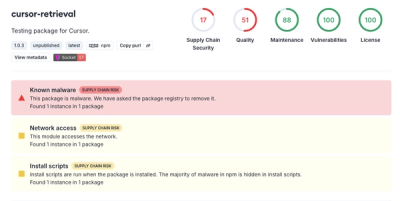
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
webcrypto-core
Advanced tools
Common layer to be used by crypto libraries based on WebCrypto API for input validation.
The webcrypto-core package is a JavaScript implementation of the Web Cryptography API specification. It provides a common interface for cryptographic operations such as hashing, signing, encryption, decryption, and key generation. This package is designed to be extensible, allowing for the addition of new cryptographic algorithms and their parameters.
Hashing
This feature allows for the hashing of data using various algorithms. The code sample demonstrates how to hash a message using the SHA-256 algorithm.
const { Sha256 } = require('webcrypto-core');
let sha256 = new Sha256();
sha256.digest({name: 'SHA-256'}, Buffer.from('message')).then(digest => {
console.log(digest);
});
Encryption/Decryption
This feature enables encryption and decryption of data. The code sample shows how to encrypt data using the AES-GCM algorithm.
const { AesGcm } = require('webcrypto-core');
let aesGcm = new AesGcm();
aesGcm.encrypt({name: 'AES-GCM', iv: Buffer.from(iv)}, key, Buffer.from(data)).then(encrypted => {
console.log(encrypted);
});
Signing/Verification
This feature is used for signing data and verifying signatures. The code sample illustrates how to sign data using the RSA-PSS algorithm.
const { RsaPss } = require('webcrypto-core');
let rsaPss = new RsaPss();
rsaPss.sign({name: 'RSA-PSS', saltLength: 32}, privateKey, Buffer.from(data)).then(signature => {
console.log(signature);
});
This package is an implementation of the Web Cryptography API based on OpenSSL. It provides similar functionalities to webcrypto-core but is specifically designed for Node.js environments and leverages OpenSSL for cryptographic operations.
A reimplementation of Node's 'crypto' module for the browser. While webcrypto-core focuses on providing a common interface for cryptographic operations, crypto-browserify aims to mimic Node.js's crypto module, making it easier for developers to write code that works both in Node.js and in the browser.
We have created a number of WebCrypto polyfills including: node-webcrypto-ossl, node-webcrypto-p11, and webcrypto-liner. webcrypto-core
was designed to be a common layer to be used by all of these libraries for input validation.
Unless you intend to create a WebCrypto polyfill this library is probably not useful to you.
npm install webcrypto-core
Current examples shows how you can implement your own WebCrypt interface
const core = require(".");
const crypto = require("crypto");
class Sha1Provider extends core.ProviderCrypto {
constructor() {
super();
this.name = "SHA-1";
this.usages = [];
}
async onDigest(algorithm, data) {
const hash = crypto.createHash("SHA1").update(Buffer.from(data)).digest();
return new Uint8Array(hash).buffer;
}
}
class SubtleCrypto extends core.SubtleCrypto {
constructor() {
super();
// Add SHA1 provider to SubtleCrypto
this.providers.set(new Sha1Provider());
}
}
class Crypto extends core.Crypto {
constructor() {
this.subtle = new SubtleCrypto();
}
getRandomValues(array) {
const buffer = Buffer.from(array.buffer);
crypto.randomFillSync(buffer);
return array;
}
}
const webcrypto = new Crypto();
webcrypto.subtle.digest("SHA-1", Buffer.from("TEST MESSAGE"))
.then((hash) => {
console.log(Buffer.from(hash).toString("hex")); // dbca505deb07e1612d944a69c0c851f79f3a4a60
})
.catch((err) => {
console.error(err);
});
FAQs
Common layer to be used by crypto libraries based on WebCrypto API for input validation.
The npm package webcrypto-core receives a total of 2,053,600 weekly downloads. As such, webcrypto-core popularity was classified as popular.
We found that webcrypto-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.