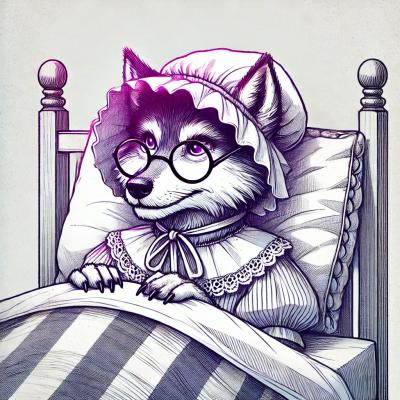
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
vuex-orm-decorators
Advanced tools
Simple Typescript decorators to improve the vuex-orm experience in Typescript by introducing typed property access.
Decorator Syntax for Vuex ORM v0.36
for better type safety and a better experience.
:fire: Quick note before start using this package
Typescript Decorators to simplify Vuex ORM integration in typescript projects. If you are using the vue-module-decorators or vue-property-decorator packages then this will allow you to use the Vuex ORM plugin in the same way.
Using the decorators allows better type safety in your projects by allowing you to create conventional Typescript properties, and annotate them as fields for a better experience. Intellisense in Visual Studio Code just works with the annotations, where it doesn't in the vanilla plugin without boilerplate.
This documentation isn't supposed to be a replacement for the Vuex ORM documentation, if you are unfamiliar with the concepts of Vuex ORM then check out their documentation. I have linked to relevant guide pages in their documentation throughout this documentation.
If you have improvements or contributions to make, I will happily check and merge in pull requests.
First install the version of vuex you want to use, Vuex ORM Decorators works with vuex 3 and vuex 4.
You can install Vuex ORM Decorators via NPM or Yarn
npm install @vuex-orm/core@^0.36
npm install vuex-orm-decorators -D
pnpm add @vuex-orm/core@^0.36
pnpm add vuex-orm-decorators -D
yarn add @vuex-orm/core@^0.36
yarn add vuex-orm-decorators -D
This package targets es2015, if you need to target es5 then you will need to get VUE-CLI to transpile this package.
Models can automatically register themselves in the database. To do so, instead of installing the Vuex ORM Database, install the wrapper provided by this library as follows:
import Vue from 'vue';
import Vuex from 'vuex';
import { ORMDatabase } from 'vuex-orm-decorators'
Vue.use(Vuex);
export default new Vuex.Store({
plugins: [
ORMDatabase.install({ namespace: 'Models' })
]
});
import { createStore } from 'vuex';
import { ORMDatabase } from 'vuex-orm-decorators';
export default createStore({
plugins: [
ORMDatabase.install({ namespace: 'Models' })
]
});
When you use a model it registers itself in the database automatically if it has not already. If you do not want auto registered models, simply install the vanilla database and register them as you would normally.
ExperimentalDecorators
to true.importHelpers: true
in tsconfig.json
.emitHelpers: true
in tsconfig.json
(only required in typescript 2)Add the following to the nuxt.config.js to transpile the library
{
build: {
transpile: ['vuex-orm-decorators']
}
}
If you want to register models automatically do not install Vuex ORM Database, just export plugin from store/index.js
import { ORMDatabase } from 'vuex-orm-decorators'
export const plugins = [ORMDatabase.install({ namespace: 'Models' })]
Out of the box a Vuex ORM Model is defined as:
import { Model } from '@vuex-orm/core';
class User extends Model {
static entity = 'users';
static fields() {
return {
id: this.attr(undefined),
name: this.attr('')
};
}
}
The defined fields don't gain type checking by Typescript in this way because they are never defined as properties of the model class. With this decorator library though it allows you to write the same in the following way to achieve type checking on your queried models:
import { Model } from '@vuex-orm/core';
import { NumberField, OrmModel, StringField } from 'vuex-orm-decorators';
@OrmModel('users')
class User extends Model {
@NumberField() id!: number;
@StringField() name!: string;
}
To create a fully reactive getter/computed, simply add your getters to the model class:
import { Model } from '@vuex-orm/core';
import { NumberField, OrmModel, StringField } from 'vuex-orm-decorators';
@OrmModel('users')
class User extends Model {
@NumberField() id!: number;
@StringField() name!: string;
get lowerName() {
return this.name.toLowerCase();
}
}
You can pass a closure to the 2nd argument of @AttrField
, @StringField
, @NumberField
, @DateField
,
and @BooleanField
decorator. The closure takes the corresponding value as an argument, and you can modify the value
however you want:
import { Model } from '@vuex-orm/core';
import { OrmModel, StringField } from 'vuex-orm-decorators';
@OrmModel('users')
class User extends Model {
@StringField(null, (name) => {
return name.toLowerCase();
}) name!: string;
}
If undefined the default values for the @AttrField
, @StringField
, @NumberField
, @DateField
, and @BooleanField
decorator will be:
@OrmModel('users')
class User extends Model {
// Default value: ''
@AttrField() email!: string;
// Default value: ''
@StringField() name!: string;
// Default value: 0
@NumberField() age!: number;
// Default value: false
@BooleanField() active!: boolean;
// Default value: null
// if can parse the date, then Date instance will be returned
@DateField() created_at!: Date;
}
If you want to set the field as nullable
, pass a null
value as default value, this will also set the isNullable
Type to true
:
@OrmModel('users')
class User extends Model {
// Default value: null, isNullable: true
@AttrField(null) email!: string;
// Default value: null, isNullable: true
@StringField(null) name!: string;
// Default value: null, isNullable: true
@NumberField(null) age!: number;
// Default value: null, isNullable: true
@BooleanField(null) active!: boolean;
// Default value: null, isNullable: true
@DateField(null) deleted_at!: Date;
}
Rather than setting a primary key by setting the
static property primaryKey
with the magic string name of the property you want to be the primary key, you can simply
annotate the property with the @PrimaryKey
decorator as follows:
No need using
@PrimaryKey
decorator on propertyid
. Propertyid
is by default theprimaryKey
.
import { Model } from '@vuex-orm/core';
import { NumberField, OrmModel, PrimaryKey, StringField } from 'vuex-orm-decorators';
@OrmModel('users')
class User extends Model {
@PrimaryKey()
@NumberField() uuid!: number;
@StringField() name!: string;
}
In this example the property uuid
replaces the default id
property as the primary key.
You can also define a composite primary key by annotating several properties with the @PrimaryKey
decorator as follows:
import { Model } from '@vuex-orm/core';
import { OrmModel, PrimaryKey, StringField } from 'vuex-orm-decorators';
@OrmModel('users')
class User extends Model {
@PrimaryKey()
@StringField() public id!: string;
@PrimaryKey()
@StringField() public voteId!: string;
}
If your model extends a base model, then STI inheritance needs to be used. The base entity name needs to be provided as the second argument to the ORMModel decorator and as third argument provide the discriminator fields:
Person : Base Entity
@OrmModel('persons')
class Person extends Model {
@NumberField() id!: number;
@StringField() name!: string;
}
Teenager extends Person
@OrmModel('teenagers', 'persons', {
PERSON: Person,
TEENAGER: Teenager
})
class Teenager extends Person {
@StringField() school!: string;
}
Adult extends Person
@OrmModel('adults', 'persons', {
PERSON: Person,
ADULT: Adult
})
class Adult extends Person {
@StringField() job!: string;
}
Now, you can create mixed types of records at once.
Person.insert({
data: [
{ type: 'PERSON', id: 1, name: 'John Doe' },
{ type: 'TEENAGER', id: 2, name: 'Jane Doe', school: '22nd Best School' },
{ type: 'ADULT', id: 3, name: 'Jane Roe', job: 'Software Engineer' }
]
});
You may define a
static typeKey
on the base entity of your hierarchy if you want to change the default discriminator field name:
@OrmModel('persons')
class Person extends Model {
/**
* The discriminator key to be used for the model when inheritance is used.
*/
static typeKey = 'PERSON';
@NumberField() id!: number;
@StringField() name!: string;
}
@PrimaryKey
sets field as Primary Key to be
used for the model.@UidField
creates a UID Type field@AttrField
creates a Generic Type field@DateField
creates a Date
instance fieldLike the Vuex ORM library, you can create primitive fields using the following decorators:
@StringField
creates a string field@NumberField
creates a number field@BooleanField
creates a boolean fieldYou can create all relationships defined in the Vuex ORM library. All the relationship decorators take the exact same arguments as the vanilla Vuex ORM library static functions.
@HasManyField
creates a HasMany relationship
field
@HasOneField
creates a HasOne relationship field
@BelongsToField
creates a BelongsTo
relationship field
@HasManyByField
creates a HasManyBy relationship
field
@HasManyThroughField
creates
a HasManyThrough relationship field
@BelongsToManyField
creates a BelongsToMany
relationship field
@MorphOneField
creates a MorphOne
relationship field
@MorphToField
creates a MorphTo
relationship field
@MorphManyField
creates a MorphMany
relationship field
@MorphToManyField
creates
a MorphToMany relationship field
@MorphedByManyField
creates
a MorphedByMany
relationship field
Vuex ORM Decorators is open-sourced software licensed under the MIT License.
FAQs
Simple Typescript decorators to improve the vuex-orm experience in Typescript by introducing typed property access.
The npm package vuex-orm-decorators receives a total of 0 weekly downloads. As such, vuex-orm-decorators popularity was classified as not popular.
We found that vuex-orm-decorators demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.