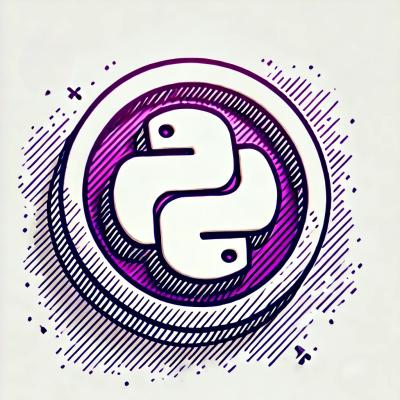
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
use-broadcast-ts
Advanced tools
Use the Broadcast Channel API in React easily with hooks and Typescript!
Use the Broadcast Channel API in React easily with hooks and Typescript!
npm install use-broadcast-ts
This package allows you to use the Broadcast API with a simple hook.
import { FC } from 'react';
import { useBroadcast } from 'use-broadcast-ts';
const MyComponent: FC = () => {
const {
state,
send,
} = useBroadcast<{ value: number }>('my-channel', { value: 0 })
return (
<>
<p>My value is: {state.value}</p>
<button onClick={() => send({value: 10})}/>
</>
);
};
export default MyComponent;
With the example above, the component will re-render when the channel receive or send a value.
import { FC, useEffect } from 'react';
import { useBroadcast } from 'use-broadcast-ts';
const MyComponent: FC = () => {
const {
send,
subscribe,
} = useBroadcast<{ value: number }>('my-channel', { value: 0 }, { subscribe: true })
useEffect(() => {
const unsub = subscribe(({value}) => console.log(`My new value is: ${value}`));
return () => unsub();
}, []);
return (
<>
<button onClick={() => send({value: 10})}/>
</>
);
};
export default MyComponent;
With the example above, the component will not re-render when the channel receive or send a value but will call the subscribe
callback.
useWasm<T>(name: string, value?: T, options?: UseBroadcastOptions): {
state: T;
send: (value: T) => void;
subscribe: (callback: (e: T) => void) => () => void;
};
Type: string
The name of the channel to use.
Type: T
(default: undefined
)
The initial value of the channel.
Type: UseBroadcastOptions
(default: {}
)
The options of the hook.
Type: boolean | undefined
(default: undefined
)
If true, the hook will not re-render the component when the channel receive a new value but will call the subscribe
callback.
Type: T
The current value of the channel.
Type: (value: T) => void
Send a new value to the channel.
Type: (callback: (e: T) => void) => () => void
Subscribe to the channel. The callback will be called when the channel receive a new value and when the options.subscribe is set to true.
You can send any of the supported types by the structured clone algorithm like :
String
Boolean
Number
Array
Object
Date
...
In short, you cannot send :
Function
Dom Element
See the MDN documentation for more information.
MIT
FAQs
Use the Broadcast Channel API in React easily with hooks or Zustand, and Typescript!
The npm package use-broadcast-ts receives a total of 2,123 weekly downloads. As such, use-broadcast-ts popularity was classified as popular.
We found that use-broadcast-ts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.