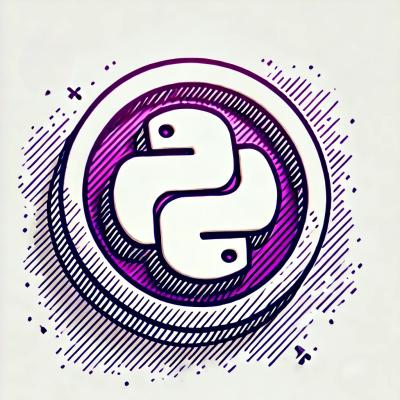
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
The vfile npm package is a virtual file format for text processing systems, which allows for the manipulation and handling of file-related information in a structured and consistent way. It is commonly used in projects involving the processing of markdown, text, and similar content, providing a standardized interface for plugins and utilities.
Creating and modifying virtual files
This feature allows users to create a new virtual file and modify its contents. The example demonstrates creating a vfile with initial content and then appending additional text to it.
const vfile = require('vfile');
const file = vfile({path: './example.txt', contents: 'Hello, world!'});
file.contents += ' Modified content.';
console.log(file.contents);
Accessing file metadata
This feature enables users to access metadata of the file such as the path and basename. The example shows how to create a vfile and retrieve its path and basename properties.
const vfile = require('vfile');
const file = vfile({path: './example.txt', contents: 'Hello, world!'});
console.log(file.path); // Outputs: './example.txt'
console.log(file.basename); // Outputs: 'example.txt'
Handling errors and messages
This feature is useful for adding error or warning messages to a file, which can be particularly helpful in linting tools or compilers. The example demonstrates adding an error message to a vfile and logging all associated messages.
const vfile = require('vfile');
const file = vfile();
file.message('Unknown error', {line: 1, column: 1});
console.log(file.messages);
Vinyl is a virtual file format that is often used in the gulp build system. It is similar to vfile in that it represents file objects in a virtual form, but it is more focused on being used with streams, particularly in the context of gulp's pipelines.
mem-fs provides an in-memory file system which stores file representations similar to vfile. While vfile is designed for text processing with a focus on linting and transformation, mem-fs is geared more towards temporary storage and manipulation of files in memory during tasks like scaffolding or testing.
vfile is a small and browser friendly virtual file format that tracks
metadata (such as a file’s path
and value
) and messages.
It was made specifically for unified and generally for the common task
of parsing, transforming, and serializing data, where vfile
handles everything
about the document being compiled.
This is useful for example when building linters, compilers, static site
generators, or other build tools.
vfile is part of the unified collective.
unifiedjs.com
vfile is different from the excellent
vinyl
in that it has a smaller API, a smaller size, and focuses on messages.
This package is ESM only:
Node 12+ is needed to use it and it must be import
ed instead of require
d.
npm:
npm install vfile
import {VFile} from 'vfile'
var file = new VFile({path: '~/example.txt', value: 'Alpha *braavo* charlie.'})
file.path // => '~/example.txt'
file.dirname // => '~'
file.extname = '.md'
file.basename // => 'example.md'
file.basename = 'index.text'
file.history // => ['~/example.txt', '~/example.md', '~/index.text']
file.message('`braavo` is misspelt; did you mean `bravo`?', {line: 1, column: 8})
console.log(file.messages)
Yields:
[
[~/index.text:1:8: `braavo` is misspelt; did you mean `bravo`?] {
reason: '`braavo` is misspelt; did you mean `bravo`?',
line: 1,
column: 8,
source: null,
ruleId: null,
position: {start: [Object], end: [Object]},
file: '~/index.text',
fatal: false
}
]
This package exports the following identifiers: VFile
.
There is no default export.
VFile(options?)
Create a new virtual file.
If options
is string
or Buffer
, treats it as {value: options}
.
If options
is a VFile
, shallow copies its data over to the new file.
All other given fields are set on the newly created VFile
.
Path related properties are set in the following order (least specific to most
specific): history
, path
, basename
, stem
, extname
, dirname
.
It’s not possible to set either dirname
or extname
without setting either
history
, path
, basename
, or stem
as well.
new VFile()
new VFile('console.log("alpha");')
new VFile(Buffer.from('exit 1'))
new VFile({path: path.join('path', 'to', 'readme.md')})
new VFile({stem: 'readme', extname: '.md', dirname: path.join('path', 'to')})
new VFile({other: 'properties', are: 'copied', ov: {e: 'r'}})
vfile.value
Buffer
, string
, null
— Raw value.
vfile.cwd
string
— Base of path
.
Defaults to process.cwd()
.
vfile.path
string?
— Path of vfile
.
Cannot be nullified.
You can set a file URL (a URL
object with a file:
protocol)
which will be turned into a path with url.fileURLToPath
.
vfile.basename
string?
— Current name (including extension) of vfile
.
Cannot contain path separators.
Cannot be nullified either (use file.path = file.dirname
instead).
vfile.stem
string?
— Name (without extension) of vfile
.
Cannot be nullified, and cannot contain path separators.
vfile.extname
string?
— Extension (with dot) of vfile
.
Cannot be set if there’s no path
yet and cannot contain path separators.
vfile.dirname
string?
— Path to parent directory of vfile
.
Cannot be set if there’s no path
yet.
vfile.history
Array.<string>
— List of file-paths the file moved between.
vfile.messages
Array.<VMessage>
— List of messages associated with the file.
vfile.data
Object
— Place to store custom information.
It’s OK to store custom data directly on the vfile
, moving it to data
gives
a little more privacy.
VFile#toString(encoding?)
Convert value of vfile
to string.
When value
is a Buffer
, encoding
is a
character encoding to understand it as (string
, default:
'utf8'
).
VFile#message(reason[, position][, origin])
Associates a message with the file, where fatal
is set to false
.
Constructs a new VMessage
and adds it to
vfile.messages
.
VFile#info(reason[, position][, origin])
Associates an informational message with the file, where fatal
is set to
null
.
Calls #message()
internally.
VFile#fail(reason[, position][, origin])
Associates a fatal message with the file, then immediately throws it.
Note: fatal errors mean a file is no longer processable.
Calls #message()
internally.
The following list of projects includes tools for working with virtual files. See unist for projects that work with nodes.
convert-vinyl-to-vfile
— transform from Vinyl to vfileto-vfile
— create a vfile from a filepathvfile-find-down
— find files by searching the file system downwardsvfile-find-up
— find files by searching the file system upwardsvfile-glob
— find files by glob patternsvfile-is
— check if a vfile passes a testvfile-location
— convert between positional and offset locationsvfile-matter
— parse the YAML front mattervfile-message
— create a vfile messagevfile-messages-to-vscode-diagnostics
— transform vfile messages to VS Code diagnosticsvfile-mkdirp
— make sure the directory of a vfile exists on the file systemvfile-rename
— rename the path parts of a vfilevfile-sort
— sort messages by line/columnvfile-statistics
— count messages per category: failures, warnings, etcvfile-to-eslint
— convert to ESLint formatter compatible outputThe following list of projects show linting results for given virtual files.
Reporters must accept Array.<VFile>
as their first argument, and return
string
.
Reporters may accept other values too, in which case it’s suggested to stick
to vfile-reporter
s interface.
vfile-reporter
— create a reportvfile-reporter-json
— create a JSON reportvfile-reporter-folder-json
— create a JSON representation of vfilesvfile-reporter-pretty
— create a pretty reportvfile-reporter-junit
— create a jUnit reportvfile-reporter-position
— create a report with content excerptsSee contributing.md
in vfile/.github
for ways to
get started.
See support.md
for ways to get help.
Ideas for new utilities and tools can be posted in vfile/ideas
.
This project has a code of conduct. By interacting with this repository, organization, or community you agree to abide by its terms.
Support this effort and give back by sponsoring on OpenCollective!
Gatsby 🥇 |
Vercel 🥇 |
Netlify![]() |
Holloway |
ThemeIsle |
Boost Hub![]() |
Expo |
You? |
The initial release of this project was authored by @wooorm.
Thanks to @contra, @phated, and others for their work on Vinyl, which was a huge inspiration.
Thanks to @brendo, @shinnn, @KyleAMathews, @sindresorhus, and @denysdovhan for contributing commits since!
FAQs
Virtual file format for text processing
We found that vfile demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.