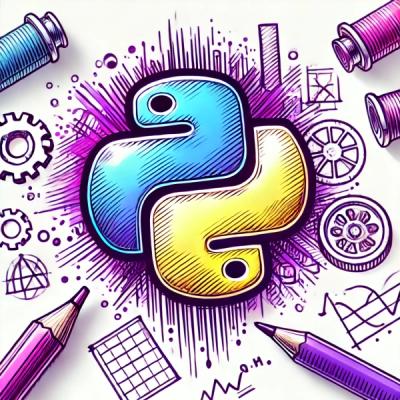
Security News
PyPI Slashes Malware Response Time: 90% of Issues Resolved in Under 24 Hours
PyPI has drastically improved its malware response times, resolving 90% of issues in under 24 hours and removing 900 projects since March 2024.
when
Advanced tools
Package description
The 'when' npm package is a robust library for working with asynchronous programming in JavaScript, particularly using promises. It provides utilities for creating, managing, and composing promises, making it easier to handle asynchronous operations and their potential complexities.
Creating Promises
This feature allows the creation of new promises. The code sample demonstrates how to create a simple promise that resolves with 'Hello, World!' after 1 second.
const when = require('when');
const promise = when.promise(function(resolve, reject) {
setTimeout(() => resolve('Hello, World!'), 1000);
});
promise.then(response => console.log(response));
Chaining Promises
This feature demonstrates chaining multiple promises. It shows how to perform a series of tasks sequentially, where each task starts only after the previous one has completed.
const when = require('when');
const cleanRoom = () => when.promise(resolve => resolve('Room cleaned'));
const removeTrash = () => when.promise(resolve => resolve('Trash removed'));
const winIcecream = () => when.promise(resolve => resolve('Won ice cream'));
cleanRoom()
.then(result => {
console.log(result);
return removeTrash();
})
.then(result => {
console.log(result);
return winIcecream();
})
.then(result => console.log(result));
Handling Errors
This feature involves error handling in promises. The code sample shows how to catch and handle errors that occur during the execution of promises.
const when = require('when');
const failTask = () => when.promise((resolve, reject) => reject('Failed task'));
failTask()
.then(result => console.log('Success:', result))
.catch(error => console.log('Error:', error));
Bluebird is a full-featured promise library with a focus on innovative features and performance. It is similar to 'when' but often cited for its superior performance and additional features like cancellation, progress tracking, and more detailed stack traces.
Q is one of the earliest promise libraries that influenced many others. It offers a similar API to 'when' but is generally considered to be less performant in modern applications. It provides a straightforward approach to handling asynchronous operations with promises.
The 'promise' package provides a minimalist implementation similar to the ES6 Promise specification. It is more lightweight compared to 'when' but lacks some of the more advanced features and utilities provided by 'when'.
Changelog
2.7.1
Readme
When.js is cujoJS's lightweight Promises/A+ and when()
implementation that powers the async core of wire.js, cujoJS's IOC Container. It features:
It passes the Promises/A+ Test Suite, is very fast and compact, and has no external dependencies.
Availble as when
through bower and yeoman, or just clone the repo and load when.js
from the root. When.js is AMD-compatible out of the box, so no need for shims.
npm install when
More help & other environments »
Promises can be used to help manage complex and/or nested callback flows in a simple manner. To get a better handle on how promise flows look and how they can be helpful, there are a couple examples below (using commonjs).
This first example will print "hello world!!!!"
if all went well, or "drat!"
if there was a problem. It also uses rest to make an ajax request to a (fictional) external service.
var rest = require('rest');
fetchRemoteGreeting()
.then(addExclamation)
.catch(handleError)
.done(function(greeting) {
console.log(greeting);
});
function fetchRemoteGreeting() {
// returns a when.js promise for 'hello world'
return rest('http://example.com/greeting');
}
function addExclamation(greeting) {
return greeting + '!!!!'
}
function handleError(e) {
return 'drat!';
}
The second example shows off the power that comes with when's promise logic. Here, we get an array of numbers from a remote source and reduce them. The example will print 150
if all went well, and if there was a problem will print a full stack trace.
var when = require('when');
var rest = require('rest');
when.reduce(when.map(getRemoteNumberList(), times10), sum)
.done(function(result) {
console.log(result);
});
function getRemoteNumberList() {
// Get a remote array [1, 2, 3, 4, 5]
return rest('http://example.com/numbers').then(JSON.parse);
}
function sum(x, y) { return x + y; }
function times10(x) {return x * 10; }
Licensed under MIT. See the license here »
Please see the contributing guide for more information on running tests, opening issues, and contributing code to the project.
Much of this code was inspired by the async innards of wire.js, and has been influenced by the great work in Q, Dojo's Deferred, and uber.js.
FAQs
A lightweight Promises/A+ and when() implementation, plus other async goodies.
The npm package when receives a total of 1,041,218 weekly downloads. As such, when popularity was classified as popular.
We found that when demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI has drastically improved its malware response times, resolving 90% of issues in under 24 hours and removing 900 projects since March 2024.
Security News
Research
The Socket Research team breaks down an obfuscated script designed to facilitate unauthorized file uploads to multiple external services.
Security News
Node.js has automated its security release process, doubling the number of releases, and is re-evaluating unsupported experimental features with the Next 10 group to enhance security.