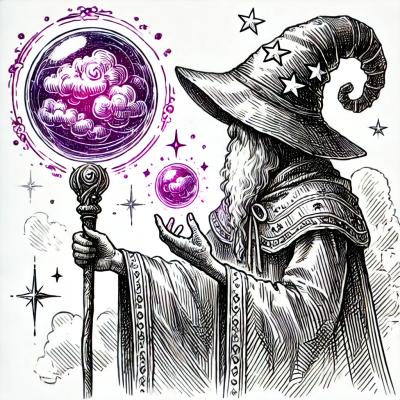
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
universal-tilt.js
Advanced tools
JavaScript & jQuery elements movement plugin based on:
Tilt.js by Gijs Rogé and vanilla-tilt.js by Șandor Sergiu
universal-tilt.js contains additional functions for mobile devices (having a gyroscope), new Position Base option and more!
At the beginning connect the library with Your project:
• using script tag in HTML:
<script src="/directory/to/library/folder/universal-tilt.js"></script>
• or via command line and CommonJS/ES6 import:
npm install universal-tilt.js // npm
yarn add universal-tilt.js // yarn
const UniversalTilt = require('universal-tilt.js'); // CommonJS
import UniversalTilt form 'universal-tilt.js'; // ES6
• Vanilla JavaScript e.g:
const tilts = document.querySelectorAll('.tilt');
const liveTilt = new UniversalTilt(tilts, {
// options...
});
• or jQuery e.g:
Connect jQuery in HTML
<script src="https://code.jquery.com/jquery-3.3.1.min.js"></script>
or include via command line and CommonJS
npm install jquery // npm
yarn add jquery // yarn
bower install jquery // bower
const jQuery = require('jquery');
and call plugin on element
$('.tilt').universalTilt({
// options...
});
• Plugin supports autoinit
To use it, add data-tilt
to html element e.g:
<div data-tilt></div>
Name | Type | Default | Description | Available options |
---|---|---|---|---|
position-base | string | element | The surface from which the location of the mouse is captured | element or window |
reset | boolean | true | Enable/disable element position reset after mouseout | true (enable), false (disable) |
mobile | boolean | true | Enable/disable tilt effect on mobile devices | true (enable), false (disable) |
shine | boolean | false | Add/remove shine effect on mouseover | true (add), false (remove) |
shine-opacity1 | number | 0 | Add/remove shine effect on mouseover | values >= 0 and <= 1 |
shine-save1 | boolean | false | Save/reset shine effect on mouseout | true (save), false (reset) |
max | number | 35 | Max tilt value | e.g: 28 |
perspective | number | 1000 | Tilt effect perspective | e.g: 700 |
scale | number | 1.0 | Element scale on mouseover | 0.9 /1.3 /etc. |
disabled | string | null | Disable axis | x or y |
reverse | boolean | false | Reverse tilt effect directory | true (reverse directory), false (standard directory) |
speed | number | 300 | Transition speed (ms) | e.g: 500 |
easing | string | cubic-bezier(.03,.98,.52,.99) | Transition easing | cubic-bezier /ease /linear /etc. |
onMouseEnter | function | null | Call function on mouse enter | el => { // code } |
onMouseMove | function | null | Call function on mouse move | el => { // code } |
onMouseLeave | function | null | Call function on mouse leave | el => { // code } |
onDeviceMove2 | function | null | Call function on device move | el => { // code } |
1 shine value must be true
2 mobile value must be true
This project is licensed under the MIT License
FAQs
Parallax tilt effect library
The npm package universal-tilt.js receives a total of 325 weekly downloads. As such, universal-tilt.js popularity was classified as not popular.
We found that universal-tilt.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.