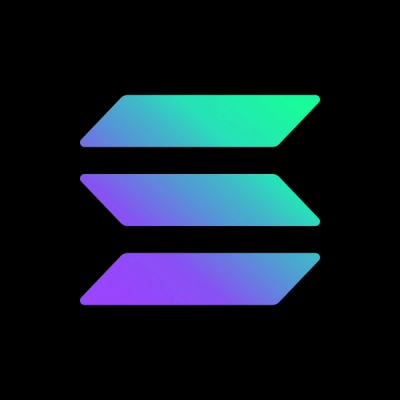
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
verto-cache-interface
Advanced tools
A communication package with Verto Cache System
Install with npm:
$ npm install verto-cache-interface
Signature:
fetchContract<T = any>(contractId: string, withValidity?: boolean, dontThrow?: boolean): Promise<StateResult<T> | undefined>
Parameters:
T: Interface of the contract state to be returned
contractId: Contract to be fetched
withValidity: Whether validity should be fetched (Default: false)
dontThrow: Whether it should not throw an error if contract is not found (Default: false)
Usage:
import { fetchContract } from "verto-cache-interface";
fetchContract("t9T7DIOGxx4VWXoCEeYYarFYeERTpWIC1V3y-BPZgKE").then((result) => {
const state = result.state;
const validity = result.validity;
});
Signature:
fetchBalanceByUserAddress = async (contractId: string, userAddress: string): Promise<UserBalance | undefined>
Parameters: contractId: Contract to be fetched userAddress: Address to obtain balance from
Usage:
import { fetchBalanceByUserAddress } from "verto-cache-interface";
fetchBalanceByUserAddress(
"bQGRi3eO4p7S583mYYXDeVn5EvGPFMiMWd5WBWatteY",
"vxUdiv2fGHMiIoek5E4l3M5qSuKCZtSaOBYjMRc94JU"
).then((result) => {
const contractId = result.contractId;
const contractName = result.name;
const contractTicker = result.ticker;
const contractLogo = result.logo;
const userBalance = result.balance;
const userAddress = result.userAddress;
const contractType = result.type;
});
Signature:
fetchBalancesForAddress = async (userAddress: string, tokenType?: string): Promise<Array<UserBalance>>
Parameters: userAddress: Address to obtain balance from tokenType: Type to filter balances from, for example: 'art'.
Usage:
import { fetchBalancesForAddress } from "verto-cache-interface";
fetchBalancesForAddress("vxUdiv2fGHMiIoek5E4l3M5qSuKCZtSaOBYjMRc94JU").then(
(result) => {
const balances: Array<UserBalance> = result;
}
);
Signature:
fetchBalancesByUsername = async (username: string, tokenType?: string): Promise<Array<UserBalance> | undefined>
Parameters: username: Username to fetch balances from. tokenType: Type to filter balances from, for example: 'art'.
Usage:
import { fetchBalancesByUsername } from "verto-cache-interface";
fetchBalancesByUsername("t8").then((result) => {
const balances: Array<UserBalance> = result;
balances.forEach((balance) => {
console.log("Contract Id ", balance.contractId);
console.log("Balance ", balance.balance);
});
});
Signature:
fetchBalancesInContract = async (contractId: string): Promise<BalanceAndContract>
Parameters: contractId: Contract to be fetched
Usage:
import { fetchBalancesInContract } from "verto-cache-interface";
fetchBalancesInContract("bQGRi3eO4p7S583mYYXDeVn5EvGPFMiMWd5WBWatteY").then(
(result) => {
const balances: BalanceAndContract = result;
const [balancesObject, contractMetadata] = balances;
const balanceForAddress =
balancesObject["vxUdiv2fGHMiIoek5E4l3M5qSuKCZtSaOBYjMRc94JU"];
const contractTicker = contractMetadata.ticker;
}
);
Signature:
fetchCollectionById = async (collectionId: string): Promise<CollectionResult | undefined>
Parameters: collectionId: Collection (contract) to be fetched
Usage:
import { fetchCollectionById } from "verto-cache-interface";
fetchCollectionById("GirFtyB_PI4oQXhEFrHZLpFUqincHrDdDxPaQ1M8r00").then(
(result) => {
const id = result.id;
const collectionName = result.name;
const description = result.description;
const owner = result.owner;
const collaborators = result.collaborators;
const items = result.items;
}
);
Signature:
fetchContractsInUser = async (addressId: string): Promise<Array<string>>
Parameters: addressId: Address to obtain related-contracts from
Usage:
import { fetchContractsInUser } from "verto-cache-interface";
fetchContractsInUser("vxUdiv2fGHMiIoek5E4l3M5qSuKCZtSaOBYjMRc94JU").then(
(result) => {
// Contract Ids
const relatedContractsToUser: Array<string> = result;
}
);
Signature:
fetchOwnershipByUsername = async (username: string): Promise<Array<string>>
Parameters: username: Username to fetch ownership from
Usage:
import { fetchOwnershipByUsername } from "verto-cache-interface";
fetchOwnershipByUsername("t8").then((result) => {
// Contract Ids
const tokensCreatedByUser: Array<string> = result;
});
Signature:
fetchTokenMetadata = async (tokenId: string, fromContract?: boolean): Promise<TokenMetadata | undefined>
Parameters: tokenId: Token (contract) id to fetch metadata from fromContract: Whether it should be fetched from the Google CDN or Verto Database (Default: False)
Usage:
import { fetchTokenMetadata } from "verto-cache-interface";
fetchTokenMetadata("bQGRi3eO4p7S583mYYXDeVn5EvGPFMiMWd5WBWatteY").then(
(result) => {
const id = result.id;
const type = result.type;
const listerUsername = result.lister;
}
);
Signature:
fetchTokenStateMetadata = async (tokenId: string): Promise<TokenStateMetadata | undefined>
Parameters: tokenId: Token (contract) id to fetch metadata from
Usage:
import { fetchTokenStateMetadata } from "verto-cache-interface";
fetchTokenStateMetadata("bQGRi3eO4p7S583mYYXDeVn5EvGPFMiMWd5WBWatteY").then(
(result) => {
const id = result.id;
const tokenName = result.name;
const owner = result.owner;
const ticker = result.ticker;
}
);
Signature:
fetchTokens = async (specificType?: string): Promise<Array<TokenMetadata>>
Parameters: specificType: Whether to filter tokens with a specific type
Usage:
import { fetchTokens } from "verto-cache-interface";
fetchTokens().then((result) => {
const allTokens: Array<TokenMetadata> = result;
allTokens.forEach((item) => {
console.log("Id ", item.id);
console.log("Type ", item.type);
console.log("Lister ", item.lister);
});
});
Signature:
fetchRandomArtwork = async (limit: number = 4): Promise<RandomArtworkResult>
Parameters: limit: Maximum number of artwork to be fetched (Default: 4)
Usage:
import { fetchRandomArtwork } from "verto-cache-interface";
fetchRandomArtwork().then((result) => {
const artwork: Array<TokenMetadata> = result.entities;
artwork.forEach((item) => {
console.log("Lister", item.lister);
console.log("Type", item.type);
console.log("Contract ID", item.contractId);
});
});
Signature:
fetchUserCreations = async (username: string): Promise<Array<string>>
Parameters: username: Username to fetch creations from
Usage:
import { fetchUserCreations } from "verto-cache-interface";
fetchUserCreations("t8").then((result) => {
// Contract Ids
const creations: Array<string> = result;
});
Signature:
fetchUserMetadataByUsername = async (username: string): Promise<UserMetadata | undefined>
Parameters: username: Username to fetch metadata from
Usage:
import { fetchUserMetadataByUsername } from "verto-cache-interface";
fetchUserMetadataByUsername("t8").then((result) => {
const username = result.username;
const addresses: Array<string> = result.addresses;
});
Signature:
fetchUsers = async (): Promise<Array<CommunityContractPeople>>
Usage:
import { fetchUsers } from "verto-cache-interface";
fetchUsers().then((result) => {
result.forEach((user) => {
console.log("Username ", user.username);
console.log("Name ", user.name);
console.log("Addresses ", user.addresses);
console.log("Image ", user.image);
console.log("Bio ", user.bio);
console.log("Links ", user.links);
});
});
Signature:
fetchUserByUsername = async (): Promise<CommunityContractPeople | undefined>
Usage:
import { fetchUserByUsername } from "verto-cache-interface";
fetchUserByUsername("t8").then((result) => {
console.log("Username ", user.username);
console.log("Name ", user.name);
console.log("Addresses ", user.addresses);
console.log("Image ", user.image);
console.log("Bio ", user.bio);
console.log("Links ", user.links);
});
Signature:
fetchRandomArtworkWithUser = async (amount?: number): Promise<Array<ArtworkOwner>>
Usage:
import { fetchRandomArtworkWithUser } from "verto-cache-interface";
fetchRandomArtworkWithUser().then((arts) => {
arts.forEach((result) => {
console.log("Artwork ID ", result.id);
console.log("Name ", result.name);
console.log("Type ", result.type);
console.log("Images ", result.images);
console.log("Owner username ", result.owner.username);
});
});
Signature:
fetchRandomCommunities = async (): Promise<TokenMetadataLookUp>
Usage:
import { fetchRandomCommunities } from "verto-cache-interface";
fetchRandomCommunities().then((result) => {
const communities = result.entities;
communities.forEach((com) => {
console.log(com.contractId);
console.log(com.type);
console.log(com.lister);
console.log(com.id);
});
});
Signature:
fetchRandomCommunitiesWithMetadata = async (): Promise<Array<RandomCommunities>>
Usage:
import { fetchRandomCommunitiesWithMetadata } from "verto-cache-interface";
fetchRandomCommunitiesWithMetadata().then((result) => {
result.forEach((com) => {
console.log(com.id);
console.log(com.name);
console.log(com.ticker);
console.log(com.logo);
console.log(com.description);
});
});
Signature:
fetchTopCommunities = async (): Promise<Array<RandomCommunities>>
Usage:
import { fetchTopCommunities } from "verto-cache-interface";
fetchTopCommunities().then((result) => {
result.forEach((com) => {
console.log(com.id);
console.log(com.name);
console.log(com.ticker);
console.log(com.logo);
console.log(com.description);
});
});
Signature:
fetchCommunitiesMetadata = async (communityContractIds: Array<string> | string): Promise<Array<RandomCommunities>>
Usage:
import { fetchCommunitiesMetadata } from "verto-cache-interface";
fetchCommunitiesMetadata(["MY-community-id1", "MY-community-id2"]).then(
(result) => {
result.forEach((com) => {
console.log(com.id);
console.log(com.name);
console.log(com.ticker);
console.log(com.logo);
console.log(com.description);
});
}
);
Signature:
fetchArtworkMetadata = async (): Promise<ArtworkMetadata | undefined>
Usage:
import { fetchArtworkMetadata } from "verto-cache-interface";
fetchArtworkMetadata().then((result) => {
console.log(result.id);
console.log(result.name);
console.log(result.lister); // Object (username, name, addresses, bio, links, image)
console.log(result.lister.addresses);
console.log(result.lister.bio);
console.log(result.lister.links);
});
Signature:
fetchTokenById = async (tokenId: string, filter?: (val: CommunityContractToken) => boolean): Promise<CommunityContractToken | undefined>
Usage:
import { fetchTokenById } from "verto-cache-interface";
fetchTokenById("ABC").then((result) => {
console.log(result.id);
console.log(result.type);
console.log(result.lister);
});
fetchTokenById("ABC", (filterData) => filterData.type === "community").then(
(result) => {
console.log(result.id);
console.log(result.type);
console.log(result.lister);
}
);
Signature:
fetchPaginated = async<T extends PaginatedToken | CommunityPeople>(type: "people" | "tokens", pageSize: number = 10, page: number = 1, sort: boolean = false): Promise<PaginatedData<T>>
Usage:
import { fetchPaginated } from "verto-cache-interface";
fetchPaginated<PaginatedToken>("tokens").then(async (result) => {
console.log(result.items); // [token1, token2, ...]
console.log(result.hasNextPage()); // true
console.log(await result.nextPage()); // CALL next page: fetchPaginated("tokens", 10, 2)
console.log(result.isEmpty()); // False
console.log(result.getPaginationInfo()); // Information about paginated results
});
Signature
fetchPriceHistory = async (pair: [string, string], desc?: boolean): Promise<Array<VwapModel>>
Usage
import { fetchPriceHistory } from "verto-cache-interface"
fetchPriceHistory(["A", "B"]).then((result) => {
result.forEach((vwap) => {
console.log(vwap.block);
console.log(vwap.vwap);
console.log(vwap.dominantToken);
});
})
Signature
fetchLatestPrice = async (pair: [string, string]): Promise<VwapModel | undefined>
Usage
import { fetchLatestPrice } from "verto-cache-interface"
fetchLatestPrice(["A", "B"]).then((result) => {
console.log(vwap.block);
console.log(vwap.vwap);
console.log(vwap.dominantToken);
})
Hooks are a way to invoke functions and then invoke certain behaviors inside the cache system.
cacheContractHook
Signature:
cacheContractHook = async (action: () => Promise<any> | any, contractId?: string | string[], refreshCommunityContract?: boolean)
Parameters:
action: Action to be called inside before executing the hook
contractId: Contract to be cached right after action
has finished its execution. If an array, it'll cache all the ids provided in the array.
refreshCommunityContract: Whether the community contract should be updated after action
has finished its execution
Usage:
import { cacheContractHook } from "verto-cache-interface";
const currentContract: string = "ABCD1234";
// const currentContracts: string[] = ['ABCD1234', '12903LLLEP'];
const executeOrder = await cacheContractHook(
async () => {
// Execute an order inside the exchange
// Or do something different, maybe buy a car.
return "ORDER_SENT";
},
currentContract,
true
);
assert(executeOrder === "ORDER_SENT");
Lifecycle:
action
(if asynchronous, it will be awaited)contractId
refreshCommunityContract
is true
, call Cache API to invoke caching of community contractimport { CacheInterfaceConstants } from "verto-cache-interface";
CacheInterfaceConstants.CACHE_API = "http://localhost";
CacheInterfaceConstants.COMMUNITY_CONTRACT = "[id]";
CacheInterfaceConstants.CONTRACT_CDN = "https://storage.googleapis.com/contracts";
render();
FAQs
A communication package with Verto Cache System
We found that verto-cache-interface demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.