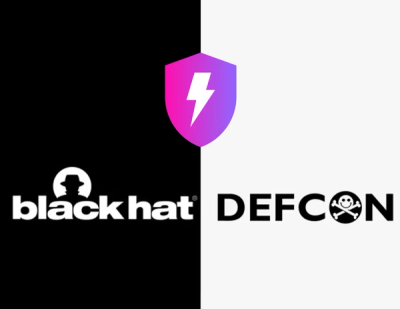
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
vue-composable
Advanced tools
Out-of-the-box ready to use composables
This library aim is to be one stop shop for many real-world composable functions, with aggressive tree-shaking to keep it light on your end code.
# @vue/composition-api
# install with yarn
yarn add @vue/composition-api vue-composable
# install with npm
npm install @vue/composition-api vue-composable
# vue 3
# install with yarn
yarn add vue-composable
# install with npm
npm install vue-composable
Check our documentation
mousemove
listener to a DOM elementresize
listener to a DOM elementscroll
listener to a DOM elementDate.now()
with custom refresh rateperformance.now()
with custom refresh rateMatchMedia
breakpoints
based on window.innerWidth
ref
ref
[vue3 only]
setInterval
on unmountlock-scroll
componentref
Storage API
, useLocalStorage
and useSessionStorage
use thislocalStorage
or on safari private it uses sessionStorage
localStorage
sessionStorage
Promise
reactive resolve and rejectlazy:true
promise
document.title
undo
and redo
ref
sfetch
wrapperWebSocket
wrapperIntersectionObserver
NetworkInformation
wrappernavigator.onLine
wrapperPage Visibility API
NavigatorLanguage
BroadcastChannel API
CSS variables
Web Worker API
New packages needed
axios
wrapper clientaxios
instancejs-cookie
wrapperThis is a monorepo project, please check packages
There's some experimental devtools support starting from 1.0.0-beta.6
, only available for vue-next
and devtools beta 6
.
To use devtools you need to install the plugin first:
import { createApp } from "vue";
import { VueComposableDevtools } from "vue-composable";
import App from "./App.vue";
const app = createApp(App);
app.use(VueComposableDevtools);
// or
app.use(VueComposableDevtools, {
id: "vue-composable",
label: "devtool composables"
});
app.mount("#app");
To add properties to the component inspector tab ComponentState
const bar = "bar";
useDevtoolsComponentState(
{
bar
},
{
type: "custom composable" // change group
}
);
const baz = () => "baz";
useDevtoolsComponentState({ baz });
// no duplicates added by default
useDevtoolsComponentState({ baz });
const the = 42;
useDevtoolsComponentState({ the });
// to allow multiple same key
useDevtoolsComponentState({ the }, { duplicate: true });
// use a devtools api list directly
interface StateBase {
key: string;
value: any;
editable: boolean;
objectType?: "ref" | "reactive" | "computed" | "other";
raw?: string;
type?: string;
}
useDevtoolsComponentState([
{
key: "_bar",
type: "direct",
value: "bar",
editable: true
},
{
key: "_baz",
type: "direct",
value: "baz",
editable: false
}
]);
// raw change
useDevtoolsComponentState((payload, ctx) => {
payload.state.push(
...[
{
key: "_bar",
type: "raw",
value: "bar",
editable: true
},
{
key: "_baz",
type: "raw",
value: "baz",
editable: false
}
]
);
});
To add timeline events:
const id = "vue-composable";
const label = "Test events";
const color = 0x92a2bf;
const { addEvent, pushEvent } = useDevtoolsTimelineLayer(
id,
description,
color
);
// adds event to a specific point in the timeline
addEvent({
time: Date.now(),
data: {
// data object
},
meta: {
// meta object
}
});
// adds event with `time: Date.now()`
pushEvent({
data: {
// data object
},
meta: {
// meta object
}
});
Allows to create a new inspector for your data.
I'm still experimenting on how to expose this API on a composable, this will likely to change in the future, suggestions are welcome.
useDevtoolsInspector(
{
id: "vue-composable",
label: "test vue-composable"
},
// list of nodes, this can be reactive
[
{
id: "test",
label: "test - vue-composable",
depth: 0,
state: {
composable: [
{
editable: false,
key: "count",
objectType: "Ref",
type: "setup",
value: myRefValue
}
]
}
}
]
);
Typescript@3.x
is not supported, the supported version can be checked on package.json, usually is the latest version or the same major as vue-3
You can contribute raising issues and by helping out with code.
Tests and Documentation are the most important things for me, because good documentation is really useful!
I really appreciate some tweaks or changes on how the documentation is displayed and how to make it easier to read.
Twitter: @pikax_dev
# install packages
yarn
# build and test for v2
yarn build --version=2
yarn test:vue2
# build and test for v3
yarn build
yarn test
git checkout -b feat/new-composable
git commit -am 'feat(composable): add a new composable'
git push origin feat/new-composable
FAQs
vue-composable
The npm package vue-composable receives a total of 2,641 weekly downloads. As such, vue-composable popularity was classified as popular.
We found that vue-composable demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.