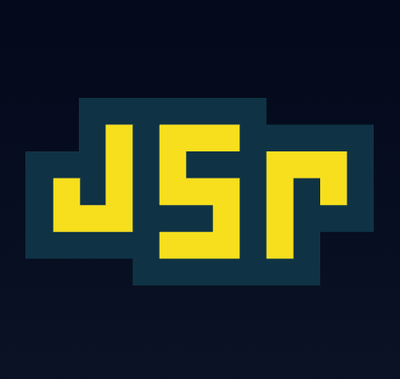
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Promises/A+ implementation. See https://github.com/promises-aplus/promises-spec.
###In the Node.js### You can install using Node Package Manager (npm):
npm install vow
###In the Browsers###
<script type="text/javascript" src="vow.min.js"></script>
Also RequireJS module format supported.
####Vow.promise([value])####
Create a new promise if no value
given, or create a new fulfilled promise if the value
is not a promise, or returns value
if the given value
is a promise.
var promise = Vow.promise(), // create a new promise
fulfilledPromise = Vow.promise('ok'), // create a new fulfilled promise
anotherPromise = Vow.promise(existingPromise); // anotherPromise is equal an existingPromise
###Promise API###
####fulfill(value)####
Fulfill promise with given value
var promise = Vow.promise();
promise.fulfill('completed'); // fulfill promise with 'completed' value
####reject(reason)####
Reject promise with given reason
var promise = Vow.promise();
promise.reject(Error('internal error')); // reject promise with Error object
####isFulfilled()#### Returns whether the promise is fulfilled
var promise = Vow.promise();
promise.isFulfilled(); // returns false
promise.fulfill('completed');
promise.isFulfilled(); // returns true
####isRejected()#### Returns whether the promise is rejected
var promise = Vow.promise();
promise.isRejected(); // returns false
promise.reject(Error('internal error'));
promise.isRejected(); // returns true
####isResolved()#### Returns whether the promise is fulfilled or rejected
var promise = Vow.promise();
promise.isResolved(); // returns false
promise.fulfill('completed'); // or promise.reject(Error('internal error'));
promise.isResolved(); // returns true
####valueOf()#### Returns value of the promise:
####then([onFulfilled], [onRejected])#### Arranges for:
onFulfilled
to be called with the value after promise is fulfilled,onRejected
to be called with the rejection reason after promise is rejected.Returns a new promise. See Promises/A+ specification for details.
var promise = Vow.promise();
promise.then(
function() { }, // to be called after promise is fulfilled
function() { }); // to be called after promise is rejected
####fail(onRejected)####
Arranges to call onRejected
on the promise's rejection reason if it is rejected. Shortcut for then(null, onRejected)
.
var promise = Vow.promise();
promise.fail(
function() { // to be called after promise is rejected
});
promise.reject(Error('error'));
####always(onResolved)####
Arranges to call onResolved
on either the promise's value if it is fulfilled, or on it's rejection reason if it is rejected. Shortcut for then(onResolved, onResolved)
.
var promise = Vow.promise();
promise.always(
function() { // to be called after promise is fulfilled or rejected
});
promise.fulfill('ok'); // or promise.reject(Error('error'));
####spread([onFulfilled], [onRejected])#### Like "then", but "spreads" the array into a variadic value handler. It useful with Vow.all, Vow.allResolved methods.
var promise1 = Vow.promise(),
promise2 = Vow.promise();
Vow.all([promise1, promise2]).spread(function(arg1, arg2) {
// arg1 should be "1", arg2 should be "'two'"
});
promise1.fulfill(1);
promise2.fulfill('two');
####done()#### Terminate a chain of promises. If the promise is rejected, throws it as an exception in a future turn of the event loop.
var promise = Vow.promise();
promise.reject(Error('Internal error'));
promise.done(); // exception to be throwed
####timeout(timeout)####
Returns a new promise that to be rejected after a timeout
if promise does not resolved beforehand.
var promise = Vow.promise(),
promiseWithTimeout1 = promise.timeout(50),
promiseWithTimeout2 = promise.timeout(200);
setTimeout(
function() {
promise.fulfill('ok');
},
100);
promiseWithTimeout1.fail(function(e) {
// promiseWithTimeout to be rejected after 50ms
});
promiseWithTimeout2.then(function(val) {
// promiseWithTimeout to be fulfilled with "'ok'" value
});
###Vow API###
####isPromise(value)####
Returns whether the given value
is a promise.
Vow.isPromise('value'); // returns false
Vow.isPromise(Vow.promise()); // returns true
####when(value, [onFulfilled], [onRejected])####
Static equivalent for promise.then. If given value
is not a promise, value
is equivalent to fulfilled promise.
####fail(value, onRejected)####
Static equivalent for promise.fail. If given value
is not a promise, value
is equivalent to fulfilled promise.
####always(value, onResolved)####
Static equivalent for promise.always. If given value
is not a promise, value
is equivalent to fulfilled promise.
####spread(value, [onFulfilled], [onRejected])####
Static equivalent for promise.spread.
If given value
is not a promise, value
is equivalent to fulfilled promise.
####done(value)####
Static equivalent for promise.done.
If given value
is not a promise, value
is equivalent to fulfilled promise.
####isFulfilled(value)####
Static equivalent for promise.isFulfilled.
If given value
is not a promise, value
is equivalent to fulfilled promise.
####isRejected(value)####
Static equivalent for promise.isRejected.
If given value
is not a promise, value
is equivalent to fulfilled promise.
####isResolved(value)####
Static equivalent for promise.isResolved.
If given value
is not a promise, value
is equivalent to fulfilled promise.
####fulfill(value)####
Returns a promise that has already been fulfilled with the given value
. If value
is a promise, returned promise will be fulfilled with fulfill/rejection value of given promise.
####reject(reason)####
Returns a promise that has already been rejected with the given reason
. If reason
is a promise, returned promise will be rejected with fulfill/rejection value of given promise.
####resolve(value)####
Returns a promise that has already been fulfilled with the given value
. If value
is a promise, returns promise
.
####all(promisesOrValues)####
Returns a promise to be fulfilled only after all items in promisesOrValues
is fulfilled, or to be rejected when the first promise is rejected.
####allResolved(promisesOrValues)####
Returns a promise to be fulfilled only after all items in promisesOrValues
is resolved.
var promise1 = Vow.promise(),
promise2 = Vow.promise();
Vow.allResolved([promise1, promise2])
.spread(function(promise1, promise2) {
promise1.valueOf(); // returns 'error'
promise2.valueOf(); // returns 'ok'
});
promise1.reject('error');
promise2.fulfill('ok');
####any(promisesOrValues)####
Returns a promise to be fulfilled only any item in promisesOrValues
is fulfilled, or to be rejected when all items is rejected (with reason of first rejected item).
####timeout(promise, timeout)####
Static equivalent for promise.timeout. If given value
is not a promise, value
is equivalent to fulfilled promise.
FAQs
DOM Promise and Promises/A+ implementation for Node.js and browsers
The npm package vow receives a total of 64,024 weekly downloads. As such, vow popularity was classified as popular.
We found that vow demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.