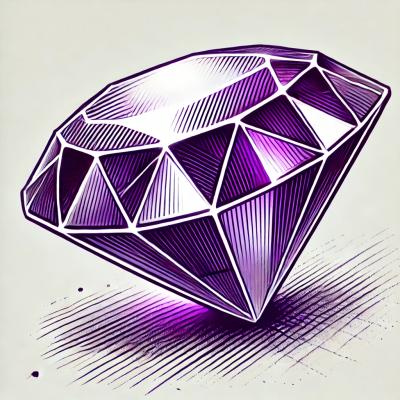
Security News
Highlights from the 2024 Rails Community Survey
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
urdf-loader
Advanced tools
Utilities for loading URDF files into THREE.js and a Web Component that loads and renders the model.
<script src=".../URDFLoader.js"></script>
<script>
const manager = new THREE.LoadingManager();
const loader = new URDFLoader(manager);
loader.load(
'T12/urdf/T12.URDF', // The path to the URDF within the package OR absolute
{
packageName : '.../package/dir/' // The equivelant of a (list of) ROS package(s):// directory
},
robot => { }, // The robot is loaded!
{
loadMeshCb: (path, ext, done) => { }, // Callback for each mesh for custom mesh processing and loading code
}
);
</script>
prismatic
, continuous
, revolute
, and fixed
joints are supported.Constructor
THREE.LoadingManager. Used for transforming load URLs.
Loads and builds the specified URDF robot in THREE.js
required
The path to the URDF file relative to the specified package directory.
required
The path representing the package://
directory(s) to load package://
relative files.
If the argument is a string, then it is used to replace the package://
prefix when loading geometry.
To specify multiple packages an object syntax is used defining the package name to the package path:
{
"package1": ".../path/to/package1",
"package2": ".../path/to/package2",
...
}
required
Callback that is called once the urdf robots have been loaded. The loaded robot is passed to the function.
See URDFRobot
documentation.
optional
An optional function that can be used to override the default mesh loading functionality. The default loader is specified at URDFLoader.defaultMeshLoader
. onComplete
is called with the mesh once the geometry has been loaded.
An optional object with the set of options to pass to the fetch
function call used to load the URDF file.
The path to load geometry relative to.
Defaults to the path relative to the loaded URDF file.
Parses URDF content and returns the robot model.
required
The xml content of a URDF file.
required
See load
.
optional
Called immediately with the generated robot. This is the same object that is returned from the function.
Note that the link geometry will not necessarily have finished being processed when this is called.
See URDFRobot
documentation.
See load
.
Object that describes the URDF Robot. An extension of THREE.Object3D
.
The name of the robot described in the <robot>
tag.
A dictionary of linkName : URDFLink
with all links in the robot.
A dictionary of jointName : URDFJoint
with all joints in the robot.
An object representing a robot joint. An extension of THREE.Object3D
.
The name of the joint.
The type of joint. Can only be the URDF types of joints.
An object containing the lower
and upper
constraints for the joint.
The axis described for the joint.
readonly
The current position or angle for joint.
Whether or not to ignore the joint limits when setting a the joint position.
The position off of the starting position to rotate or move the joint to.
The name of the link.
<!-- Register the Element -->
<script href=".../urdf-viewer-element.js" />
<script>customElements.define('urdf-viewer', URDFViewer)</script>
<body>
<urdf-viewer package=".../package/dir/" urdf="T12/urdf/T12.URDF" up="Z+" display-shadow ambient-color="red"></urdf-viewer>
</body>
Corresponds to the package
parameter in URDFLoader.load
. Supported are:
Single package:
// 1. Example for single package named `default_package`
<urdf-viewer package=".../path/to/default_package" ...></urdf-viewer>
Fallback within 1: If the target package within the package://
relative files do not match the default path it is assumed that the default path is the parent folder that contains the target package(s).
// 1. Example for single package named `default_package` with fallback:
<urdf-viewer package=".../path/to/parent" ...></urdf-viewer>
// since `parent` does not match `default_package`
// the path ".../path/to/parent/default_package" is assumed
Serialized package map:
E.g. if the meshes of a URDF are distributed over mutliple packages.
// 2. Example for serialized package map that contains `package1` and `package2`
<urdf-viewer package="package1:.../path/to/package1, package2:.../path/to/package1" ...></urdf-viewer>
Corresponds to the urdfpath
parameter in URDFLoader.load
.
The element uses fetch options { mode: 'cors', credentials: 'same-origin' }
to load the urdf file.
Whether or not hte display should ignore the joint limits specified in the model when updating angles.
The axis to associate with "up" in THREE.js. Values can be [+-][XYZ].
Whether or not the render the shadow under the robot.
The color of the ambient light specified with css colors.
Automatically redraw the model every frame instead of waiting to be dirtied.
Recenter the camera only after loading the model.
All of the above attributes have corresponding camel case properties.
Sets or gets the angles of the robot as a dictionary of joint-name
to radian
pairs.
Sets the given joint to the provided angle in radians.
Sets all joint names specified as keys to radian angle value.
Dirty the renderer so the element will redraw next frame.
Recenter the camera to the model and redraw.
Fires when the URDF has changed and a new one is starting to load.
Fires when the ignore-limits
attribute changes.
Fires when the URDF has finished loading and getting processed.
Fires when all the geometry has been fully loaded.
Install Node.js and NPM.
Run npm install
.
Run npm run server
.
Visit localhost:9080/javascript/example/
to view the page.
The software is available under the Apache V2.0 license.
Copyright © 2018 California Institute of Technology. ALL RIGHTS RESERVED. United States Government Sponsorship Acknowledged. Any commercial use must be negotiated with with Office of Technology Transfer at the California Institute of Technology. This software may be subject to U.S. export control laws. By accepting this software, the user agrees to comply with all applicable U.S. export laws and regulations. User has the responsibility to obtain export licenses, or other export authority as may be required before exporting such information to foreign countries or providing access to foreign persons. Neither the name of Caltech nor its operating division, the Jet Propulsion Laboratory, nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
FAQs
URDF Loader for THREE.js and webcomponent viewer
The npm package urdf-loader receives a total of 6,690 weekly downloads. As such, urdf-loader popularity was classified as popular.
We found that urdf-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A record 2,709 developers participated in the 2024 Ruby on Rails Community Survey, revealing key tools, practices, and trends shaping the Rails ecosystem.
Security News
In 2023, data breaches surged 78% from zero-day and supply chain attacks, but developers are still buried under alerts that are unable to prevent these threats.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.