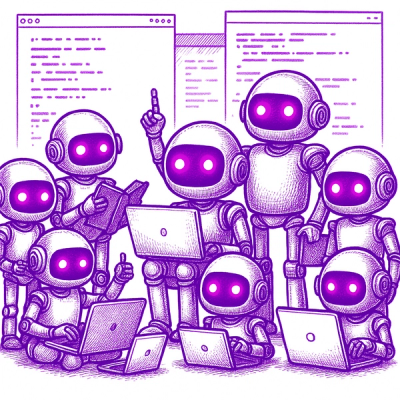
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
The web-push npm package is a Node.js library that allows you to send push notifications to web applications. It implements the Web Push Protocol and is commonly used to send notifications to users' browsers.
Generate VAPID keys
This feature allows you to generate VAPID (Voluntary Application Server Identification) keys, which are used to authenticate your server with the push service.
const webpush = require('web-push');
const vapidKeys = webpush.generateVAPIDKeys();
console.log(vapidKeys);
Set VAPID details
This feature allows you to set the VAPID details, including the contact email and the public and private VAPID keys, which are necessary for sending authenticated push notifications.
const webpush = require('web-push');
webpush.setVapidDetails(
'mailto:example@yourdomain.org',
'YOUR_PUBLIC_VAPID_KEY',
'YOUR_PRIVATE_VAPID_KEY'
);
Send a push notification
This feature allows you to send a push notification to a subscribed client. You need to provide the subscription object and the payload you want to send.
const webpush = require('web-push');
const subscription = {
endpoint: 'https://fcm.googleapis.com/fcm/send/abc123',
keys: {
p256dh: 'YOUR_P256DH_KEY',
auth: 'YOUR_AUTH_KEY'
}
};
const payload = JSON.stringify({ title: 'Hello', body: 'World' });
webpush.sendNotification(subscription, payload)
.then(response => console.log('Sent:', response))
.catch(error => console.error('Error:', error));
push-notifications is a Node.js library that supports multiple push notification services, including web push, Apple Push Notification Service (APNs), and Firebase Cloud Messaging (FCM). It offers a more versatile solution for developers who need to send notifications across different platforms.
Web push requires that push messages triggered from a backend be done via the Web Push Protocol and if you want to send data with your push message, you must also encrypt that data according to the Message Encryption for Web Push spec.
This module makes it easy to send messages and will also handle legacy support for browsers relying on GCM for message sending / delivery.
Installation is simple, just install via npm.
npm install web-push --save
The common use case for this library is an application server using a GCM API key and VAPID keys.
const webpush = require('web-push');
// VAPID keys should be generated only once.
const vapidKeys = webpush.generateVAPIDKeys();
webpush.setGCMAPIKey('<Your GCM API Key Here>');
webpush.setVapidDetails(
'mailto:example@yourdomain.org',
vapidKeys.publicKey,
vapidKeys.privateKey
);
// This is the same output of calling JSON.stringify on a PushSubscription
const pushSubscription = {
endpoint: '.....',
keys: {
auth: '.....',
p256dh: '.....'
}
};
webpush.sendNotification(pushSubscription, 'Your Push Payload Text');
When subscribing to push messages, you'll need to pass your VAPID key, which you can do like so:
registration.pushManager.subscribe({
userVisibleOnly: true,
applicationServerKey: '<Your Public Key from generateVAPIDKeys()>'
});
You can install web-push
globally and use it for sending notifications
and / or generating VAPID keys.
Install like so:
npm install web-push -g
Then you can run the following commands:
Usage:
web-push send-notification --endpoint=<url> [--key=<browser key>] [--auth=<auth secret>] [--payload=<message>] [--encoding=<aesgcm | aes128gcm>] [--ttl=<seconds>] [--vapid-subject=<vapid subject>] [--vapid-pubkey=<public key url base64>] [--vapid-pvtkey=<private key url base64>] [--proxy=<http proxy uri>] [--gcm-api-key=<api key>]
web-push generate-vapid-keys [--json]
Example of send notification:
> web-push generate-vapid-keys --json
> {"publicKey":"BGtkbcjrO12YMoDuq2sCQeHlu47uPx3SHTgFKZFYiBW8Qr0D9vgyZSZPdw6_4ZFEI9Snk1VEAj2qTYI1I1YxBXE","privateKey":"I0_d0vnesxbBSUmlDdOKibGo6vEXRO-Vu88QlSlm5j0"}
The subscription value:
{
"endpoint": "https://fcm.googleapis.com/fcm/send/d61c5u920dw:APA91bEmnw8utjDYCqSRplFMVCzQMg9e5XxpYajvh37mv2QIlISdasBFLbFca9ZZ4Uqcya0ck-SP84YJUEnWsVr3mwYfaDB7vGtsDQuEpfDdcIqOX_wrCRkBW2NDWRZ9qUz9hSgtI3sY",
"expirationTime": null,
"keys": {
"p256dh": "BL7ELU24fJTAlH5Kyl8N6BDCac8u8li_U5PIwG963MOvdYs9s7LSzj8x_7v7RFdLZ9Eap50PiiyF5K0TDAis7t0",
"auth": "juarI8x__VnHvsOgfeAPHg"
}
}
The command example:
web-push send-notification \
--endpoint=https://fcm.googleapis.com/fcm/send/d61c5u920dw:APA91bEmnw8utjDYCqSRplFMVCzQMg9e5XxpYajvh37mv2QIlISdasBFLbFca9ZZ4Uqcya0ck-SP84YJUEnWsVr3mwYfaDB7vGtsDQuEpfDdcIqOX_wrCRkBW2NDWRZ9qUz9hSgtI3sY \
--key=BL7ELU24fJTAlH5Kyl8N6BDCac8u8li_U5PIwG963MOvdYs9s7LSzj8x_7v7RFdLZ9Eap50PiiyF5K0TDAis7t0 \
--auth=juarI8x__VnHvsOgfeAPHg \
--vapid-subject=mailto:example@qq.com \
--vapid-pubkey=BGtkbcjrO12YMoDuq2sCQeHlu47uPx3SHTgFKZFYiBW8Qr0D9vgyZSZPdw6_4ZFEI9Snk1VEAj2qTYI1I1YxBXE \
--vapid-pvtkey=I0_d0vnesxbBSUmlDdOKibGo6vEXRO-Vu88QlSlm5j0 \
--payload=Hello
const pushSubscription = {
endpoint: '< Push Subscription URL >',
keys: {
p256dh: '< User Public Encryption Key >',
auth: '< User Auth Secret >'
}
};
const payload = '< Push Payload String >';
const options = {
gcmAPIKey: '< GCM API Key >',
vapidDetails: {
subject: '< \'mailto\' Address or URL >',
publicKey: '< URL Safe Base64 Encoded Public Key >',
privateKey: '< URL Safe Base64 Encoded Private Key >'
},
timeout: <Number>
TTL: <Number>,
headers: {
'< header name >': '< header value >'
},
contentEncoding: '< Encoding type, e.g.: aesgcm or aes128gcm >',
urgency:'< Default is "normal" >',
topic:'< Use a maximum of 32 characters from the URL or filename-safe Base64 characters sets. >',
proxy: '< proxy server options >',
agent: '< https.Agent instance >'
}
webpush.sendNotification(
pushSubscription,
payload,
options
);
Note:
sendNotification()
you don't need to define a payload, and this method will work without a GCM API Key and / or VAPID keys if the push service supports it.
Push Subscription
The first argument must be an object containing the details for a push subscription.
The expected format is the same output as JSON.stringify'ing a PushSubscription in the browser.
Payload
The payload is optional, but if set, will be the data sent with a push message.
This must be either a string or a node Buffer.
Note: In order to encrypt the payload, the pushSubscription must have a keys object with p256dh and auth values.
Options
Options is an optional argument that if defined should be an object containing any of the following values defined, although none of them are required.
setGCMAPIKey()
.https.request
method. If the proxy
options defined, agent
will be ignored!Note: As of this writing, if a push notification request contains a VAPID
subject
referencing anhttps://localhost
URI (set either using theoptions
argument or via the globalsetVapidDetails()
method), Safari's push notification endpoint rejects the request with aBadJwtToken
error.
A promise that resolves if the notification was sent successfully with details of the request, otherwise it rejects.
In both cases, resolving or rejecting, you'll be able to access the following values on the returned object or error.
const vapidKeys = webpush.generateVAPIDKeys();
// Prints 2 URL Safe Base64 Encoded Strings
console.log(vapidKeys.publicKey, vapidKeys.privateKey);
None.
Returns an object with publicKey and privateKey values which are URL Safe Base64 encoded strings.
Note: You should create these keys once, store them and use them for all future messages you send.
webpush.setGCMAPIKey('Your GCM API Key');
This method expects the GCM API key that is linked to the gcm_sender_id
in
your web app manifest.
You can use a GCM API Key from the Google Developer Console or the Cloud Messaging tab under a Firebase Project.
None.
webpush.setVapidDetails(
'mailto:user@example.org',
process.env.VAPID_PUBLIC_KEY,
process.env.VAPID_PRIVATE_KEY
);
Globally sets the application's VAPID subject, public key, and private key, to be used in subsequent calls to sendNotification()
and generateRequestDetails()
that don't specifically override them in their options
argument.
The setVapidDetails
method expects the following input:
https:
or mailto:
URI (as per the VAPID spec).None.
const pushSubscription = {
endpoint: 'https://....',
keys: {
p256dh: '.....',
auth: '.....'
}
};
webPush.encrypt(
pushSubscription.keys.p256dh,
pushSubscription.keys.auth,
'My Payload',
'aes128gcm'
)
.then(encryptionDetails => {
});
Encrypts the payload according to the Message Encryption for Web Push standard.
(sendNotification will automatically encrypt the payload for you, so if you use sendNotification you don't need to worry about it).
The encrypt()
method expects the following input:
This method returns an object with the following fields:
const parsedUrl = url.parse(subscription.endpoint);
const audience = parsedUrl.protocol + '//' +
parsedUrl.hostname;
const vapidHeaders = vapidHelper.getVapidHeaders(
audience,
'mailto: example@web-push-node.org',
vapidDetails.publicKey,
vapidDetails.privateKey,
'aes128gcm'
);
The getVapidHeaders() method will take in the values needed to create an Authorization and Crypto-Key header.
The getVapidHeaders()
method expects the following input:
This method returns an object with the following fields:
const pushSubscription = {
endpoint: '< Push Subscription URL >';
keys: {
p256dh: '< User Public Encryption Key >',
auth: '< User Auth Secret >'
}
};
const payload = '< Push Payload String >';
const options = {
gcmAPIKey: '< GCM API Key >',
vapidDetails: {
subject: '< \'mailto\' Address or URL >',
publicKey: '< URL Safe Base64 Encoded Public Key >',
privateKey: '< URL Safe Base64 Encoded Private Key >',
}
TTL: <Number>,
headers: {
'< header name >': '< header value >'
},
contentEncoding: '< Encoding type, e.g.: aesgcm or aes128gcm >',
urgency:'< Default is normal "Defult" >',
topic:'< Use a maximum of 32 characters from the URL or filename-safe Base64 characters sets. >',
proxy: '< proxy server options >'
}
try {
const details = webpush.generateRequestDetails(
pushSubscription,
payload,
options
);
} catch (err) {
console.error(err);
}
Note: When calling
generateRequestDetails()
the payload argument does not need to be defined, passing in null will return no body and exclude any unnecessary headers. Headers related to the GCM API Key and / or VAPID keys will be included if supplied and required.
Push Subscription
The first argument must be an object containing the details for a push subscription.
The expected format is the same output as JSON.stringify'ing a PushSubscription in the browser.
Payload
The payload is optional, but if set, will be encrypted and a Buffer
will be returned via the payload
parameter.
This argument must be either a string or a node Buffer.
Note: In order to encrypt the payload, the pushSubscription must have a keys object with p256dh and auth values.
Options
Options is an optional argument that if defined should be an object containing any of the following values defined, although none of them are required.
setGCMAPIKey()
.An object containing all the details needed to make a network request, the object will contain:
Browser | Push without Payload | Push with Payload | VAPID | Notes |
---|---|---|---|---|
Chrome | ✓ v42+ | ✓ v50+ | ✓ v52+ | In v51 and less, the `gcm_sender_id` is needed to get a push subscription. |
Edge | ✓ v17+ (April 2018) | ✓ v17+ (April 2018) | ✓ v17+ (April 2018) | |
Firefox | ✓ v44+ | ✓ v44+ | ✓ v46+ | |
Opera | ✓ v39+ * | ✓ v39+ * | ✗ |
* Opera supports push on Android but not on desktop.
The `gcm_sender_id` is needed to get a push subscription. |
Safari | ✓ v16+ | ✓ v16+ | ✓ v16+ | Safari 16 in macOS 13 or later |
Samsung Internet Browser | ✓ v4.0.10-53+ | ✓ v5.0.30-40+ | ✗ | The `gcm_sender_id` is needed to get a push subscription. |
MDN
There's an example on MDN.
Also, the Service Worker Cookbook is full of Web Push examples using this library.
Prerequisites:
- Java JDK or JRE (http://www.oracle.com/technetwork/java/javase/downloads/index.html)
To run tests:
npm test
FAQs
Web Push library for Node.js
The npm package web-push receives a total of 186,221 weekly downloads. As such, web-push popularity was classified as popular.
We found that web-push demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.