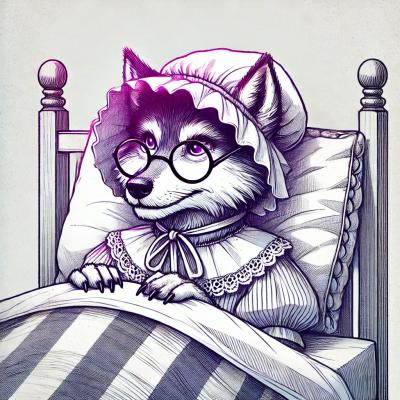
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
web-ui-pack
Advanced tools
Web package with high scalable WebComponents and helpers
.jsx/.tsx
supportUsing npm:
npm install web-ui-pack
You can see demo here or just clone repo and run npm i & npm start
Common rules:
WUP....Element
and <wup-...>
(for html-tags)$...
(events $onShow
, $onHide
, methods $show
, $hide
, props like $isOpen
etc.)$defaults
(common options for current class) and personal $options
(per each component). See details in example$options
are observed. So changing options affects on component immediately (every component has static observedOptions
as set of watched options)web-ui-pack
directly. Instead use web-ui-pack/path-to-element
or setup sideEffects in package.json (for webpack)jsx/tsx
instead of className
use class
attribute (React issue)got...
(gotReady, gotRemoved)WUPPopupElement, <wup-popup />
This is the most smart & crazy element you've ever seen (see Demo)
Typescript
import WUPPopupElement, { ShowCases } from "web-ui-pack/popup/popupElement";
// redefine some defaults; WARN: you can change placement rules here without changing $options per each element!!!
WUPPopupElement.$defaults.offset = [2, 2];
WUPPopupElement.$defaults.minWidthByTarget = true;
WUPPopupElement.$defaults.arrowEnable = true;
// create element
const el = document.createElement("wup-popup");
// WARN el.$options is a observable-clone of WUPPopupElement.$defaults
// WARN: ShowCases is const enum and import ShowCases available only in Typescript
el.$options.showCase = ShowCases.onClick | ShowCases.onFocus; // show popup by target.click and/or target.focus events
el.$options.target = document.querySelector("button");
/*
Placement can be $top, $right, $bottom, $left (top - above at the target etc.)
every placement has align options: $start, $middle, $end (left - to align at start of target)
also you can set $adjust to allow Reduce popup to fit layout
*/
el.$options.placement = [
WUPPopupElement.$placements.$top.$middle; // place at the top of target and align by vertical line
WUPPopupElement.$placements.$bottom.$middle.$adjust, // adjust means 'ignore align to fit layout`
WUPPopupElement.$placements.$bottom.$middle.$adjust.$resizeHeight, // resize means 'allow to resize to fit layout'
]
document.body.append(el);
HTML, JSX, TSX
<button id="btn1">Target</button>
<!-- You can skip pointing attribute 'target' if popup appended after target -->
<wup-popup target="#btn1" placement="top-start">Some content here</wup-popup>
How to extend/override
/// popup.ts
class Popup extends WUPPopupElement {
// take a look on definition of WUPPopupElement and you will find internals
protected override canShow(showCase: WUPPopup.ShowCases): boolean {
if (showCase === WUPPopup.ShowCases.onHover) {
return false;
}
return true;
}
}
const tagName = "ext-popup";
customElements.define(tagName, Popup);
// That's it. New Popup with custom tag 'ext-popup' is ready
// add for intellisense (for *.ts only)
declare global {
// add element to document.createElement
interface HTMLElementTagNameMap {
[tagName]: Popup;
}
// add element for tsx/jsx intellisense
namespace JSX {
interface IntrinsicElements {
[tagName]: IntrinsicElements["wup-popup"];
}
}
}
use import focusFirst from "web-ui-pack/helpers/focusFirst"
etc.
WARN: don't use import {focusFirst} from "web-ui-pack;
because in this case the whole web-ui-pack module traps in compilation of dev-bundle and increases time of compilation
Set focus on parent itself or first possible element inside
Find first parent with active scroll X/Y
nestedProperty.set(obj, "value.nestedValue", 1) sets obj.value.nestedValue = 1
nestedProperty.get(obj, "nestedValue1.nestVal2") returns value from obj.nestedValue1.nestVal2
converts object to observable (via Proxy) to allow listen for changes
More strict (for Typescript) wrapper of addEventListener() that returns callback with removeListener()
Fires when element/children takes focus once (fires again after onFocusLost on element)
Fires when element/children completely lost focus
Spy on method-call of object
Produce Promise during for "no less than pointed time"; it helps for avoding spinner blinking during the very fast api-request in case: pending > waitResponse > resetPending
Changes camelCase, snakeCase, kebaCase text to user-friendly
import observer from "web-ui-pack/helpers/observer";
const rawNestedObj = { val: 1 };
const raw = { date: new Date(), period: 3, nestedObj: rawNestedObj, arr: ["a"] };
const obj = observer.make(raw);
const removeListener = observer.onPropChanged(obj, (e) => console.warn("prop changed", e)); // calls per each changing
const removeListener2 = observer.onChanged(obj, (e) => console.warn("object changed", e)); // calls once after changing of bunch props
obj.period = 5; // fire onPropChanged
obj.date.setHours(0, 0, 0, 0); // fire onPropChanged
obj.nestedObj.val = 2; // fire onPropChanged
obj.arr.push("b"); // fire onPropChanged
obj.nestedObj = rawNestedObj; // fire onPropChanged
obj.nestedObj = rawNestedObj; // WARNING: it fire events again because rawNestedObj !== obj.nestedObj
removeListener(); // unsubscribe
removeListener2(); // unsubscribe
// before timeout will be fired onChanged (single time)
setTimeout(() => {
console.warn("WARNING: raw vs observable", {
equal: raw === obj,
equalByValueOf: raw.valueOf() === obj.valueOf(),
isRawObserved: observer.isObserved(raw),
isObjObserved: observer.isObserved(obj),
});
// because after assigning to observable it converted to observable also
console.warn("WARNING: rawNestedObj vs observable", {
equal: rawNestedObj === obj.nestedObj,
equalByValueOf: rawNestedObj.valueOf() === obj.nestedObj.valueOf(),
isRawObserved: observer.isObserved(rawNestedObj),
isNestedObserved: observer.isObserved(obj.nestedObj),
});
});
observed
is converted to observed
alsolength
; also sort
/reverse
triggers eventsvalueOf()
so you maybe interested in custom valueOf to avoid unexpected issues0.0.4 (Apr 1, 2022)
FAQs
Web package with UI elements
The npm package web-ui-pack receives a total of 95 weekly downloads. As such, web-ui-pack popularity was classified as not popular.
We found that web-ui-pack demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.