vite-plugin-electron
Electron 🔗 Vite
English
|
简体中文
Features
- 🚀 Fully compatible with Vite and Vite's ecosystem (Based on Vite)
- 🎭 Flexible configuration
- 🐣 Few APIs, easy to use
- 🔥 Hot restart
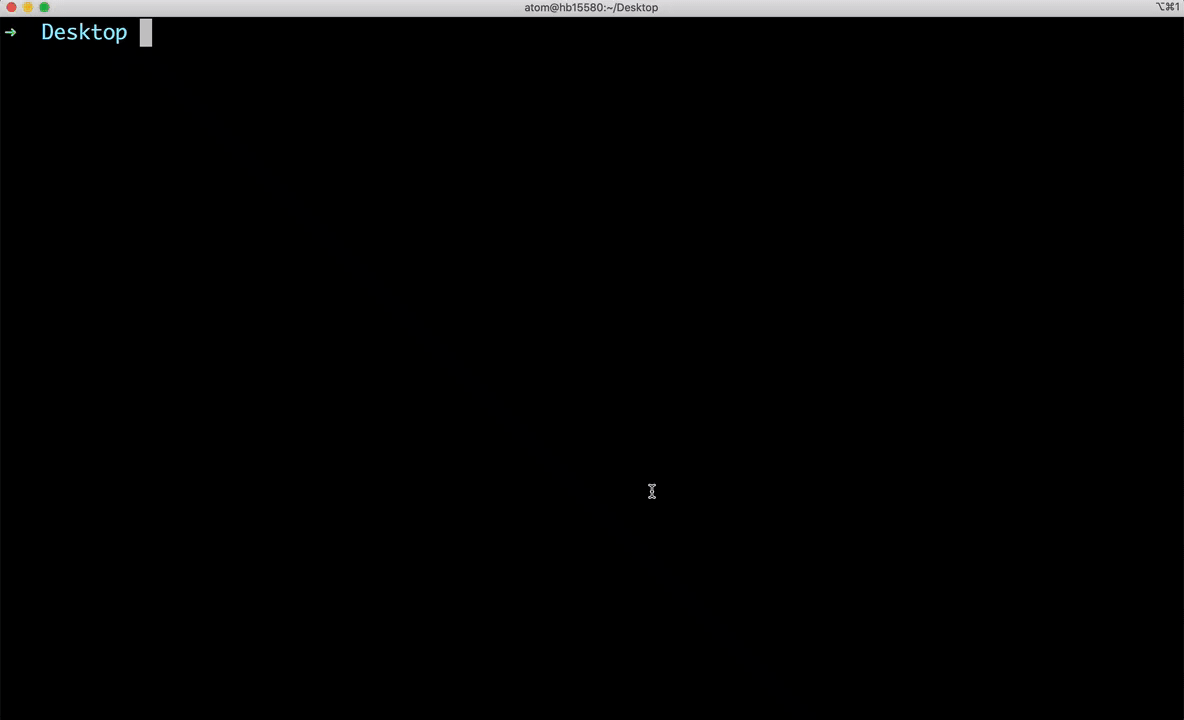
Quick Setup
- Add the following dependency to your project
npm i -D vite-plugin-electron
- Add
vite-plugin-electron
to the plugins
section of vite.config.ts
import electron from 'vite-plugin-electron'
export default {
plugins: [
electron({
entry: 'electron/main.ts',
}),
],
}
- Create the
electron/main.ts
file and type the following code
import { app, BrowserWindow } from 'electron'
app.whenReady().then(() => {
const win = new BrowserWindow({
title: 'Main window',
})
if (process.env.VITE_DEV_SERVER_URL) {
win.loadURL(process.env.VITE_DEV_SERVER_URL)
} else {
win.loadFile('dist/index.html');
}
})
- Add the
main
entry to package.json
{
+ "main": "dist-electron/main.js"
}
That's it! You can now use Electron in your Vite app ✨
API (Define)
electron(config: Configuration | Configuration[])
export interface Configuration {
entry?: import('vite').LibraryOptions['entry']
vite?: import('vite').InlineConfig
onstart?: (args: {
/**
* Electron App startup function.
* It will mount the Electron App child-process to `process.electronApp`.
* @param argv default value `['.', '--no-sandbox']`
*/
startup: (argv?: string[]) => Promise<void>
/** Reload Electron-Renderer */
reload: () => void
}) => void | Promise<void>
}
Recommend Structure
Let's use the official template-vanilla-ts created based on create vite
as an example
+ ├─┬ electron
+ │ └── main.ts
├─┬ src
│ ├── main.ts
│ ├── style.css
│ └── vite-env.d.ts
├── .gitignore
├── favicon.svg
├── index.html
├── package.json
├── tsconfig.json
+ └── vite.config.ts
JavaScript API
vite-plugin-electron
's JavaScript APIs are fully typed, and it's recommended to use TypeScript or enable JS type checking in VS Code to leverage the intellisense and validation.
Configuration
- typedefineConfig
- functionresolveViteConfig
- function, Resolve the default Vite's InlineConfig
for build Electron-MainwithExternalBuiltins
- functionbuild
- functionstartup
- function
Example
import { build, startup } from 'vite-plugin-electron'
const isDev = process.env.NODE_ENV === 'development'
const isProd = process.env.NODE_ENV === 'production'
build({
entry: 'electron/main.ts',
vite: {
mode: process.env.NODE_ENV,
build: {
minify: isProd,
watch: isDev ? {} : null,
},
plugins: [{
name: 'plugin-start-electron',
closeBundle() {
if (isDev) {
startup()
}
},
}],
},
})
How to work
It just executes the electron .
command in the Vite build completion hook and then starts or restarts the Electron App.
Be aware
- 🚨 By default, the files in
electron
folder will be built into the dist-electron
- 🚨 Currently,
"type": "module"
is not supported in Electron - 🚨 In general, Vite may not correctly build Node.js packages, especially C/C++ native modules, but Vite can load them as external packages. So, put your Node.js package in
dependencies
. Unless you know how to properly build them with Vite.
electron({
entry: 'electron/main.ts',
vite: {
build: {
rollupOptions: {
external: [
'serialport',
'sqlite3',
],
},
},
},
}),