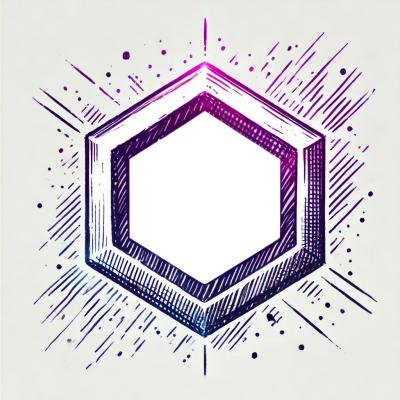
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
vtex-commons
Advanced tools
Handy VTEX library for NodeJs
This module wrapps the most common used VTEX APIs requests. Currently containing:
uuid
npm i vtex-commons
Here's a example of how to use the Master Data's Search
const VTEX = require('vtex-commons')
mondule.exports = async () => {
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.MasterData().Search({
entity : 'CL',
rule : 'email=imagooddeveloper@maybe.com',
fields : '_all'
})
}
You might be wondering: "Where are the key and token required to perform a private request? I can't see them in the code!"
You're right, they aren't in the code. And if you keep your credentials within your code, you're going to Developers Hell.
When you instanciate the module, it assumes you have at least two enviroment variables already set:
Therefore, there's no need to write them down in your code. If you need to use anothers name for this variables other then the provided, you'll be able to overwrite the default ones. More on that in a minute.
This method requires an object with four parameters:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.MasterData().Search({
entity : 'CL',
rule : 'email=imagooddeveloper@maybe.com',
fields : '_all'
})
This method requires an object with two required parameters:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.MasterData().Post({
entity : 'CL',
data : {name : 'Jane'}
})
This method requires an object with two parameters:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.MasterData().Delete({
entity : 'CL',
id : '54353fsdf-42342rfsfsfs-23rfdsfsdfds'
})
This method requires an object with three parameters:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.MasterData().Patch({
entity : 'CL',
data : {name : 'Jane'}
id : '54353fsdf-42342rfsfsfs-23rfdsfsdfds'
})
This method requires an object with one parameter:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.OMS().LoadOrder({
orderId : '54353fsdf-01'
})
This method requires an object with one parameter:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.OMS().ListOrders({
filter : 'orderBy=creationDate,desc&page=1&q=jemail=imagooddeveloper@maybe.com&f_creationDate=creationDate:%5B2021-11-17T03:00:00.000Z%20TO%202021-11-18T02:59:59.999Z%5D'
})
This method requires an object with one parameter:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.OMS().CancelOrder({
orderId : '54353fsdf-01'
})
This method requires an object with one parameter:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.Catalog().GetSKU({
skuId : 818
})
This method requires an object with one parameter:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.Catalog().GetProduct({
productId : 213
})
This method requires an object with one parameter:
vtex = new VTEX({
store : 'dummystore'
})
const searchResult = await vtex.Catalog().GetProductByRefId({
refId : 213
})
This method requires an object with one parameter:
vtex = new VTEX({
store : 'dummystore'
})
const orderForm = await vtex.Checkout().LoadOrderformById({
orderformId : 'hgcfgtr456yhef'
})
This method requires an object with the following parameters:
It returns the giftcard's redemption code.
vtex = new VTEX({
store : 'dummystore'
})
const giftcard = await vtex.Giftcard().CreateGiftcard({
value : 1
})
This method requires a object with the following parameter:
vtex = new VTEX({
store : 'dummystore'
})
const reservation = await vtex.Logistics().ListReservationById({
id : '00-1234567890-01'
})
If you want add filters to the request or list a specific subscription, you'll have to pass the 'rule' parameter. If the rule parameters is not passed, the method will return the first page of subscriptions (limited by 15 lines by default).
vtex = new VTEX({
store : 'dummystore'
})
const subs = await Subscriptions().List({
rule : 'customerEmail=test%40test.com&page=1&size=15'
})
This module is intended for any sort of tools (third party or not) that might be hepful when using vtex-tools.
This a very simple implementation of the well known npm module https://www.npmjs.com/package/uuid Good for using as unique ids in master data's entities, cache and session identifying.
vtex = new VTEX({
store : 'dummystore'
})
//default V4
vtex.Utils().uuid()
//'1b9d6bcd-bbfd-4b2d-9b5d-ab8dfbbd4bed'
//using V1
vtex.Utils().uuid(1)
//'2c5ea4c0-4067-11e9-8bad-9b1deb4d3b7d'
These are the default values when you instanciate the module:
{
appKey : "APP_KEY",
appToken : "APP_TOKEN",
store : null, /*required*/
env : "vtexcommercestable.com.br"
}
You can easily overwrite them:
vtex = new VTEX({
store : 'dummystore',
appKey : 'STORE_KEY',
appToken : 'STORE_TOKEN',
})
REMEMBER: appKey AND appToken SHOULD NOT BE FILLED WITH YOUR CREDENTIALS, BUT WITH THE NAMES OF THE ENVIROMENT VARIABLES THAT CONTAIN THEM.
If you really need to provide the credentials directly to the contructor, say from a query result, you can do this using the following snippet:
vtex = new VTEX({
store : 'dummystore',
appKey : 'vtexappkey-dummiestore-ABCDEF', //the real key
appToken : 'REWREWRW5435435REWREW432RWFSGFDYTR543TGEGFDFDSREWr34TREGFDGDGDWSADWEFDSDFDSAFSADEFGEFD', //the real token
credentials: true
})
Aways wrapp the request inside a try catch. The module will throw an error that will break the execution if something goes wrong with the request.
Fell free to improve this module. Pull requests will be welcome. Just try to keep the existing structure.
Thanks
FAQs
Handy VTEX library for NodeJs
The npm package vtex-commons receives a total of 0 weekly downloads. As such, vtex-commons popularity was classified as not popular.
We found that vtex-commons demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.