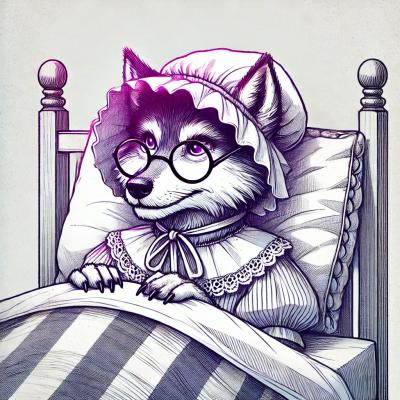
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
webrtc-adapter
Advanced tools
A shim to insulate apps from WebRTC spec changes and browser prefix differences
The webrtc-adapter npm package is a shim to insulate apps from spec changes and prefix differences in WebRTC APIs. It helps developers write code that works across different browsers by providing a consistent API.
Browser Compatibility
The webrtc-adapter package helps ensure that WebRTC code is compatible across different browsers by normalizing the API differences. This code sample demonstrates how to use the adapter to get browser details.
const adapter = require('webrtc-adapter');
console.log(adapter.browserDetails.browser); // Outputs the browser name
console.log(adapter.browserDetails.version); // Outputs the browser version
Unified API
The webrtc-adapter package provides a unified API for accessing media devices, making it easier to write cross-browser WebRTC applications. This code sample shows how to use the adapter to access the user's media devices.
const adapter = require('webrtc-adapter');
const getUserMedia = navigator.mediaDevices.getUserMedia || navigator.getUserMedia || navigator.webkitGetUserMedia || navigator.mozGetUserMedia;
getUserMedia({ video: true, audio: true })
.then(stream => {
console.log('Got MediaStream:', stream);
})
.catch(error => {
console.error('Error accessing media devices.', error);
});
Peer Connection
The webrtc-adapter package helps manage peer connections by normalizing the API across different browsers. This code sample demonstrates how to create a peer connection and handle ICE candidates.
const adapter = require('webrtc-adapter');
const pc = new RTCPeerConnection();
pc.onicecandidate = event => {
if (event.candidate) {
console.log('New ICE candidate:', event.candidate);
}
};
pc.createOffer()
.then(offer => pc.setLocalDescription(offer))
.then(() => console.log('Offer created and set as local description'))
.catch(error => console.error('Error creating offer:', error));
The simple-peer package is a lightweight wrapper around the WebRTC API that simplifies peer-to-peer data, video, and audio connections. Unlike webrtc-adapter, which focuses on normalizing the WebRTC API across browsers, simple-peer provides a higher-level abstraction for creating and managing peer connections.
The peerjs package is a library that simplifies WebRTC peer-to-peer data, video, and audio connections. It provides a server component for managing peer connections, which is different from webrtc-adapter's focus on API normalization. PeerJS is more focused on providing an easy-to-use API for creating peer connections.
The rtcpeerconnection package is a simple wrapper around the RTCPeerConnection API, providing a more straightforward interface for creating and managing WebRTC connections. Unlike webrtc-adapter, which aims to normalize the WebRTC API across different browsers, rtcpeerconnection focuses on simplifying the use of the RTCPeerConnection API.
adapter.js is a shim to insulate apps from spec changes and prefix differences. In fact, the standards and protocols used for WebRTC implementations are highly stable, and there are only a few prefixed names. For full interop information, see webrtc.org/web-apis/interop.
This repository used to be part of the WebRTC organisation on github but moved. We aim to keep the old repository updated with new releases.
npm install webrtc-adapter
bower install webrtc-adapter
Just import adapter:
import adapter from 'webrtc-adapter';
No further action is required.
Copy to desired location in your src tree or use a minify/vulcanize tool (node_modules is usually not published with the code). See webrtc/samples repo as an example on how you can do this.
In the gh-pages branch prebuilt ready to use files can be downloaded/linked directly. Latest version can be found at https://webrtc.github.io/adapter/adapter-latest.js. Specific versions can be found at https://webrtc.github.io/adapter/adapter-N.N.N.js, e.g. https://webrtc.github.io/adapter/adapter-1.0.2.js.
You will find adapter.js
in bower_components/webrtc-adapter/
.
In node_modules/webrtc-adapter/out/ folder you will find 4 files:
adapter.js
- includes all the shims and is visible in the browser under the global adapter
object (window.adapter).adapter_no_edge.js
- same as above but does not include the Microsoft Edge (ORTC) shim.adapter_no_edge_no_global.js
- same as above but is not exposed/visible in the browser (you cannot call/interact with the shims in the browser).adapter_no_global.js
- same as adapter.js
but is not exposed/visible in the browser (you cannot call/interact with the shims in the browser).Include the file that suits your need in your project.
Head over to test/README.md and get started developing.
patch
, minor
or major
in place of <version>
. Run npm version <version> -m 'bump to %s'
and type in your password lots of times (setting up credential caching is probably a good idea).git pull
npm publish
(you need access to the webrtc-adapter npmjs package). For big changes, consider using a tag version such as next
and then change the dist-tag after testing.Note: Currently only tested on Linux, not sure about Mac but will definitely not work on Windows.
In some cases it may be necessary to do a patch version while there are significant changes changes on the master branch. To make a patch release,
git checkout tags/vMajor.minor.patch
.git cherry-pick some-commit-hash
.npm version patch
. This will create a new patch version and publish it on github.origin/bumpVersion
branch and publish the new version using npm publish
.FAQs
A shim to insulate apps from WebRTC spec changes and browser prefix differences
The npm package webrtc-adapter receives a total of 260,818 weekly downloads. As such, webrtc-adapter popularity was classified as popular.
We found that webrtc-adapter demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.