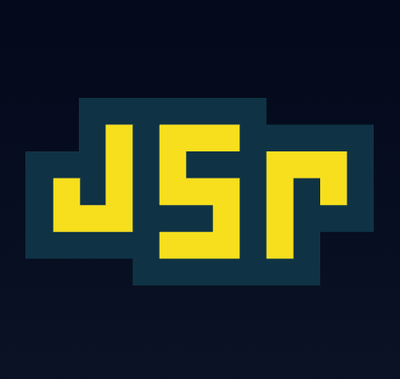
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
#wodge A collection of useful functions.
###w.symbol
some cross platform symbols (tick
and cross
)
###w.extend(object) Merge a list of objects, left to right, into one.
Object
a sequence of Object instances to be extended####Examples
> w.extend({}, { one: 1, three: 3 }, { one: "one", two: 2 }, { four: 4 });
{ one: 'one',
three: 3,
two: 2,
four: 4 }
###w.clone(input) Clones an object or array
Object | Array
the input to cloneReturns: Object,Array
####Examples
> date = new Date()
Fri May 09 2014 13:54:34 GMT+0200 (CEST)
> w.clone(date)
{} // a Date instance doensn't own any properties
> date.clive = "hater"
'hater'
> w.clone(date)
{ clive: 'hater' }
> array = [1,2,3]
[ 1, 2, 3 ]
> newArray = w.clone(array)
[ 1, 2, 3 ]
> array === newArray
false
###w.omit(object, toOmit) Returns a clone of the input object, minus the specified properties
Object
the object to cloneArray.<string>
an array of property names to omit from the cloneReturns: Object
####Examples
> w.omit({ one: 1, two: 2, three: 3, four: 4 }, [ "two", "four" ]);
{ one: 1, three: 3 }
###w.escapeRegExp() escape special regular expression characters
####Examples
> w.escapeRegExp("(.*)");
'\\(\\.\\*\\)'
###w.pluck(arrayOfObjects, the) Plucks the value of the specified property from each object in the input array
Array.<Object>
the input array of objectsstring
property to pluckReturns: Array
####Examples
> var data = [
... {one: 1, two: 2},
... {two: "two"},
... {one: "one", two: "zwei"},
... ];
undefined
> w.pluck(data, "one");
[ 1, 'one' ]
> w.pluck(data, "two");
[ 2, 'two', 'zwei' ]
> w.pluck(data, "one", "two");
[ 1, 'two', 'one' ]
###w.isNumber() Returns true if input is a number
####Examples
> w.isNumber(0)
true
> w.isNumber(1)
true
> w.isNumber(1.1)
true
> w.isNumber(0xff)
true
> w.isNumber(0644)
true
> w.isNumber(6.2e5)
true
> w.isNumber(a)
false
> w.isNumber(NaN)
false
> w.isNumber(Infinity)
false
###w.isPlainObject(input)
Returns true if input type is object
and not an Array
*
the input to testReturns: boolean
####Examples
> w.isPlainObject(new Date())
true
> w.isPlainObject({ clive: "hater" })
true
> w.isPlainObject([ 0, 1 ])
false
###w.arrayify(input) Takes input and guarantees an array back. Result can be one of three things:
puts a single scalar in an array
converts array-like object (e.g. arguments
) to a real array
converts null or undefined to an empty array
input *
the input value to convert to an array
Returns: Array
####Examples
> w.arrayify(null)
[]
> w.arrayify(0)
[ 0 ]
> w.arrayify([ 1, 2 ])
[ 1, 2 ]
> function f(){ return w.arrayify(arguments); }
undefined
> f(1,2,3)
[ 1, 2, 3 ]
###w.every(object, iterator) Returns true if the supplied iterator function returns true for every property in the object
Object
the object to inspectfunction
the iterator function to run against each key/value pair, the args are (value, key)
.Returns: Boolean
####Examples
> function aboveTen(input){ return input > 10; }
undefined
> w.every({ eggs: 12, carrots: 30, peas: 100 }, aboveTen)
true
> w.every({ eggs: 6, carrots: 30, peas: 100 }, aboveTen)
false
###w.each(object, callback) Runs the iterator function against every key/value pair in the input object
Object
the object to iteratefunction
the iterator function to run against each key/value pair, the args are (value, key)
.####Examples
> var total = 0;
undefined
> function addToTotal(n){ total += n; }
undefined
> w.each({ eggs: 3, celery: 2, carrots: 1 }, addToTotal)
undefined
> total
6
###w.bytesToSize(bytes, [precision]) Convert bytes to human-readable size
number
the bytes value to convertnumber
number of decimal placesReturns: string
####Examples
> w.bytesToSize(10000)
'10 KB'
> w.bytesToSize(10000, 1)
'9.8 KB'
> w.bytesToSize(10000, 2)
'9.77 KB'
> w.bytesToSize(10000, 3)
'9.766 KB'
###w.getHomeDir() Cross-platform home directory retriever
####Examples
> w.getHomeDir()
'/Users/Lloyd'
###w.fill(fillWith, len) Create a new string filled with the supplied character
string
the fill characternumber
the length of the output stringReturns: string
####Examples
> w.fill("a", 10)
'aaaaaaaaaa'
> w.fill("ab", 10)
'aaaaaaaaaa'
###w.padRight(input, width, [padWith]) Add padding to the right of a string
string
the string to padnumber
the desired final widthstring
the padding characterReturns: string
####Examples
> w.padRight("clive", 1)
'clive'
> w.padRight("clive", 1, "-")
'clive'
> w.padRight("clive", 10, "-")
'clive-----'
###w.exists(array, value) returns true if a value, or nested object value exists in an array
Array
the array to search*
the value to search forReturns: boolean
####Examples
> w.exists([ 1, 2, 3 ], 2)
true
> w.exists([ { result: false }, { result: false } ], { result: true })
false
> w.exists([ { result: true }, { result: false } ], { result: true })
true
> w.exists([ { result: true }, { result: true } ], { result: true })
true
###w.without(array, toRemove) Returns the input array, minus the specied values
Array
the input array*
a single, or array of values to omitReturns: Array
####Examples
> w.without([ 1, 2, 3 ], 2)
[ 1, 3 ]
> w.without([ 1, 2, 3 ], [ 2, 3 ])
[ 1 ]
###w.first(objectArray, prop, val)
Returns the first object in the input array with property
set to value
.
Array.<Object>
input array of objectsstring
property to inspect*
desired valueReturns: Object,undefined
####Examples
> w.first([{ product: "egg", stock: true }, { product: "chicken", stock: true }], "stock", true)
{ product: 'egg', stock: true }
> w.first([{ product: "egg", stock: true }, { product: "chicken", stock: true }], "stock", false)
undefined
###w.commonDir(files) commonDir returns the directory common to each path in the list
Array
An array of file paths to inspectReturns: string- A single path ending with the path separator, e.g. "/user/some/folder/"
####Examples
> files = fs.readdirSync(".").map(function(file){ return path.resolve(file); })
[ '/Users/Lloyd/Documents/75lb/wodge/.DS_Store',
'/Users/Lloyd/Documents/75lb/wodge/.git',
'/Users/Lloyd/Documents/75lb/wodge/.gitignore',
'/Users/Lloyd/Documents/75lb/wodge/.jshintrc',
'/Users/Lloyd/Documents/75lb/wodge/README.md',
'/Users/Lloyd/Documents/75lb/wodge/lib',
'/Users/Lloyd/Documents/75lb/wodge/node_modules',
'/Users/Lloyd/Documents/75lb/wodge/package.json',
'/Users/Lloyd/Documents/75lb/wodge/test' ]
> w.commonDir(files)
'/Users/Lloyd/Documents/75lb/wodge/'
###w.union() merge two arrays into a single array of unique values
####Examples
> var array1 = [ 1, 2 ], array2 = [ 2, 3 ];
undefined
> w.union(array1, array2)
[ 1, 2, 3 ]
> var array1 = [ { id: 1 }, { id: 2 } ], array2 = [ { id: 2 }, { id: 3 } ];
undefined
> w.union(array1, array2)
[ { id: 1 }, { id: 2 }, { id: 3 } ]
> var array2 = [ { id: 2, blah: true }, { id: 3 } ]
undefined
> w.union(array1, array2)
[ { id: 1 },
{ id: 2 },
{ id: 2, blah: true },
{ id: 3 } ]
> w.union(array1, array2, "id")
[ { id: 1 }, { id: 2 }, { id: 3 } ]
###w.commonSequence(a, b) Returns the initial elements which both input arrays have in common
Array
first array to compareArray
second array to compareReturns: Array
####Examples
> w.commonSequence([1,2,3], [1,2,4])
[ 1, 2 ]
###w.escapeForJSON(input) strips special characters, making suitable for storage in a JS/JSON string
string
the inputReturns: string
####Examples
> w.escapeForJSON("hello\nthere")
'hello\\nthere'
FAQs
a wodge of functional dough
The npm package wodge receives a total of 3 weekly downloads. As such, wodge popularity was classified as not popular.
We found that wodge demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.