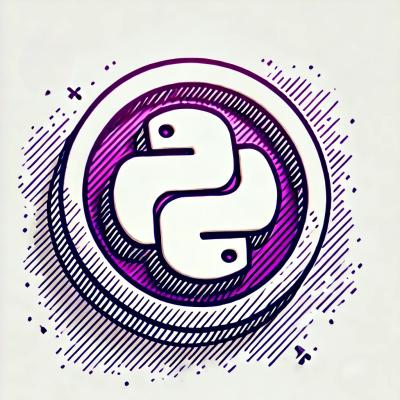
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
vue-echarts
Advanced tools
Vue.js (v2/v3) component for Apache ECharts™ (v5).
[!IMPORTANT] We have released an import code generator that can generate precise import code by pasting the
option
code.
If you are migrating from vue-echarts
≤ 5, you should read the Migration to v6 section before you update to v6.
Not ready yet? Read documentation for older versions here →
npm i echarts vue-echarts
To make vue-echarts
work for Vue 2 (<2.7.0), you need to have @vue/composition-api
installed (@vue/runtime-core
for TypeScript support):
npm i @vue/composition-api
npm i @vue/runtime-core # for TypeScript support
If you are using NuxtJS on top of Vue 2, you'll need @nuxtjs/composition-api
:
npm i @nuxtjs/composition-api
And then add '@nuxtjs/composition-api/module'
in the buildModules
option in your nuxt.config.js
.
<template>
<v-chart class="chart" :option="option" />
</template>
<script setup>
import { use } from "echarts/core";
import { CanvasRenderer } from "echarts/renderers";
import { PieChart } from "echarts/charts";
import {
TitleComponent,
TooltipComponent,
LegendComponent
} from "echarts/components";
import VChart, { THEME_KEY } from "vue-echarts";
import { ref, provide } from "vue";
use([
CanvasRenderer,
PieChart,
TitleComponent,
TooltipComponent,
LegendComponent
]);
provide(THEME_KEY, "dark");
const option = ref({
title: {
text: "Traffic Sources",
left: "center"
},
tooltip: {
trigger: "item",
formatter: "{a} <br/>{b} : {c} ({d}%)"
},
legend: {
orient: "vertical",
left: "left",
data: ["Direct", "Email", "Ad Networks", "Video Ads", "Search Engines"]
},
series: [
{
name: "Traffic Sources",
type: "pie",
radius: "55%",
center: ["50%", "60%"],
data: [
{ value: 335, name: "Direct" },
{ value: 310, name: "Email" },
{ value: 234, name: "Ad Networks" },
{ value: 135, name: "Video Ads" },
{ value: 1548, name: "Search Engines" }
],
emphasis: {
itemStyle: {
shadowBlur: 10,
shadowOffsetX: 0,
shadowColor: "rgba(0, 0, 0, 0.5)"
}
}
}
]
});
</script>
<style scoped>
.chart {
height: 400px;
}
</style>
<template>
<v-chart class="chart" :option="option" />
</template>
<script>
import { use } from "echarts/core";
import { CanvasRenderer } from "echarts/renderers";
import { PieChart } from "echarts/charts";
import {
TitleComponent,
TooltipComponent,
LegendComponent
} from "echarts/components";
import VChart, { THEME_KEY } from "vue-echarts";
use([
CanvasRenderer,
PieChart,
TitleComponent,
TooltipComponent,
LegendComponent
]);
export default {
name: "HelloWorld",
components: {
VChart
},
provide: {
[THEME_KEY]: "dark"
},
data() {
return {
option: {
title: {
text: "Traffic Sources",
left: "center"
},
tooltip: {
trigger: "item",
formatter: "{a} <br/>{b} : {c} ({d}%)"
},
legend: {
orient: "vertical",
left: "left",
data: [
"Direct",
"Email",
"Ad Networks",
"Video Ads",
"Search Engines"
]
},
series: [
{
name: "Traffic Sources",
type: "pie",
radius: "55%",
center: ["50%", "60%"],
data: [
{ value: 335, name: "Direct" },
{ value: 310, name: "Email" },
{ value: 234, name: "Ad Networks" },
{ value: 135, name: "Video Ads" },
{ value: 1548, name: "Search Engines" }
],
emphasis: {
itemStyle: {
shadowBlur: 10,
shadowOffsetX: 0,
shadowColor: "rgba(0, 0, 0, 0.5)"
}
}
}
]
}
};
}
};
</script>
<style scoped>
.chart {
height: 400px;
}
</style>
[!IMPORTANT] We encourage manually importing components and charts from ECharts for smaller bundle size. We've built an import code generator to help you with that. You can just paste in your
option
code and we'll generate the precise import code for you.
But if you really want to import the whole ECharts bundle without having to import modules manually, just add this in your code:
import "echarts";
Drop <script>
inside your HTML file and access the component via window.VueECharts
.
<script src="https://cdn.jsdelivr.net/npm/vue@3.4.23"></script>
<script src="https://cdn.jsdelivr.net/npm/echarts@5.4.3"></script>
<script src="https://cdn.jsdelivr.net/npm/vue-echarts@6.7.3"></script>
const app = Vue.createApp(...)
// register globally (or you can do it locally)
app.component('v-chart', VueECharts)
<script src="https://cdn.jsdelivr.net/npm/vue@2.7.16"></script>
<script src="https://cdn.jsdelivr.net/npm/echarts@5.4.3"></script>
<script src="https://cdn.jsdelivr.net/npm/vue-echarts@6.7.3"></script>
// register globally (or you can do it locally)
Vue.component("v-chart", VueECharts);
See more examples here.
init-options: object
Optional chart init configurations. See echarts.init
's opts
parameter here →
Injection key: INIT_OPTIONS_KEY
.
theme: string | object
Theme to be applied. See echarts.init
's theme
parameter here →
Injection key: THEME_KEY
.
option: object
ECharts' universal interface. Modifying this prop will trigger ECharts' setOption
method. Read more here →
💡 When
update-options
is not specified,notMerge: false
will be specified by default when thesetOption
method is called if theoption
object is modified directly and the reference remains unchanged; otherwise, if a new reference is bound tooption
,notMerge: true
will be specified.
update-options: object
Options for updating chart option. See echartsInstance.setOption
's opts
parameter here →
Injection key: UPDATE_OPTIONS_KEY
.
group: string
Group name to be used in chart connection. See echartsInstance.group
here →
autoresize: boolean | { throttle?: number, onResize?: () => void }
(default: false
)
Whether the chart should be resized automatically whenever its root is resized. Use the options object to specify a custom throttle delay (in milliseconds) and/or an extra resize callback function.
loading: boolean
(default: false
)
Whether the chart is in loading state.
loading-options: object
Configuration item of loading animation. See echartsInstance.showLoading
's opts
parameter here →
Injection key: LOADING_OPTIONS_KEY
.
manual-update: boolean
(default: false
)
For performance critical scenarios (having a large dataset) we'd better bypass Vue's reactivity system for option
prop. By specifying manual-update
prop with true
and not providing option
prop, the dataset won't be watched any more. After doing so, you need to retrieve the component instance with ref
and manually call setOption
method to update the chart.
You can bind events with Vue's v-on
directive.
<template>
<v-chart :option="option" @highlight="handleHighlight" />
</template>
Note
Only the
.once
event modifier is supported as other modifiers are tightly coupled with the DOM event system.
Vue-ECharts support the following events:
highlight
→downplay
→selectchanged
→legendselectchanged
→legendselected
→legendunselected
→legendselectall
→legendinverseselect
→legendscroll
→datazoom
→datarangeselected
→timelinechanged
→timelineplaychanged
→restore
→dataviewchanged
→magictypechanged
→geoselectchanged
→geoselected
→geounselected
→axisareaselected
→brush
→brushEnd
→brushselected
→globalcursortaken
→rendered
→finished
→zr:click
zr:mousedown
zr:mouseup
zr:mousewheel
zr:dblclick
zr:contextmenu
See supported events here →
As Vue-ECharts binds events to the ECharts instance by default, there is some caveat when using native DOM events. You need to prefix the event name with native:
to bind native DOM events (or you can use the .native
modifier in Vue 2, which is dropped in Vue 3).
<template>
<v-chart @native:click="handleClick" />
</template>
Vue-ECharts provides provide/inject API for theme
, init-options
, update-options
and loading-options
to help configuring contextual options. eg. for init-options
you can use the provide API like this:
import { THEME_KEY } from 'vue-echarts'
import { provide } from 'vue'
// composition API
provide(THEME_KEY, 'dark')
// options API
{
provide: {
[THEME_KEY]: 'dark'
}
}
import { THEME_KEY } from 'vue-echarts'
// in component options
{
provide: {
[THEME_KEY]: 'dark'
}
}
Note
You need to provide an object for Vue 2 if you want to change it dynamically.
// in component options { data () { return { theme: { value: 'dark' } } }, provide () { return { [THEME_KEY]: this.theme } } }
setOption
→getWidth
→getHeight
→getDom
→getOption
→resize
→dispatchAction
→convertToPixel
→convertFromPixel
→containPixel
→showLoading
→hideLoading
→getDataURL
→getConnectedDataURL
→clear
→dispose
→Static methods can be accessed from echarts
itself.
style-src
or style-src-elem
If you are applying a CSP to prevent inline <style>
injection, you need to use files from dist/csp
directory and include dist/csp/style.css
into your app manually.
💡 Please make sure to read the migration guide for ECharts 5 as well.
The following breaking changes are introduced in vue-echarts@6
:
vue@2.7.0
, @vue/composition-api
is required to be installed to use Vue-ECharts with Vue 2 (and also @vue/runtime-core
for TypeScript support).options
is renamed to option
to align with ECharts itself.option
will respect update-options
configs instead of checking reference change.watch-shallow
is removed. Use manual-update
for performance critical scenarios.mergeOptions
is renamed to setOption
to align with ECharts itself.showLoading
and hideLoading
is removed. Use the loading
and loading-options
props instead.appendData
is removed. (Due to ECharts 5's breaking change.)vue-echarts
. Use those methods from echarts
directly.width
, height
, isDisposed
and computedOptions
) are removed. Use the getWidth
, getHeight
, isDisposed
and getOption
methods instead.100%×100%
size by default, instead of 600×400
.pnpm i
pnpm serve
Open http://localhost:8080
to see the demo.
The Apache Software Foundation Apache ECharts, ECharts, Apache, the Apache feather, and the Apache ECharts project logo are either registered trademarks or trademarks of the Apache Software Foundation.
6.7.3
padding
on the component root doesn't work.FAQs
Vue.js component for Apache ECharts™.
The npm package vue-echarts receives a total of 0 weekly downloads. As such, vue-echarts popularity was classified as not popular.
We found that vue-echarts demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.