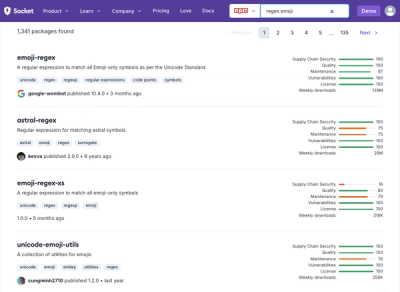
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
This node.js module is useful if you want to load other modules and be sure all warming code is executed before you continue.
Install module into own project
# npm install --save warm
Create new class and call .parallel
method
Warm = requre('warm');
new Warm('./database', './redis').parallel(function (err) {
if(err) { throw err; }
console.log('Initial code was executed in all described modules');
});
Modules database
and redis
should return special method (.init
by default), which resolve promise or call callback on finish. See Modules
You can pass additional options like plain object: new Warm({ ... }, 'module1', ...)
:
Name | Default | Description |
---|---|---|
.expect | promise | will init method inform about finish with promise (reject/resolve it), or will fire callback with error (expect: 'callback' ) |
.path | '' | prefix for requiring modules |
.strict | true | should return error if init method not found in module |
.init | init | init method name |
Warm = requre('warm');
// load modules in array or additional parameters with options
options1 = {
expect: 'callback'
}
options2 = {
strict: true
}
new Warm(options1, ['./module1', './module2'], './module3', options2).parallel(console.log);
// execute already loaded module
new Warm(require('./module3')).parallel(console.log);
// handle promise
new Warm('./module1').parallel().then(function () {
console.log('loaded');
}).catch(function (err) {
console.log('error:', err);
})
Module should contain method .init
(or other, described in options) which will return promise or file callback. Example:
module.exports = function () {
return 'i will not fire';
};
module.exports.init = function (callback) {
setTimeout(function () {
console.log('>>> i will fire durning warming');
callback();
}, 100);
};
Additional samples can be found in test folder
FAQs
warm up node modules before using
We found that warm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.