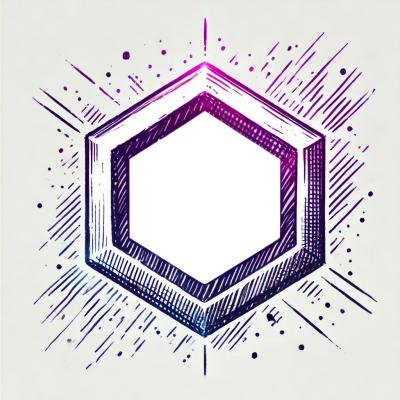
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
vue-easy-google-autocomplete
Advanced tools
A simple and easy-to-use Vue 3 component for Google Places Autocomplete, with customizable hooks and debouncing.
A comprehensive Vue 3 library for integrating Google Places Autocomplete with options for both component-based and composition API (hook) usage. This tool allows users to efficiently search and select addresses, offering features like debouncing, language support, and customizable predictions.
Install the package with npm:
npm install vue-easy-google-autocomplete
Or using yarn:
yarn add vue-easy-google-autocomplete
To use the Google Places API, you need an API key from Google Cloud. Refer to this guide for obtaining your API key.
Here’s a basic example showcasing how to implement the GooglePlacesAutocomplete
component in a Vue 3 project:
<template>
<div>
<GooglePlacesAutocomplete
:apiKey="apiKey"
placeholder="Enter an address..."
language="en"
@place-selected="handlePlaceSelected"
/>
</div>
</template>
<script>
import { defineComponent } from 'vue';
import { GooglePlacesAutocomplete } from 'vue-easy-google-autocomplete';
export default defineComponent({
components: {
GooglePlacesAutocomplete,
},
data() {
return {
apiKey: 'YOUR_GOOGLE_API_KEY',
};
},
methods: {
handlePlaceSelected(placeDetails) {
console.log('Selected place details:', placeDetails);
},
},
});
</script>
Alternatively, use the hook useGooglePlacesAutocomplete
for more granular control:
<template>
<div class="autocomplete-box">
<input
type="text"
v-model="query"
@input="fetchPredictions(query)"
:placeholder="placeholder"
/>
<ul v-if="predictions.length" class="suggestions-list">
<li
v-for="prediction in predictions"
:key="prediction.place_id"
@click="selectPlace(prediction)"
class="suggestion-item"
>
{{ prediction.description }}
</li>
</ul>
</div>
</template>
<script>
import { ref, defineComponent } from 'vue';
import { useGooglePlacesAutocomplete } from 'vue-easy-google-autocomplete';
export default defineComponent({
setup() {
const apiKey = 'YOUR_GOOGLE_API_KEY';
const {
predictions,
fetchPredictions,
fetchPlaceDetails,
resetPredictions,
} = useGooglePlacesAutocomplete(apiKey, {
debounceTime: 500,
predictionOptions: {
types: ['address'],
componentRestrictions: { country: 'br' },
},
language: 'en',
});
const query = ref('');
const selectPlace = async (prediction) => {
query.value = prediction.description;
const placeDetails = await fetchPlaceDetails(prediction.place_id);
console.log('Selected place details:', placeDetails);
resetPredictions();
};
return { query, predictions, fetchPredictions, selectPlace };
},
});
</script>
apiKey
(required): Your Google Places API key.placeholder
(optional, default: "Enter an address..."
): Placeholder text for the input field.language
(optional, default: "en"
): Language code for Google Places API localization (e.g., "pt-BR"
for Brazilian Portuguese).predictionOptions
(optional): Additional options to refine predictions, such as types or country restrictions.debounceTime
: Milliseconds to debounce input queries.predictionOptions
: Options for the Google Places autocomplete query.libraries
: Libraries to load alongside the Google Maps API, default is ['places']
.language
: Language for the API, default is 'en'
.place-selected
: Triggered upon selecting an address, providing detailed place information.Customizing the autocomplete service:
<template>
<GooglePlacesAutocomplete
:apiKey="apiKey"
language="pt-BR"
:predictionOptions="{
types: ['address'],
componentRestrictions: { country: 'br' },
}"
@place-selected="handlePlaceSelected"
/>
</template>
Input is debounced by default at 300ms, adjustable with debounceTime
:
<template>
<GooglePlacesAutocomplete
:apiKey="apiKey"
:debounceTime="500" // Custom debounce time
@place-selected="handlePlaceSelected"
/>
</template>
To enhance security, restrict your API key usage to specific referrer URLs within the Google Cloud Console settings.
Contributions to the JSnapCam are welcome! If you find any issues or have suggestions for improvements, feel free to open an issue or submit a pull request.
Thanks goes to these wonderful people (emoji key):
Guilherme L. Faustino 💻 📖 ⚠️ |
To contribute or modify the library, follow these steps for local setup:
Clone the repository:
git clone https://github.com/iguilhermeluis/vue-easy-google-autocomplete.git
Install dependencies:
npm install
Run the development server:
npm run dev
This project is licensed under the MIT License, promoting open-source use and contribution.
FAQs
A simple and easy-to-use Vue 3 component for Google Places Autocomplete, with customizable hooks and debouncing.
The npm package vue-easy-google-autocomplete receives a total of 6 weekly downloads. As such, vue-easy-google-autocomplete popularity was classified as not popular.
We found that vue-easy-google-autocomplete demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.