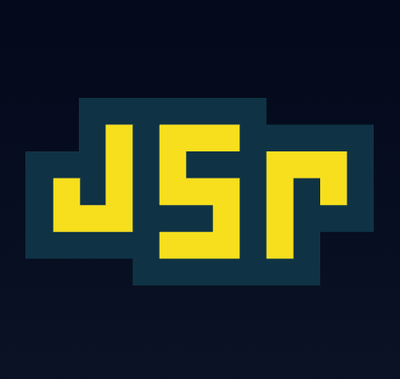
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
web3-core
Advanced tools
The web3-core package is a core module of the Web3.js library, which is used to interact with the Ethereum blockchain. It provides essential functionalities such as managing accounts, sending transactions, and interacting with smart contracts.
Managing Accounts
This feature allows you to create and manage Ethereum accounts. The code sample demonstrates how to create a new Ethereum account using the web3-core package.
const Web3 = require('web3');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
// Create a new account
const account = web3.eth.accounts.create();
console.log(account);
Sending Transactions
This feature allows you to send transactions on the Ethereum network. The code sample demonstrates how to sign and send a transaction using the web3-core package.
const Web3 = require('web3');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
const transaction = {
to: '0xRecipientAddress',
value: web3.utils.toWei('0.1', 'ether'),
gas: 2000000
};
web3.eth.accounts.signTransaction(transaction, 'YOUR_PRIVATE_KEY').then(signedTx => {
web3.eth.sendSignedTransaction(signedTx.rawTransaction).on('receipt', console.log);
});
Interacting with Smart Contracts
This feature allows you to interact with smart contracts deployed on the Ethereum network. The code sample demonstrates how to call a method on a smart contract using the web3-core package.
const Web3 = require('web3');
const web3 = new Web3('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID');
const contractABI = [/* ABI array */];
const contractAddress = '0xContractAddress';
const contract = new web3.eth.Contract(contractABI, contractAddress);
contract.methods.myMethod().call().then(console.log);
The ethers.js library is a complete and compact library for interacting with the Ethereum blockchain and its ecosystem. It provides similar functionalities to web3-core, such as managing accounts, sending transactions, and interacting with smart contracts. Ethers.js is known for its simplicity and ease of use.
Truffle Contract is a library that provides a better abstraction for interacting with Ethereum smart contracts. It is part of the Truffle Suite and offers a higher-level API compared to web3-core, making it easier to work with smart contracts. It integrates seamlessly with the Truffle framework for development and testing.
This is a sub-package of web3.js.
The core package contains core functions for web3.js packages.
Please read the documentation for more.
You can install the package either using NPM or using Yarn
npm install web3-core
yarn add web3-core
const core = require('web3-core');
const CoolLib = function CoolLib() {
// sets _requestmanager and adds basic functions
core.packageInit(this, arguments);
};
CoolLib.providers;
CoolLib.givenProvider;
CoolLib.setProvider();
CoolLib.BatchRequest();
CoolLib.extend();
...
All the TypeScript typings are placed in the types
folder.
FAQs
Web3 core tools for sub-packages. This is an internal package.
We found that web3-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.