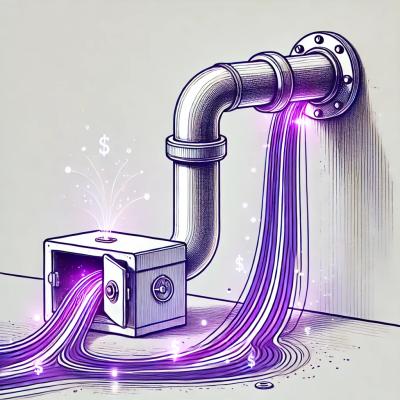
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
wifix-scan-coreplugin
Advanced tools
A wifix plugin to use all the wifix core libraries in a simple bundle
The Wifix Scan CorePlugin is a Cordova plugin that provides essential functionality for scanning Wi-Fi networks.
To install the Wifix Scan CorePlugin in your Cordova project, use the following command:
cordova plugin add wifix-scan-coreplugin
you can find a demo ionic cordova app using this library here:
Sample App - wifix-library-ionic-cordova
No special configurations needs to be done, just ensure that these permissions are granted in AndroidManifest.xml
:
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.WRITE_SETTINGS" tools:ignore="ProtectedPermissions" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
<uses-feature android:name="android.hardware.location.gps" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.CHANGE_NETWORK_STATE" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.CHANGE_WIFI_STATE" />
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
In your xcode project you need to:
You will need to add these entitlements so that the current access for wifi information is allowed, otherwise it will fail to fetch:
You can add these entitlements / capabilities directly to your xcode project on Signing & capabilities or by adding these entries to your config.xml
cordova project:
<config-file parent="com.apple.developer.networking.HotspotConfiguration"
target="*/Entitlements-Debug.plist">
<true />
</config-file>
<config-file parent="com.apple.developer.networking.HotspotConfiguration"
target="*/Entitlements-Release.plist">
<true />
</config-file>
<config-file parent="com.apple.developer.networking.networkextension"
target="*/Entitlements-Debug.plist">
<array />
</config-file>
<config-file parent="com.apple.developer.networking.networkextension"
target="*/Entitlements-Release.plist">
<array />
</config-file>
<config-file parent="com.apple.developer.networking.wifi-info"
target="*/Entitlements-Debug.plist">
<true />
</config-file>
<config-file parent="com.apple.developer.networking.wifi-info"
target="*/Entitlements-Release.plist">
<true />
</config-file>
Currently, due to Apple restrictions on Wifi Access Information and ICMP restrictions, the following features ARE NOT available in iOS:
This issue is related to the FastSocket deployment target being set to 8.0. You can resolve this by doing
pod install
In the root folder of your cordova/ ionic project.
// Create an instance of the Plugin
const wifix = new window.cordova.plugins.WifixScan();
wifix.doQuickScan(
(supportManager) => {
console.log("===> The scan was succesful");
console.log(
`Scan details and support provided via supportManager: ${supportManager}`
);
let testResult = await this.supportManager.runWifiSignalTest();
console.log(`Result from testing wifi signal: ${testResult}`)
},
(error) => {
console.log("XXX> error from ");
console.log(error);
},
"1234567890",
"myapikey"
);
The WifixScan
class encapsulates the public API for the Wifix plugin. It is responsible for handling scan requests and executing the required workflow for them.
constructor(baseURL: string = WifixConstants.DEFAULT_ISP_URL)
WifixScan
class.baseURL
(optional): The base URL for scan HTTP requests. Defaults to the default ISP URL specified in WifixConstants
.baseURL: string
WifixConstants.DEFAULT_ISP_URL
doFullScan
MethodThe doFullScan
method is a crucial function within the WifixScan
class, designed for initiating a full scan workflow in your Wifix npm plugin library. This method performs various checks, gathers information, and provides callbacks for handling success and errors.
/**
* Initiates a full scan.
*
* @param {(supportManager: ScanSupportManager) => void} successCallback - A callback function that receives an instance of the support manager for further scan testing.
* @param {(error: Error) => void} errorCallback - A callback function to handle errors during the scan process.
* @param {string} userContract - The user's contract information.
* @param {string} apiKey - The API key for using the Wifix plugin (optional, defaults to an empty string).
*/
doFullScan(successCallback, errorCallback, userContract, apiKey = '') {}
successCallback
(Function): A callback function that receives an instance of the ScanSupportManager
when the scan is succesful. This instance is crucial for further scan testing and exploration.
errorCallback
(Function): A callback function that receives an Error
object in case any step of the scan process encounters an error.
userContract
(String): The user's contract information required for the scan.
apiKey
(String, NOT OPTIONAL): The API key used for the Wifix plugin. Should be provided, otherwise an Invalid Key Error will be thrown instead.
doQuickScan
MethodThe doQuickScan
method initiates a Support (Quick) scan intended to provide assistance to the customer. This scan performs tests and provides a ScanSupportManager
instance through the success callback for further scan testing.
/**
* Initiates a Support (Quick) scan. This scan is intended to provide help to the customer by making tests with the `ScanSupportManager` instance provided on the callback.
*
* @param {(supportManager: ScanSupportManager) => void} successCallback - A callback function that has an instance of the support manager that can be used for further scan testing.
* @param {(error: Error) => void} errorCallback - A callback function to handle an error if any step of the scan process fails.
* @param {string} userContract - The user's contract information.
* @param {string} apiKey - The API key to use for this plugin.
*/
doQuickScan(successCallback, errorCallback, userContract, apiKey) {}
successCallback
(Function): A callback function that receives an instance of the ScanSupportManager
when the scan is succesful. This instance is crucial for further scan testing and exploration.
errorCallback
(Function): A callback function that receives an Error
object in case any step of the scan process encounters an error.
userContract
(String): The user's contract information required for the scan.
apiKey
(String, NOT OPTIONAL): The API key used for the Wifix plugin. Should be provided, otherwise an Invalid Key Error will be thrown instead.
ScanSupportManager
ClassThe ScanSupportManager
class is responsible for handling various scan support features such as tests, capturing pictures, opening channels, and initiating video conferences. This instance is returned on a succesful scan in the callback function for both doFullScan
or doQuickScan
method.
Each method of this Scan Support Manager, except for those that perform other things like getCurrentScanData
, takeScanPicture
and startRemoteAssistance
, returns a result object ScanSupportTestResult
providing information about the successfulness of the test.
The definition of ScanSupportTestResult
is the following:
{
sucess: boolean, //Tells if the test or method executed was succesful or not
message: string, // A message with information about the current test
error: string, // If present, indicates the error ocurred during the test / method
}
getCurrentScanData
MethodGets the current scan info by returning an object with the following structure:
/**
* returns the data for the current scan like scanCode, host of the current ISP api, wifiGateway and more.
* @returns {ISPApiData} - the data of the current scan
*/
getCurrentScanData () {
runWifiSignalTest
MethodRuns the WiFi signal test and updates the remote scan information.
/**
* Runs the WiFi signal test and updates the remote scan information.
* @returns {ScanSupportTestResult} - The result of the test.
*/
async runWifiSignalTest() {
return { success: true, message: "Wifi signal data updated successfully", error: null };
}
runCurrentWifiAndNearestWifiNetworksTest
MethodRuns the current WiFi info and nearest WiFi networks test and updates the remote scan information.
/**
* Runs the current WiFi info and nearest WiFi networks test and updates the remote scan information.
* @returns {ScanSupportTestResult} - The result of the test.
*/
async runCurrentWifiAndNearestWifiNetworksTest() {
return { success: true, message: "Wifi and nearest wifis data updated successfully", error: null };
}
runCurrentNetworkInfoAndDevicesConnectedTest
MethodRuns the current network info and connected devices test and updates the remote scan information.
/**
* Runs the current network info and connected devices test and updates the remote scan information.
* @returns {ScanSupportTestResult} - The result of the test.
*/
async runCurrentNetworkInfoAndDevicesConnectedTest() {
return { success: true, message: "Current network and connected devices data updated successfully", error: null };
}
runSpeedTest
MethodRuns a speed test on the current WiFi network and updates the remote scan information.
/**
* Runs a speed test on the current WiFi network and updates the remote scan information.
* @returns {ScanSupportTestResult} - The result of the test.
*/
async runSpeedTest() {
return { success: true, message: "Speed Test data updated successfully", error: null };
}
takeAndUploadPicture
MethodOpen the device camera to take a picture and send it to the server to update the proper scan data.
/**
* takes a picture from camera and uploads it to the proper scan data
* @returns {ScanSupportTestResult} - The result of taking the picture
*/
async takeAndUploadPicture () {}
startRemoteAssistance
MethodThis method makes a request to start a remote assistance, since this is a asynchronous request that can take long time. You will need to provide callbacks for each scenario of the remote assistance response:
/**
*
* @param {(result: RemoteAssistanceRequestResult) => void} onSuccess - callback when remote assitance request was succesful
* @param {(error: string) => void} onError - callback when the remote assistance request was failed
* @param {() => void | undefined} onClosed - optional callback when the remote assistance was remotely closed.
* @returns
*/
startRemoteAssistance(onSuccess, onError, (onClosed = undefined));
object
with the following properties is passed:
string
providing information about the error is passedcloseRemoteAssistance
callback is provided, so that you can close this communication. Not closing it could lead to unexpected behavior and errors.FAQs
A wifix plugin to use all the wifix core libraries in a simple bundle
The npm package wifix-scan-coreplugin receives a total of 0 weekly downloads. As such, wifix-scan-coreplugin popularity was classified as not popular.
We found that wifix-scan-coreplugin demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.