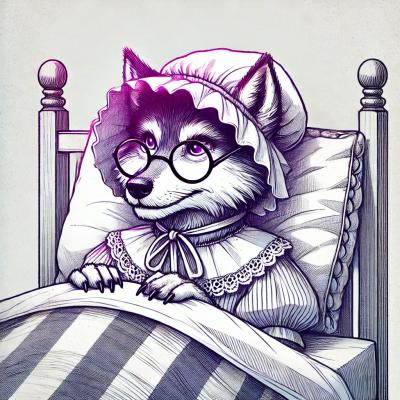
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
use-debounce
Advanced tools
The use-debounce package provides a collection of hooks and functions to debounce any fast-changing value in React applications. The main purpose is to limit the rate at which a function gets invoked. This is particularly useful for performance optimization in scenarios such as search input fields, where you might want to wait for a pause in typing before sending a request to a server.
useDebounce
This hook allows you to debounce any fast-changing value. The first parameter is the value to debounce, and the second parameter is the delay in milliseconds.
import { useDebounce } from 'use-debounce';
const [value] = useDebounce(text, 1000);
useDebouncedCallback
This hook gives you a debounced function that you can call. It will only invoke the provided function after the specified delay has elapsed since the last call.
import { useDebouncedCallback } from 'use-debounce';
const [debouncedCallback] = useDebouncedCallback(
() => {
// function to be debounced
},
1000
);
useThrottledCallback
Similar to debouncing, throttling is another rate-limiting technique. This hook provides a throttled function that will only allow one execution per specified time frame.
import { useThrottledCallback } from 'use-debounce';
const [throttledCallback] = useThrottledCallback(
() => {
// function to be throttled
},
1000
);
lodash.debounce is a method from the popular Lodash library. It provides debouncing functionality similar to use-debounce but is not a hook and does not have React-specific optimizations.
debounce is a minimalistic package that offers a debounce function. It is not tailored for React and does not provide hooks, but it can be used in any JavaScript application.
react-throttle offers components and hooks to throttle events or values in React applications. It is similar to use-debounce's throttling capabilities but focuses specifically on throttling rather than debouncing.
Install it with yarn:
yarn add use-debounce
Or with npm:
npm i use-debounce --save
The simplest way to start playing around with use-debounce is with this CodeSandbox snippet: https://codesandbox.io/s/kx75xzyrq7
More complex example with searching for matching countries using debounced input: https://codesandbox.io/s/rr40wnropq (thanks to https://twitter.com/ZephDavies)
According to https://twitter.com/dan_abramov/status/1060729512227467264
import React, { useState } from 'react';
import { useDebounce } from 'use-debounce';
export default function Input() {
const [text, setText] = useState('Hello');
const debouncedText = useDebounce(text, 1000);
return (
<div>
<input
defaultValue={'Hello'}
onChange={(e) => {
setText(e.target.value);
}}
/>
<p>Actual value: {text}</p>
<p>Debounce value: {debouncedText}</p>
</div>
);
}
10.0.4
FAQs
Debounce hook for react
The npm package use-debounce receives a total of 1,205,678 weekly downloads. As such, use-debounce popularity was classified as popular.
We found that use-debounce demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.