wix-style-processor
An alternative Wix Styles TPA processor.
This package provides a parser / transformer that scans your inlined CSS, and replaces its dynamic style declarations to the values defined by the user's website / template.
Installation
$ npm i -S wix-style-processor
Usage
CSS
.my-selector {
--my-font: "font(Body-M)";
--my-font2: "font({theme: 'Body-M', size: '10px', lineHeight: '2em', weight: 'bold', style:'italic'})"
--default-width: "number(42)";
font: "font(--my-font)";
width: calc(100% - "number(--default-width)");
color: "color(color-8)";
background-color: "join(opacity(color-1, 0.5), opacity(color-8, 0.5))";
color: "opacity(color-8, 0.3)";
color: "withoutOpacity(opacity(color-8, 0.3))";
color: "darken(color-8, 0.3)";
font: "font(--my-font2)";
border-width: "unit(--var-from-settings, px)";
}
Module initialization
import styleProcessor from 'wix-style-processor';
$(document).ready(() => {
styleProcessor.init().then(() => {
})
});
Plugin support
You can customize and extend the module's behavior with the use of plugins.
Plugins are invoked during the processing phase of the CSS declarations, and they let you override the built-in transformations (such as opacity or join), or add transformations of your own.
There are 2 kinds of plugins, which will be detailed below.
1. CSS Custom functions plugins
These plugins define functions that transform the value-side of the CSS declaration.
Usage
Plugin definition (JS):
import styleProcessor from 'wix-style-processor';
styleProcessor.plugins.addCssFunction(
'increment',
(param1, param2 ,..., siteParams?) => parseInt(params[0]) + 1
);
...
CSS definition:
.foo {
--baz: 1;
bar: "increment(number(--baz))"px;
}
The CSS above will be replaced to:
.foo {
--baz: 1;
bar: 2px;
}
2. Declaration Replacer plugins
These plugins allow you to replace the entire key / value of the CSS declaration.
Since they're invoked upon each and every declaration, there's no need to name them.
Example
Plugin definition (JS):
import styleProcessor from 'wix-style-processor';
styleProcessor.plugins.addDeclarationReplacer((key, value, siteParams) => ({
key: 'ZzZ-' + key + '-ZzZ',
value: '#-' + value + '-#'
}));
CSS definition:
.foo {
bar: 4;
}
The CSS above will be replaced to:
.foo {
ZzZ-bar-ZzZ: #-4-#;
}
It is a DeclarationReplacer plugin
that allows you to change dynamically LTR/RTL replacements in your CSS, you can use this plugin.
Enhanced Editor mode
This mode take leverage of native css vars to speed up the rendering of editor changes.
This feature will be enabled only on browsers that supports it and in Editor / Preview
mode.
Benchmarks
Without css-vars usage: (the first line is a timing of first render)
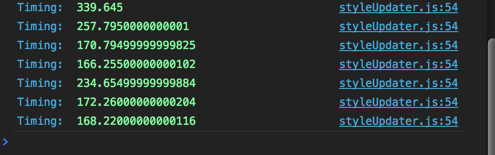
With css-vars usage: (the first line is a timing of first render)
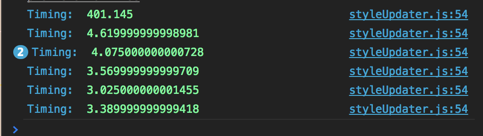
Demo:
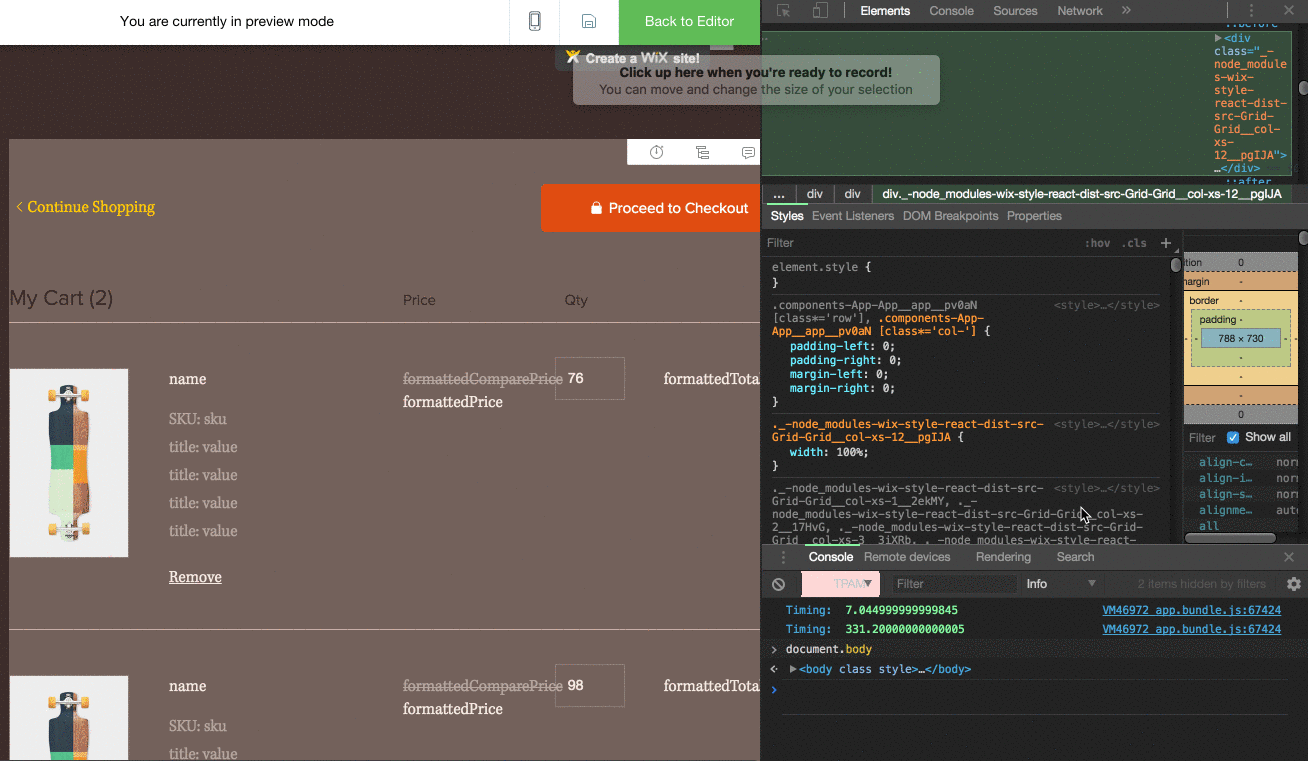
Important
This module only parses inline CSS.
It won't process any wix style params from an external (linked) CSS file.
The recommended approach for CSS inlining is by automating it in your build step - e.g. by using Webpack's style-loader.