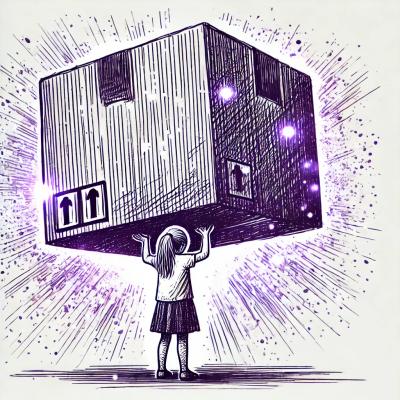
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
This repository contains a collection of React components used for developing new customer facing applications at Volvo Car Corporation.
This repository contains a collection of React components used for developing new customer facing applications at Volvo Car Corporation.
Furthermore, this library also exposes it's internal toolkit to enable you to build own custom React components using the same patterns.
You can view the components in action over at our storybook.
Please read the CONTRIBUTING.md document for more information on how to contribute to vcc-ui
Assuming you have a basic React project setup: Install the vcc-ui package via npm or Yarn.
npm install vcc-ui --save
Now wrap your top-level render method in the vcc-ui <StyleProvider>
higher order component (HOC). This will inline the CSS required to render the components being used.
import { StyleProvider } from 'vcc-ui'
import App from './app';
ReactDOM.render(
<StyleProvider><App/></StyleProvider>,
document.getElementById('root')
);
Now you can start using the components:
import React from 'react';
import { Block, CTA } from 'vcc-ui'
export default class App extends React.Component {
render() {
return (
<Block>
<CTA withArrow="right">Click me!</CTA>
</Block>
);
}
}
While the required CSS is automatically inlined via the <StyleProvider>
, assets like images and fonts are not. In order to use them you'll have to symlink (or copy) them from the vcc-ui package into your folder used for serving static files. As an example: For an app created using the create-react-app cli tool, it would look like this:
ln -s ./node_modules/vcc-ui/static ./public/vcc-ui
Components like the <Logo type="square">
will NOT work if you haven't done this.
The vcc-ui library has the following Volvo Car branded fonts included in the assets folder
Their names and paths are exposed via the fonts
module inside vcc-ui. Use the styleRenderer
and loadFonts
utility functions provided by vcc-ui:
import { StyleProvider, styleRenderer, loadFonts } from "vcc-ui";
const renderer = styleRenderer();
loadFonts({
fonts: ["Volvo Novum Light", "Volvo Broad"],
pathPrefix: "/static/",
fontDisplay: "block"
},
renderer
)
ReactDOM.render(
<StyleProvider renderer={renderer}><App /></StyleProvider>,
document.getElementById('root')
);
All components are compatible with the React API and will accept properties like any JSX or HTML element: E.g: onClick
or id
.
A block component is an unstyled <div>
, and a starting point for building your own custom block component
extend
- Object: Customize CSS properties for custom theming/styling of component (none
- default)as
- String: Define what HTML element should be used (div
- default)<Block
extend={{
color: 'red',
background: 'black'
}}
>
Hello
</Block>
An inline component is an unstyled <span>
, and a starting point for building your own custom inline component
extend
- Object: Customize CSS properties for custom theming/styling of component (none
- default)as
- String: Define what HTML element should be used (span
- default)<Inline>Hello</Inline>
withArrow
- String: up, down, or right (false
- default)style
- String: onDark, onLight (onLight
- default)secondary
: Boolean: apply secondary button styles (false
- default)fullWidth
: Boolean: let CTA width grow to parent container width (false
- default)<CTA secondary style="onDark" withArrow="right">Hello</CTA>
An unstyled component that can be used for creating your own clickable components. It's basically a native HTML button element with all the browser default styling removed. Read more about (buttons and acessbility)[https://developer.mozilla.org/en-US/docs/Web/Accessibility/ARIA/ARIA_Techniques/Using_the_button_role]
extend
- Object: Customize CSS properties for custom theming/styling of component (none
- default)<Click>I'm an unstyled clickable button</Click>
withArrow
- String: up, down, or right (false
- default)style
- String: onLight, onDark (onLight
- default)<Link withArrow="right">Hello</Link>
type
- Pick an icon from icons
constant (account
, search
, facebook
, twitter
, youtube
, linkedin
, instagram
, globe
)size
- s (12px), m (18px) or l (24px)<Icon type={icons.search} />
direction
- String: up, down, left, or right<Arrow direction="right" />
type
- String: square or wordmark (square
- default)<Logo type="square" />
hideOnScroll
- Boolean, (false
- default)sticky
- Boolean, (false
- default)<Nav>Hello</Nav>
dropdown
- Boolean, (false
- default)isACtive
- Boolean, (false
- default)<NavItem dropdown isActive>Hello</NavItem>
This module contains references to the icons available in vcc-ui
import { ICONS } from 'vcc-ui'
This module contains references to the brand colors available in vcc-ui
import { COLORS } from 'vcc-ui'
This module contains references to the margins in used vcc-ui
import { MARGINS } from 'vcc-ui'
This module contains references to the fonts used in vcc-ui
import { FONTS } from 'vcc-ui'
This module contains references to the font-sizes used in vcc-ui
import { FONT_SIZES } from 'vcc-ui'
import { BREAKPOINTS } from 'vcc-ui'
Breakpoints are currently:
Helpers available via BREAKPOINTS
:
example: untilM
is equal to @media (max-width: 768px)
example: fromL
is equal to @media (min-width: 1280px)
The styling engine behind vcc-ui is built on top of Fela - A small, high-performnt and framework-agnostic toolbelt to handle state-driven styling in JavaScript.
Use your own, or use the built breakpoints provided by vcc-ui to
import {Block, BREAKPOINTS} from 'vcc-ui'
<Block
extend={{
[BREAKPOINTS.untilL]: {
display: "none"
},
[BREAKPOINTS.fromL]: {
display: "flex",
}
}}
>
Hello
</Block>
You can use the renderer to specify arbitrary CSS or "reset" styles
renderer.renderStatic(
{
margin: 0,
height: "100%",
fontFamily: "Volvo Sans Light, Arial",
fontWeight: "normal",
"-webkit-font-smoothing": "antialiased"
},
"body"
);
Depending on what framework you use, the approach will slightly differ. Check out the examples folder to get an idea how this can is achieved.
import { getStyles, serverRendered, StyleProvider } from vcc-ui
renderToHtml: (render, Comp, meta) => {
const serverRenderer = styleRenderer();
const html = render(
<StyleProvider renderer={serverRenderer}>
<Comp />
</StyleProvider>
);
meta.styleTags = getStyles(serverRenderer).map(({ media, css }) => (
<style dangerouslySetInnerHTML={{ __html: css }} media={media} />
));
return html;
},
For languages like Arabic or Hebrew we need to be able to render components in right to left mode. This can be configured in the renderer AND ConfigContext:
import { serverRendered, StyleProvider } from vcc-ui
const renderer = styleRenderer({ isRtl: true});
<StyleProvider renderer={renderer}>
<ConfigContext.Provider value={{ isRtl: true }}>
<App />
</ConfigContext.Provider>
</StyleProvider>
You also need to set <body dir="rtl"
> in your HTML template.
A HOC that provides CSS styling context to all child components
Provide additional config for the vcc-ui components.
value (object)
Example
<StyleProvider renderer={renderer}>
<ConfigContext.Provider value={{ pathPrefix: "https://cdn.volvocars.com/" }}>
<App />
</ConfigContext.Provider>
</StyleProvider>
The rendering engine that can be customized and passed to the style provider
Get the styles that was generated for a render, useful for when sending server side generated styles to client
Will load a given list of fonts by injecting @font-face declarations
FAQs
A React library for building user interfaces at Volvo Cars
The npm package vcc-ui receives a total of 2,837 weekly downloads. As such, vcc-ui popularity was classified as popular.
We found that vcc-ui demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.