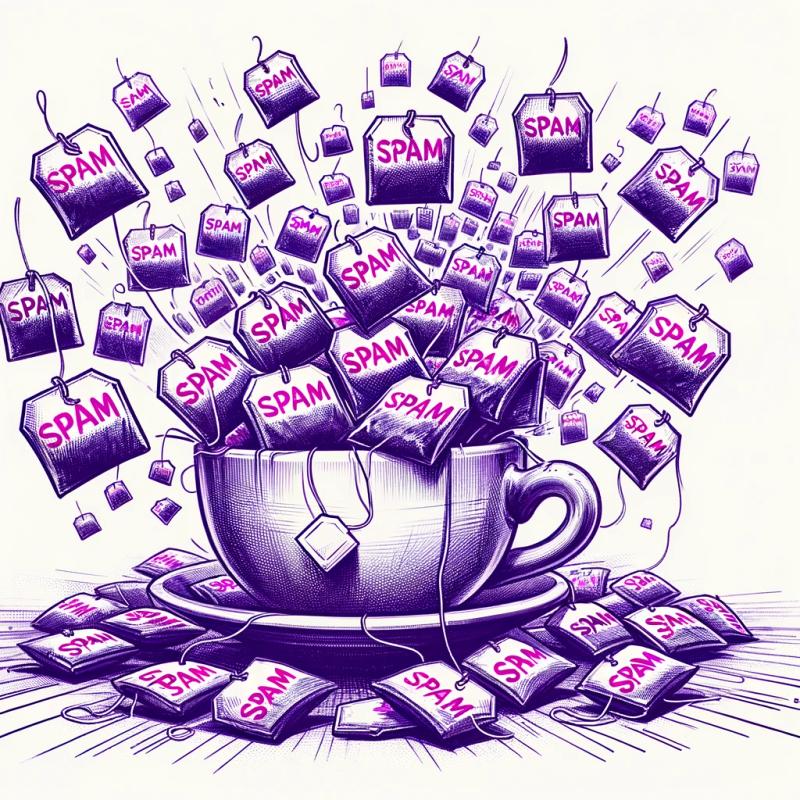
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
validate-value
Advanced tools
Readme
validate-value validates values against JSON schemas.
Category | Status |
---|---|
Version | |
Dependencies | |
Dev dependencies | |
Build | |
License |
$ npm install validate-value
The rewrite of validate-value
in version 9.0.0 follows the infamous quote "parse, don't validate"1. The quote asserts that parsing data is more valuable than validating it. This is very obvious when comparing the two approaches in TypeScript:
const doThingsWithValidation = async function (options: unknown): Promise<void> {
// This throws, if the options are not valid.
validateOptions(options);
const typedOptions = options as Options;
doSomethingWithAnOption(typedOptions.someOption);
};
const doThingsWithParsing = async function (options: unknown): Promise<Result<void, Error>> {
// This parses the options and unwraps them, throwing if they were invalid.
// If invalid options were something our program could handle, we would not
// want to throw here and instead handle the error appropriately.
// In this example we want the function to throw, if the options are invalid.
const typedOptions = parseOptions(options).unwrapOrThrow();
doSomethingWithAnOption(typedOptions.someOption);
};
In the second example, the typedOptions
contained in the Result
returned from the parseOptions
call already have the type that we expect them to have and we don't have to assert them or assign them to a new variable in any way. This combines better support from the TypeScript compiler with better error handling from defekt
.
First you need to integrate validate-value into your application:
const { parse, Parser } = require('validate-value');
If you use TypeScript, use the following code instead:
import { parse, Parser } from 'validate-value';
Then, create a new instance and provide a JSON schema that you would like to use for parsing:
const parser = new Parser({
type: 'object',
properties: {
username: { type: 'string' },
password: { type: 'string' }
},
additionalProperties: false,
required: [ 'username', 'password' ]
});
If you are using TypeScript, you will want to provide a type for the parsed value:
interface User {
username: string;
password: string;
}
const parser = new Parser<User>({
type: 'object',
properties: {
username: { type: 'string' },
password: { type: 'string' }
},
additionalProperties: false,
required: [ 'username', 'password' ]
});
Afterwards, you may use the parse
function to parse a value:
const user = {
username: 'Jane Doe',
password: 'secret'
};
const parseResult = parser.parse(user);
if (parseResult.hasError()) {
// The user object did not match the parser's schema. This should be handled.
} else {
// The user object was parsed successfully and the result's value can now be used.
doSomethingWithUser(parseResult.value);
}
After parsing, the value in the returned Result
will have the type User
, since it was passed to the parser upon construction.
For details on the error handling in the example above, check the documentation of the Result
type in defekt
.
By default, the error message uses value
as identifier and .
as the separator for the object that is parsed, but sometimes you may want to change this. Therefor, provide the desired identifier and separator as second parameter to the parse
function:
const user = {
username: 'Jane Doe',
password: 'secret'
};
const parseResult = parser.parse(user, { valueName: 'person', separator: '/' });
For convenience, there is also the parse
function, which skips the creation of a parser instance. You can use this if you're only going to use a schema for validation once. Otherwise, it is recommended to first create a parser instance, since then the JSON schema is only compiled once:
import { parse } from 'validate-value';
const parseResult = parse<User>(user, {
type: 'object',
properties: {
username: { type: 'string' },
password: { type: 'string' }
},
additionalProperties: false,
required: [ 'username', 'password' ]
});
To verify that a variable is of a specific type, use the isOfType
function. Hand over the value you would like to verify, and a JSON schema describing that type. The function returns true
if the given variable matches the schema, and false
if it doesn't:
const { isOfType } = require('validate-value');
const user = {
username: 'Jane Doe',
password: 'secret'
};
const schema = {
type: 'object',
properties: {
username: { type: 'string' },
password: { type: 'string' }
},
additionalProperties: false,
required: [ 'username', 'password' ]
};
if (isOfType(user, schema)) {
// ...
}
When using TypeScript, you may even specify a generic type parameter, and use the function as a type guard:
import { isOfType, JsonSchema } from 'validate-value';
interface User {
username: string;
password: string;
}
const user = {
username: 'Jane Doe',
password: 'secret'
};
const schema: JsonSchema = {
type: 'object',
properties: {
username: { type: 'string' },
password: { type: 'string' }
},
additionalProperties: false,
required: [ 'username', 'password' ]
};
if (isOfType<User>(user, schema)) {
// ...
}
1: "Parse, don't validate", Alexis King, 2019.
To run quality assurance for this module use roboter:
$ npx roboter
FAQs
validate-value validates values against JSON schemas.
The npm package validate-value receives a total of 434 weekly downloads. As such, validate-value popularity was classified as not popular.
We found that validate-value demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.