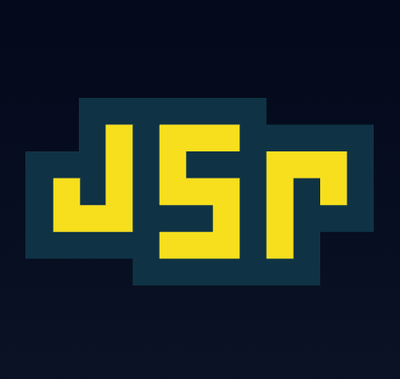
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The 'unstorage' npm package is a versatile storage utility that provides a unified API for interacting with various storage backends. It allows developers to easily switch between different storage mechanisms such as local storage, session storage, and even remote storage solutions without changing the core logic of their applications.
Local Storage
This feature allows you to interact with local storage using a simple API. You can set, get, and remove items from local storage.
const { createStorage } = require('unstorage');
const localStorage = createStorage();
// Set a value
await localStorage.setItem('key', 'value');
// Get a value
const value = await localStorage.getItem('key');
console.log(value); // Outputs: 'value'
// Remove a value
await localStorage.removeItem('key');
Session Storage
This feature allows you to interact with session storage using the same API as local storage. You can set, get, and remove items from session storage.
const { createStorage } = require('unstorage');
const sessionStorage = createStorage({ driver: 'session' });
// Set a value
await sessionStorage.setItem('key', 'value');
// Get a value
const value = await sessionStorage.getItem('key');
console.log(value); // Outputs: 'value'
// Remove a value
await sessionStorage.removeItem('key');
Remote Storage
This feature allows you to interact with remote storage solutions via HTTP. You can set, get, and remove items from a remote storage backend.
const { createStorage } = require('unstorage');
const remoteStorage = createStorage({ driver: 'http', baseURL: 'https://api.example.com/storage' });
// Set a value
await remoteStorage.setItem('key', 'value');
// Get a value
const value = await remoteStorage.getItem('key');
console.log(value); // Outputs: 'value'
// Remove a value
await remoteStorage.removeItem('key');
LocalForage is a fast and simple storage library for JavaScript. It improves the offline experience of your web app by using asynchronous storage (IndexedDB or WebSQL) with a simple, localStorage-like API. Compared to unstorage, LocalForage is more focused on client-side storage and does not provide a unified API for different storage backends.
idb-keyval is a small library that provides a simple key-value store backed by IndexedDB. It is very lightweight and easy to use, making it a good choice for simple storage needs. However, it lacks the flexibility and unified API of unstorage, which supports multiple storage backends.
store2 is a versatile storage library for all JavaScript environments, including Node.js and browsers. It provides a unified API for localStorage, sessionStorage, and memory storage. While it offers similar functionality to unstorage, it does not support remote storage solutions.
Unstorage provides an async Key-Value storage API with conventional features like multi driver mounting, watching and working with metadata, dozens of built-in drivers and a tiny core.
Install unstorage
npm package:
# yarn
yarn add unstorage
# npm
npm install unstorage
# pnpm
pnpm add unstorage
import { createStorage } from "unstorage";
const storage = createStorage(/* opts */);
await storage.getItem("foo:bar"); // or storage.getItem('/foo/bar')
👉 Check out the the documentation for usage information.
pnpm install
pnpm dev
to start jest watcher verifying changespnpm test
before pushing to ensure all tests and lint checks passingv1.13.0
FAQs
Universal Storage Layer
The npm package unstorage receives a total of 959,242 weekly downloads. As such, unstorage popularity was classified as popular.
We found that unstorage demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.