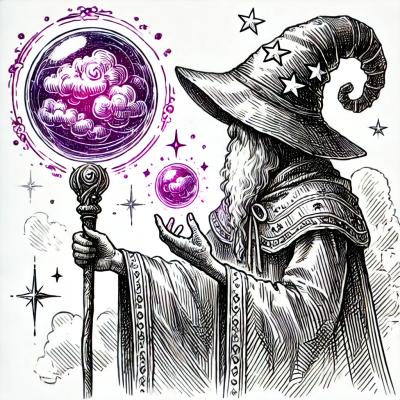
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
web3-core-requestmanager
Advanced tools
The web3-core-requestmanager package is a core component of the Web3.js library, which is used to interact with Ethereum blockchain nodes. It provides the functionality to send JSON-RPC requests to Ethereum nodes and handle the responses. This package is essential for managing the communication between the client application and the Ethereum network.
Sending JSON-RPC Requests
This feature allows you to send JSON-RPC requests to an Ethereum node. In this example, a request is sent to get the current block number from the Ethereum node.
const Web3 = require('web3');
const RequestManager = require('web3-core-requestmanager');
const web3 = new Web3('http://localhost:8545');
const requestManager = new RequestManager(web3.currentProvider);
const payload = {
jsonrpc: '2.0',
method: 'eth_blockNumber',
params: [],
id: 1
};
requestManager.send(payload, (error, result) => {
if (error) {
console.error('Error:', error);
} else {
console.log('Block Number:', result.result);
}
});
Batch Requests
This feature allows you to send multiple JSON-RPC requests in a single batch. In this example, two requests are sent: one to get the current block number and another to get the current gas price.
const Web3 = require('web3');
const RequestManager = require('web3-core-requestmanager');
const web3 = new Web3('http://localhost:8545');
const requestManager = new RequestManager(web3.currentProvider);
const batch = new web3.BatchRequest();
batch.add(requestManager.send({
jsonrpc: '2.0',
method: 'eth_blockNumber',
params: [],
id: 1
}, (error, result) => {
if (error) {
console.error('Error:', error);
} else {
console.log('Block Number:', result.result);
}
}));
batch.add(requestManager.send({
jsonrpc: '2.0',
method: 'eth_gasPrice',
params: [],
id: 2
}, (error, result) => {
if (error) {
console.error('Error:', error);
} else {
console.log('Gas Price:', result.result);
}
}));
batch.execute();
The ethers.js library is a complete and compact library for interacting with the Ethereum blockchain and its ecosystem. It provides similar functionalities to web3-core-requestmanager, such as sending JSON-RPC requests and managing communication with Ethereum nodes. Ethers.js is known for its simplicity and ease of use.
The web3.js library is a comprehensive library for interacting with the Ethereum blockchain. It includes the web3-core-requestmanager package as one of its core components. Web3.js provides a wide range of functionalities, including sending JSON-RPC requests, managing accounts, and interacting with smart contracts.
Ethjs is a lightweight and modular library for interacting with the Ethereum blockchain. It provides similar functionalities to web3-core-requestmanager, such as sending JSON-RPC requests and managing communication with Ethereum nodes. Ethjs is designed to be simple and efficient, making it a good choice for lightweight applications.
This is a sub-package of web3.js.
This requestmanager package is used by most web3.js packages.
Please read the documentation for more.
npm install web3-core-requestmanager
const Web3WsProvider = require('web3-providers-ws');
const Web3RequestManager = require('web3-core-requestmanager');
const requestManager = new Web3RequestManager(new Web3WsProvider('ws://localhost:8546'));
FAQs
Web3 module to handle requests to external providers.
The npm package web3-core-requestmanager receives a total of 294,848 weekly downloads. As such, web3-core-requestmanager popularity was classified as popular.
We found that web3-core-requestmanager demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.