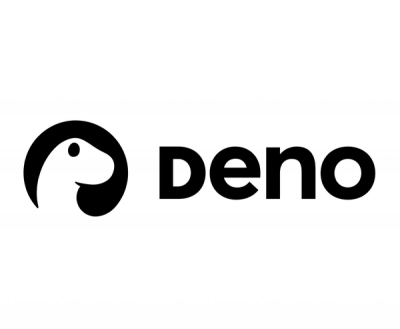
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
useful-object
Advanced tools
Typescript util to add useful methods to global Object, Array and more types.
Typescript util to add useful methods to global Object, Array and more types.
npm install useful-object --save
import "useful-object"; // 49.8K (gzipped: 11.8K)
....
const obj: any = {
name: {
firstName: "Avi",
lastName: "Punes"
}
};
obj.get("name.firstName"); // return "Avi"
interface MyInterface {
name: {
firstName: string;
lastName: string;
};
}
const obj: MyInterface = {
name: {
firstName: "Avi",
lastName: "Punes"
}
};
const firstName: string = obj.getSafe<MyInterface, string>(
obj => obj.name.firstName
); // Avi
const lastName: string = obj.getSafe((obj: MyInterface) => obj.name.lastName); // Punes
const firstName: string = await obj.get("name.firstName").toPromise(); // Avi
obj.get("name.firstName")
.toObservable()
.subscribe((firstName: string) => console.log(firstName)); // logs Avi
const firstName: string = await obj
.get("name.firstName")
.toPromise()
.delay(1000); // Avi after one second
const array = [1, 50, 3, 10];
array.subset("0..1"); // [1, 50]
array.subset("*..1"); // [1, 50]
array.subset("1..2"); // [50, 3]
array.subset("2..*"); // [3, 10]
array.subset("*..*"); // [1, 50, 3, 10]
const throwingFunc = () => {
throw "Some Error";
};
throwingFunc.attempt();
// console.error "Some Error"
// returns undefined
throwingFunc.attempt("Use this default value");
// console.error "Some Error"
// returns "Use this default value"
throwingFunc.attempt("Use this default value", () => {});
// returns "Use this default value"
const notThrowingFunc = () => {
return 5 * 3;
};
notThrowingFunc.attempt<number>(); // 15
npm test
FAQs
Typescript util to add useful methods to global Object, Array and more types.
The npm package useful-object receives a total of 7 weekly downloads. As such, useful-object popularity was classified as not popular.
We found that useful-object demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.