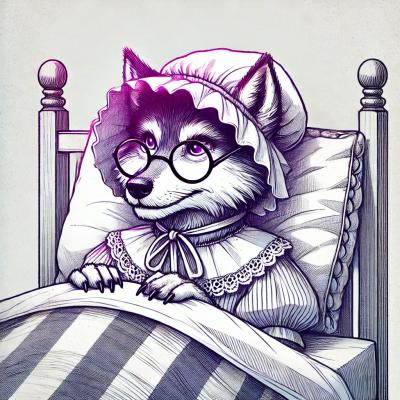
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
web-ui-pack
Advanced tools
Web package with high scalable WebComponents and helpers
You can see demo here or just clone repo and run npm i & npm start
wupdark
(<body wupdark>
) and define general background & text colors.jsx/.tsx
support (for React/Vue)It's developed with Typescript and has huge built-in documentation (JSDoc). Every method,property,event is documented well so you don't need extra resource to take an example to implement or configure elements. In build-result without comments you will see that it's small-enough
Install with npm npm install web-ui-pack
Add import WUPPopupElement from "web-ui-pack";
into main.js file
For usage with React see CODESTYLE.md
For usage with HTML + VSCode extend VSCode settings
// .vscode/settings.json
{
// ...
"html.customData": ["node_modules/web-ui-pack/types.html.json"]
}
Type <wup w-
to see suggestions (if it doesn't work reload VSCode). More details below
multiSelect
Common rules:
WUP..Element
, WUP..Control
and has <wup-...>
html-tags$...
(events $onShow
, $onHide
, methods $show
, $hide
, props like $isShown
etc.)$defaults
(common options for current class) and personal $options
(per each component). See details in example$options
are observed. So changing options affects on component immediately after empty timeout (every component has static observedOptions
as set of watched options)attributes
updates $options
automatically. So document.querySelector('wup-spin').$options.inline
equal to <wup-spin inline />
web-ui-pack
directly (due to tree-shaking can be not smart enough). Instead use web-ui-pack/path-to-element
exclude: /node_modules\/(?!(web-ui-pack)\/).*/
for babel-loader)jsx/tsx
instead of className
use class
attribute (React issue)$options
, but if you change some option it removes related attribute (for performance reasons). Better to avoid usage attributes at allgot...
(gotReady, gotRemoved)Check how you can use every element/control (popupElement for example) Also check code style example
Typescript
import WUPPopupElement, { ShowCases } from "web-ui-pack/popup/popupElement";
// redefine some defaults; WARN: you can change placement rules here without changing $options per each element!!!
WUPPopupElement.$defaults.offset = [2, 2];
WUPPopupElement.$defaults.minWidthByTarget = true;
WUPPopupElement.$defaults.arrowEnable = true;
// create element
const el = document.createElement("wup-popup");
// WARN el.$options is a observable-clone of WUPPopupElement.$defaults
// WARN: ShowCases is const enum and import ShowCases available only in Typescript
el.$options.showCase = ShowCases.onClick | ShowCases.onFocus; // show popup by target.click and/or target.focus events
el.$options.target = document.querySelector("button");
/*
Placement can be $top, $right, $bottom, $left (top - above at the target etc.)
every placement has align options: $start, $middle, $end (left - to align at start of target)
also you can set $adjust to allow Reduce popup to fit layout
*/
el.$options.placement = [
WUPPopupElement.$placements.$top.$middle; // place at the top of target and align by vertical line
WUPPopupElement.$placements.$bottom.$middle.$adjust, // adjust means 'ignore align to fit layout`
WUPPopupElement.$placements.$bottom.$middle.$adjust.$resizeHeight, // resize means 'allow to resize to fit layout'
]
document.body.append(el);
HTML, JSX, TSX
<button id="btn1">Target</button>
<!-- You can skip pointing attribute 'target' if popup appended after target -->
<wup-popup w-target="#btn1" w-placement="top-start">Some content here</wup-popup>
How to extend/override
/// popup.ts
// you can override via prototypes
const original = WUPPopupElement.prototype.goShow;
WUPPopupElement.prototype.goShow = function customGoShow() {
if (window.isBusy) {
return null;
}
return original(...arguments);
};
/*** OR create extended class ***/
class Popup extends WUPPopupElement {
// take a look on definition of WUPPopupElement and you will find internals
protected override goShow(showCase: WUPPopup.ShowCases): boolean {
if (showCase === WUPPopup.ShowCases.onHover) {
return false;
}
return super.goShow(showCase);
}
}
const tagName = "ext-popup";
customElements.define(tagName, Popup);
// That's it. New Popup with custom tag 'ext-popup' is ready
// add for intellisense (for *.ts only)
declare global {
// add element to document.createElement
interface HTMLElementTagNameMap {
[tagName]: Popup;
}
// add element for tsx/jsx intellisense
namespace JSX {
interface IntrinsicElements {
[tagName]: IntrinsicElements["wup-popup"];
}
}
}
use import focusFirst from "web-ui-pack/helpers/focusFirst"
etc.
WARN: don't use import {focusFirst} from "web-ui-pack;
because in this case the whole web-ui-pack module traps in compilation of dev-bundle and increases time of compilation
Animate (show/hide) element as dropdown via scale and counter-scale for children
Animate (show/hide) every element via moving from target to own position
Compare by Date-values without Time
Copy hh:mm:ss.fff part from B to A
Returns parsed date from string based on pointed format
Returns a string representation of a date-time according to pointed format
Find first parent with active scroll X/Y
Find all parents with active scroll X/Y
Set focus on element or first possible nested element
Check if element is visible in scrollable parents
Sum without float-precision-issue
Scale value from one range to another
nestedProperty.set(obj, "value.nestedValue", 1) sets obj.value.nestedValue = 1
nestedProperty.get(obj, "nested.val2", out?: {hasProp?: boolean} ) returns value from obj.nested.val2
deep cloning object
converts object to observable (via Proxy) to allow listen for changes
More strict (for Typescript) wrapper of addEventListener() that returns callback with removeListener()
Fires when element/children takes focus once (fires again after onFocusLost on element)
Handles wheel & touch events for custom scrolling
Returns callback when scrolling is stopped (via checking scroll position every frame-render)
Fires when element/children completely lost focus
Spy on method-call of object
Produce Promise during for "no less than pointed time"; it helps for avoding spinner blinking during the very fast api-request in case: pending > waitResponse > resetPending
Scroll the HTMLElement's parent container such that the element is visible to the user and return promise by animation end
Class makes pointed element scrollable and implements carousel-scroll behavior (appends new items during the scrolling). Supports swipe/pageUp/pageDown/mouseWheel events.
Returns count of chars in lower case (for any language with ignoring numbers, symbols)
Returns count of chars in upper case (for any language with ignoring numbers, symbols)
Changes camelCase, snakeCase, kebaCase text to user-friendly
Locale-object with definitions related to user-locale
Plane time object without date
Be sure that you familiar with common rules
web-ui-pack is compiled to ESNext. So some features maybe don't exist in browsers. To resolve it include the lib into babel-loader (for webpack check module.rules...exclude sections
// webpack.config.js { test: /\.(js|jsx)$/, exclude: (() => { // these packages must be included to change according to browserslist const include = ["web-ui-pack"]; return (v) => v.includes("node_modules") && !include.some((lib) => v.includes(lib)); })(), use: [ "babel-loader", ], },
<wup-popup />
etcIt's possible if you missed import or it was removed by optimizer of wepback etc. To fix this you need to force import at least once
import { WUPSelectControl, WUPTextControl } from "web-ui-pack"; // this force webpack not ignore imports (if imported used only as html-tags without direct access) const sideEffect = WUPTextControl && WUPSelectControl; !sideEffect && console.error("Missed"); // It's required otherwise import is ignored by webpack // or WUPTextControl.$defaults.validateDebounceMs = 500; WUPSelectControl.$defaults.validateDebounceMs = 500; // etc.
see demo/faq
FAQs
Web package with UI elements
We found that web-ui-pack demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.