colors.grey[11],
},
primary: ({ pressed }) => ({
backgroundColor: pressed ? theme.colors.grey[10] : theme.colors.grey[11],
}),
rounded: {
borderRadius: 25,
},
disabled: { backgroundColor: theme.colors.grey[7] },
}));
Pass your styles into Unistyle's useStyle hook like normal
const { styles } = useStyles(stylesheet);
Use unisx
to construct your styles:
const dynamicStyles = unisx({
styles, // This styles from the Unistyles useStyles hook
conditions: [
"defaultStyle", // A string means always applied
{ primary: true }, // Will apply styles.primary
{ rounded: true }, // Will apply styles.rounded
{ disabled: false }, // Will NOT apply styles.disabled
],
});
Apply the constructed styles to your components:
const MyComponent = () => {
return (
<View style={dynamicStyles}>
<Text>Dynamic Styles are Awesome!</Text>
</View>
);
};
export default MyComponent;
For components with state (e.g., Pressable
), you can pass the state object into the dynamicFunction feature of Unistyles:
const MyButton = (dynamicFunctionParams: PressableStateCallbackType) => {
const dynamicStyles = unisx({
styles,
dynamicFunctionParams, // Optional params to pass to Unistyles, great for passing in pressed / hovered states
conditions: [
"defaultStyle",
{ rounded: true },
{ primary: true },
{ disabled },
],
});
return (
<Pressable onPress={onPress} style={(state) => dynamicStyles(state)}>
<Text>Press Me</Text>
</Pressable>
);
};
export default MyButton;
import React from "react";
import { PropsWithChildren } from "react";
import {
Pressable,
PressableProps,
PressableStateCallbackType,
Text,
} from "react-native";
import { useStyles, createStyleSheet } from "react-native-unistyles";
import { unisx } from "unisx";
interface IButton extends PropsWithChildren<PressableProps> {
asChild?: boolean;
rounded?: boolean;
variant?: "primary" | "secondary";
disabled?: boolean;
onPress?: () => void;
}
export const Button = ({
children,
asChild,
rounded,
variant = "primary",
disabled,
onPress = () => null,
...rest
}: IButton) => {
const { styles } = useStyles(stylesheet);
const dynamicStyles = (dynamicFunctionParams: PressableStateCallbackType) =>
unisx({
styles,
dynamicFunctionParams,
conditions: [
"defaultStyle", // Always applies styles.defaultStyle
{ rounded: rounded === true }, // Applies styles.rounded if rounded (in this case a prop) is true.
{ primary: variant === "primary" }, // Applies styles.primary if variant (in this case a prop) equals 'primary'
{ secondary: variant === "secondary" }, // Applies styles.secondary if variant (in this case a prop) equals 'secondary'
{ disabled }, // Applies styles.disabled if disabled (in this case a prop) equals true
],
});
return (
<Pressable
onPress={() => !disabled && onPress()}
style={(state) => dynamicStyles(state)}
{...rest}
>
{asChild ? (
children
) : (
<Text style={styles.buttonText(disabled)}>{children}</Text>
)}
</Pressable>
);
};
const stylesheet = createStyleSheet((theme) => ({
defaultStyle: {
height: 50,
justifyContent: "center",
alignItems: "center",
borderRadius: 12,
backgroundColor: theme.colors.grey[11],
},
primary: ({ pressed }) => ({
backgroundColor: pressed ? theme.colors.grey[10] : theme.colors.grey[11],
}),
secondary: ({ pressed }) => ({
backgroundColor: pressed ? "green" : "red",
}),
disabled: { backgroundColor: theme.colors.grey[7] },
rounded: {
borderRadius: 25,
},
buttonText: (disabled?: boolean) => ({
color: disabled ? theme.colors.grey[9] : theme.colors.grey[1],
}),
}));
const dynamicStyles = unisx({
styles,
conditions: [
['style1,', 'style2']: variant === 'primary'}, // Proposed new optional syntax
{style1: variant === 'primary'}, // existing syntax
]
})
Note: Keys must be strings in Javascript objects. Maps can have keys of arrays, however I'm not sure how/if this could be made to work somehow using a map.
If you have suggestions for how unisx
could be improved, or want to report a bug, open an issue! Any and all contributions are welcome.
MIT © Mike Hamilton
FAQs
---
The npm package unisx receives a total of 8 weekly downloads. As such, unisx popularity was classified as not popular.
We found that unisx demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.