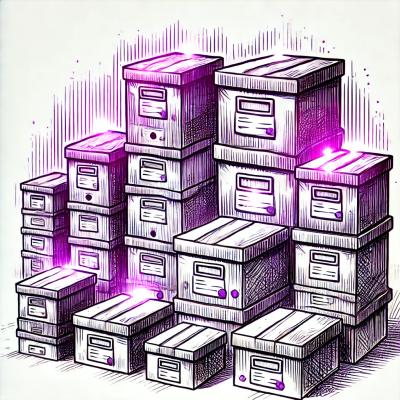
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
value-or-promise
Advanced tools
A thenable to streamline a possibly sync / possibly async workflow.
The 'value-or-promise' npm package is designed to handle values that could either be a direct value or a promise that resolves to a value. It provides utility functions to work with such values or promises in a consistent way, without having to check the nature of the value each time.
Handling values or promises uniformly
This feature allows you to handle both direct values and promises using the same API, which simplifies the code when the nature of the value is not known in advance or can vary.
const { ValueOrPromise } = require('value-or-promise');
function getValueOrPromise(value) {
return new ValueOrPromise(() => value)
.then(v => v)
.resolve();
}
// Usage with a direct value
getValueOrPromise(42).then(console.log); // 42
// Usage with a promise
getValueOrPromise(Promise.resolve(42)).then(console.log); // 42
Chaining operations
This feature allows chaining multiple operations on a value or promise, with each operation being applied as the previous one resolves. It's similar to promise chaining but works with non-promise values as well.
const { ValueOrPromise } = require('value-or-promise');
function chainOperations(value) {
return new ValueOrPromise(() => value)
.then(v => v * 2)
.then(v => v + 1)
.resolve();
}
// Usage with a direct value
chainOperations(10).then(console.log); // 21
// Usage with a promise
chainOperations(Promise.resolve(10)).then(console.log); // 21
Error handling
This feature provides a way to handle errors that may occur during the processing of a value or promise, similar to how errors are caught in promise chains.
const { ValueOrPromise } = require('value-or-promise');
function handleErrors(value) {
return new ValueOrPromise(() => value)
.then(v => {
if (v < 0) throw new Error('Negative value');
return v;
})
.catch(error => 'Error: ' + error.message)
.resolve();
}
// Usage with a direct value
handleErrors(-10).then(console.log); // Error: Negative value
// Usage with a promise
handleErrors(Promise.resolve(-10)).then(console.log); // Error: Negative value
Bluebird is a comprehensive promise library that provides a rich set of tools for working with promises. It offers features like promise cancellation, timeouts, and aggregation methods. Compared to 'value-or-promise', Bluebird focuses more on enhancing the promise API rather than abstracting over values and promises.
Pify is a module that promisifies callback-style functions. While it doesn't directly handle values or promises like 'value-or-promise', it does provide a way to work with asynchronous operations in a promise-like manner, which can then be used with 'value-or-promise' for uniform handling.
Co is a generator based flow-control utility that is primarily used with Node.js generator functions and promises. It allows you to yield any promise and it's similar to 'value-or-promise' in the sense that it abstracts away the handling of promises, but it uses a different approach based on generators.
A thenable to streamline a possibly sync / possibly async workflow.
yarn add value-or-promise
or npm install value-or-promise
Instead of writing:
function myFunction() {
const valueOrPromise = getValueOrPromise();
if (isPromise(valueOrPromise)) {
return valueOrPromise.then(v => onValue(v));
}
return onValue(valueOrPromise);
}
...write:
function myFunction() {
return new ValueOrPromise(getValueOrPromise)
.then(v => onValue(v))
.resolve();
}
When working with functions that may or may not return promises, we usually have to duplicate handlers in both the synchronous and asynchronous code paths. In the most basic scenario included above, using value-or-promise
already provides some code savings, i.e. we only have to reference doSomethingWithValue
once.
Things start to get even more beneficial when we add more sync-or-async functions to the chain.
Instead of writing:
function myFunction() {
const valueOrPromise = getValueOrPromise();
if (isPromise(valueOrPromise)) {
return valueOrPromise
.then(v => first(v))
.then(v => second(v));
}
const nextValueOrPromise = first(ValueOrPromise)
if (isPromise(nextValueOrPromise)) {
return nextValueOrPromise.then(v => second(v));
}
return second(nextValueOrPromise);
}
...write:
function myFunction() {
return new ValueOrPromise(getValueOrPromise)
.then(v => first(v))
.then(v => second(v))
.resolve();
}
Even with shorter chains, value-or-promise
comes in handy when managing errors.
Instead of writing:
function myFunction() {
try {
const valueOrPromise = getValueOrPromise();
if (isPromise(valueOrPromise)) {
return valueOrPromise
.then(v => onValue(v))
.catch(error => console.log(error));
}
const nextValueOrPromise = onValue(valueOrPromise);
if (isPromise(nextValueOrPromise)) {
return nextValueOrPromise
.catch(error => console.log(error));
}
return nextValueOrPromise;
} catch (error) {
console.log(error);
}
}
...write:
function myFunction() {
return new ValueOrPromise(getValueOrPromise)
.then(v => onValue(v))
.catch(error => console.log(error))
.resolve();
}
A simpler way of streamlining the above is to always return a promise.
Instead of writing:
function myFunction() {
const valueOrPromise = getValueOrPromise();
if (isPromise(valueOrPromise)) {
return valueOrPromise.then(v => onValue(v));
}
return onValue(valueOrPromise);
}
...or writing:
function myFunction() {
return new ValueOrPromise(getValueOrPromise)
.then(v => onValue(v))
.resolve();
}
...we could write:
function myFunction() {
return Promise.resolve(getValueOrPromise)
.then(v => onValue(v));
}
...but then we would always have to return a promise! If we are trying to avoid the event loop when possible, this will not suffice.
ValueOrPromise.all(...)?
We can use ValueOrPromise.all(...)
analogous to Promise.all(...)
to create a new ValueOrPromise
object that will either resolve to an array of values, if none of the passed ValueOrPromise
objects contain underlying promises, or to a new promise, if one or more of the ValueOrPromise
objects contain an underlying promise, where the new promise will resolve when all of the potential promises have resolved.
For example:
function myFunction() {
const first = new ValueOrPromise(getFirst);
const second = new ValueOrPromise(getSecond);
return ValueOrPromise.all([first, second]).then(
all => onAll(all)
).resolve();
}
myFunction
with return a value if and only if getFirst
and getSecond
both return values. If either returns a promise, myFunction
will return a promise. If both getFirst
and getSecond
return promises, the new promise returned by myFunction
will resolve only after both promises resolve, just like with Promise.all
.
The value-to-promise
concept is by Ivan Goncharov.
Implementation errors are my own.
1.0.12
FAQs
A thenable to streamline a possibly sync / possibly async workflow.
The npm package value-or-promise receives a total of 4,945,503 weekly downloads. As such, value-or-promise popularity was classified as popular.
We found that value-or-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.