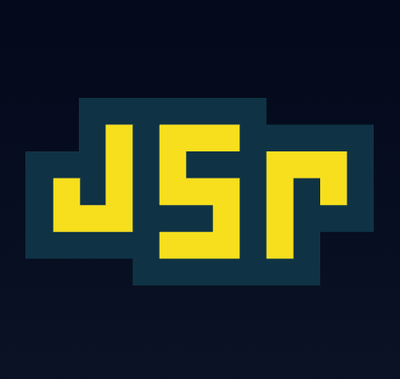
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The vega-util npm package provides utility functions for Vega, a visualization grammar. These utilities include functions for data manipulation, type checking, and other common operations needed when working with Vega visualizations.
Type Checking
Vega-util provides functions to check the type of a variable, such as isArray, isObject, and isString.
const vegaUtil = require('vega-util');
console.log(vegaUtil.isArray([1, 2, 3])); // true
console.log(vegaUtil.isObject({a: 1})); // true
console.log(vegaUtil.isString('hello')); // true
String Manipulation
Vega-util includes functions for string manipulation, such as pad and truncate.
const vegaUtil = require('vega-util');
console.log(vegaUtil.pad('test', 10)); // 'test '
console.log(vegaUtil.truncate('This is a long string', 10)); // 'This is a…'
Data Manipulation
Vega-util provides functions for data manipulation, such as array conversion and object extension.
const vegaUtil = require('vega-util');
const data = [1, 2, 3, 4, 5];
console.log(vegaUtil.array(data)); // [1, 2, 3, 4, 5]
console.log(vegaUtil.extend({}, {a: 1}, {b: 2})); // {a: 1, b: 2}
Lodash is a modern JavaScript utility library delivering modularity, performance, and extras. It provides a wide range of utility functions for common programming tasks, similar to vega-util but with a broader scope and more extensive functionality.
Underscore is a JavaScript library that provides a whole mess of useful functional programming helpers without extending any built-in objects. It offers similar utilities for data manipulation and type checking as vega-util.
Ramda is a practical functional library for JavaScript programmers. It makes it easy to create functional pipelines and provides utilities for data manipulation and type checking, similar to vega-util but with a focus on functional programming.
JavaScript utilities for Vega. Provides a set of helper methods used throughout Vega modules, including function generators, type checkers, log messages, and additional utilities for Object, Array and String values.
Functions and function generators for accessing and comparing values.
# vega.accessor(function[, fields, name]) <>
Annotates a function instance with a string array of dependent data fields and a string name, and returns the input function. Assumes the input function takes an object (data tuple) as input, and that strings in the fields array correspond to object properties accessed by the function. Once annotated, Vega dataflows can track data field dependencies and generate appropriate output names (e.g., when computing aggregations) if the function is used as an accessor.
Internally, this method assigns the field array to the fields
property of the input function, and the name to the fname
property.
To be future-proof, clients should not access these properties
directly. Instead, use the accessorFields and
accessorName methods.
# vega.accessorFields(accessor) <>
Returns the array of dependent field names for a given accessor function. Returns null if no field names have been set.
# vega.accessorName(accessor) <>
Returns the name string for a given accessor function. Returns null if no name has been set.
# vega.compare(fields[, orders]) <>
Generates a comparator function for sorting data values, based on the given
set of fields and optional sort orders. The fields argument must be
either a string or an array of strings, indicating the name of object
properties to sort by, in precedence order. Field strings may include
nested properties (e.g., foo.bar.baz
). The orders argument must be
either a string or an array of strings; the valid string values are
'ascending'
(for ascending sort order of the corresponding field) or
'descending'
(for descending sort order of the corresponding field).
If the orders argument is omitted, is shorter than the fields array,
or includes values other than 'ascending'
or 'descending'
,
corresponding fields will default to ascending order.
Given an input value, returns a function that simply returns that value. If the input value is itself a function, that function is returned directly.
# vega.field(field[, name]) <>
Generates an accessor function for retrieving the specified field value.
The input field string may include nested properties (e.g., foo.bar.baz
).
An optional name argument indicates the accessor name for the generated
function; if excluded the field string will be used as the name (see the
accessor method for more details).
var fooField = vega.field('foo');
fooField({foo: 5}); // 5
vega.accessorName(fooField); // 'foo'
vega.accessorFields(fooField); // ['foo']
var pathField = vega.field('foo.bar', 'path');
pathField({foo: {bar: 'vega'}}); // 'vega'
pathField({foo: 5}); // undefined
vega.accessorName(pathField); // 'path'
vega.accessorFields(pathField); // ['foo.bar']
An accessor function that returns the value of the id
property of an
input object.
An accessor function that simply returns its value argument.
Generates an accessor function that returns a key string (suitable for
using as an object property name) for a set of object fields. The
fields argument must be either a string or string array, with each
entry indicating a property of an input object to be used to produce
representative key values. The resulting key function is an
accessor instance with the accessor name 'key'
.
var keyf = vega.key(['foo', 'bar']);
keyf({foo:'hi', bar:5}); // 'hi|5'
vega.accessorName(keyf); // 'key'
vega.accessorFields(keyf); // ['foo', 'bar']
An accessor function that simply returns the value one (1
).
An accessor function that simply returns the value zero (0
).
An accessor function that simply returns the boolean true
value.
An accessor function that simply returns the boolean false
value.
Functions for checking the type of JavaScript values.
Returns true
if the input value is an Array instance, false
otherwise.
Returns true
if the input value is a Function instance, false
otherwise.
Returns true
if the input value is a Number instance, false
otherwise.
Returns true
if the input value is an Object instance, false
otherwise.
Returns true
if the input value is a String instance, false
otherwise.
Functions for manipulating JavaScript Object values.
# vega.extend(target[, source1, source2, …]) <>
Extends a target object by copying (in order) all enumerable properties of the input source objects.
# vega.inherits(child, parent)
A convenience method for setting up object-oriented inheritance. Assigns the
prototype
property of the input child function, such that the child
inherits the properties of the parent function's prototype via prototypal
inheritance. Returns the new child prototype object.
Functions for manipulating JavaScript Array values.
Ensures that the input value is an Array instance. If so, the value is
simply returned. If not, the value is wrapped within a new single-element
an array, returning [value]
.
# vega.extentIndex(array[, accessor]) <>
Returns the array indices for the minimum and maximum values in the input
array (as a [minIndex, maxIndex]
array), according to natural ordering.
The optional accessor argument provides a function that is first applied
to each array value prior to comparison.
vega.extentIndex([1,5,3,0,4,2]); // [3, 1]
vega.extentIndex([
{a: 3, b:2},
{a: 2, b:1},
{a: 1, b:3}
], vega.field('b')); // [1, 2]
Returns the last element in the input array. Similar to the built-in
Array.pop
method, except that it does not remove the last element. This
method is a convenient shorthand for array[array.length - 1]
.
# vega.merge(compare, array1, array2[, output]) <>
Merge two sorted arrays into a single sorted array. The input compare function is a comparator for sorting elements and should correspond to the pre-sorted orders of the array1 and array2 source arrays. The merged array contents are written to the output array, if provided. If output is not specified, a new array is generated and returned.
Given an input array of values, returns a new Object instance whose
property keys are the values in array, each assigned a property value
of 1
. Each value in array is coerced to a String value and so
should map to a reasonable string key value.
vega.toSet([1, 2, 3]); // {'1':1, '2':1, '3':1}
# vega.visitArray(array, [filter,] visitor) <>
Vists the values in an input array, invoking the visitor function
for each array value that passes an optional filter. If specified,
the filter function is called with each individual array value. If
the filter function return value is truthy, the returned value is
then passed as input to the visitor function. Thus, the filter
not only performs filtering, it can serve as a value transformer.
If the filter function is not specified, all values in the array
are passed to the visitor function. Similar to the built-in
Array.forEach
method, the visitor function is invoked with three
arguments: the value to visit, the current index into the source array,
and a reference to the soure array.
// console output: 1 0; 3 2
vega.visitArray([0, -1, 2],
function(x) { return x + 1; },
function(v, i, array) { console.log(v, i); });
Functions for generating and manipulating JavaScript String values.
# vega.pad(string, length[, character, align]) <>
Pads a string value with repeated instances of a character up to a
specified length. If character is not specified, a space (' '
) is used.
By default, padding is added to the end of a string. An optional align
parameter specifies if padding should be added to the 'left'
(beginning),
'center'
, or 'right'
(end) of the input string.
vega.pad('15', 5, '0', 'left'); // '00015'
# vega.repeat(string, count) <>
Given an input string, returns a new string that repeats the input count times.
vega.repeat('0', 5); // '00000'
# vega.splitAccessPath(path) <>
Splits an input string representing an access path for JavaScript object properties into an array of constituent path elements.
vega.splitAccessPath('foo'); // ['foo']
vega.splitAccessPath('foo.bar'); // ['foo', 'bar']
vega.splitAccessPath('foo["bar"]'); // ['foo', 'bar']
vega.splitAccessPath('foo[0].bar'); // ['foo', '0', 'bar']
Returns an output representation of an input value that is both JSON
and JavaScript compliant. For Object and String values, JSON.stringify
is
used to generate the output string. Primitive types such as Number or Boolean
are returned as-is. This method can be used to generate values that can then
be included in runtime-compiled code snippets (for example, via the Function
constructor).
# vega.truncate(string length[, align, ellipsis]) <>
Truncates an input string to a target length. The optional align argument
indicates what part of the string should be truncated: 'left'
(the beginning),
'center'
, or 'right'
(the end). By default, the 'right'
end of the string
is truncated. The optional ellipsis argument indicates the string to use to
indicate truncated content; by default the ellipsis character (…
, same as
\u2026
) is used.
Generates a new logger instance for selectively writing log messages to the JavaScript console. The optional level argument indicates the initial log level to use (one of None, Warn, Info, or Debug), and defaults to None if not specified.
The generated logger instance provides the following methods:
console.warn
method
if the current log level is Warn or higher.console.log
method if the current log level is Info or higher.console.log
method
if the current log level is Debug or higher.Constant value indicating a log level of 'None'. If set as the log level of a logger instance, all log messages will be suppressed.
Constant value indicating a log level of 'Warn'. If set as the log level of a logger instance, only warning messages will be presented.
Constant value indicating a log level of 'Info'. If set as the log level of a logger instance, both warning and info messages will be presented.
Constant value indicating a log level of 'Debug'. If set as the log level of a logger instance, all log messages (warning, info and debug) will be presented.
Throws a new error with the provided error message. This is a convenience method adding a layer of indirection for error handling, for example allowing error conditions to be included in expression chains.
vega.error('Uh oh'); // equivalent to: throw Error('Uh oh')
// embed error in an expression
return isOk ? returnValue : vega.error('Not OK');
FAQs
JavaScript utilities for Vega.
The npm package vega-util receives a total of 178,492 weekly downloads. As such, vega-util popularity was classified as popular.
We found that vega-util demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.