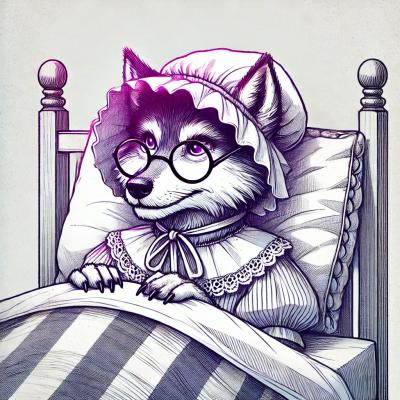
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The varint npm package is used for encoding and decoding variable-length integers. It is particularly useful in scenarios where you need to efficiently store or transmit integers that can vary greatly in size, such as in network protocols or file formats.
Encoding Integers
This feature allows you to encode an integer into a variable-length format. The encoded result is an array of bytes.
const varint = require('varint');
const encoded = varint.encode(300); // [172, 2]
console.log(encoded);
Decoding Integers
This feature allows you to decode a variable-length encoded integer back into its original integer form.
const varint = require('varint');
const decoded = varint.decode([172, 2]); // 300
console.log(decoded);
Encoding Integers to Buffer
This feature allows you to encode an integer directly into a Buffer, which can be useful for more advanced use cases involving binary data.
const varint = require('varint');
const buffer = Buffer.alloc(10);
const bytesWritten = varint.encode(300, buffer, 0); // 2
console.log(buffer.slice(0, bytesWritten));
Decoding Integers from Buffer
This feature allows you to decode an integer from a Buffer, which is useful when working with binary data streams.
const varint = require('varint');
const buffer = Buffer.from([172, 2]);
const decoded = varint.decode(buffer); // 300
console.log(decoded);
protobufjs is a comprehensive library for working with Protocol Buffers, which includes functionality for encoding and decoding variable-length integers. It is more feature-rich compared to varint, offering schema definitions and more complex data structures.
msgpack-lite is a library for encoding and decoding data in the MessagePack format, which includes support for variable-length integers. It is similar to varint but also supports a wider range of data types and is optimized for performance.
leb128 is a library for encoding and decoding LEB128 (Little Endian Base 128) integers, which is another form of variable-length integer encoding. It is similar to varint but uses a different encoding scheme.
FAQs
protobuf-style varint bytes - use msb to create integer values of varying sizes
The npm package varint receives a total of 0 weekly downloads. As such, varint popularity was classified as not popular.
We found that varint demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.