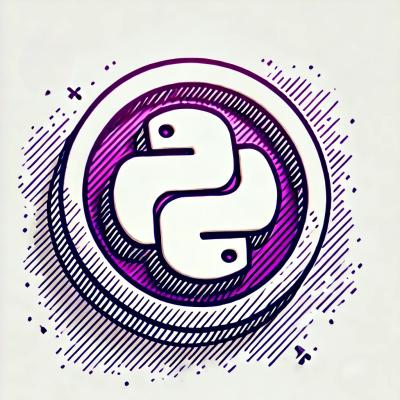
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
workbox-streams
Advanced tools
The workbox-streams package is part of the Workbox suite of libraries, which are designed to make it easier to build high-quality Progressive Web Apps (PWAs) by providing tools that leverage service workers. Specifically, workbox-streams allows you to efficiently combine multiple strategies for fetching resources, enabling you to use streams to dynamically construct responses within a service worker. This can be particularly useful for scenarios where you want to cache certain parts of a request or dynamically generate content.
Concatenating responses from multiple sources
This feature allows you to concatenate responses from multiple sources, such as cache or network, into a single response. This is useful for constructing a full page response from separate parts.
import {strategy, concatenate, concatenateToResponse} from 'workbox-streams';
self.addEventListener('fetch', (event) => {
event.respondWith((async () => {
const parts = [
caches.match('/header.html'),
fetch('/main-content.html'),
caches.match('/footer.html')
];
return concatenateToResponse(parts);
})());
});
Using streams to dynamically generate content
This feature demonstrates how you can use streams to dynamically generate content by combining static and dynamic parts of a response. This is particularly useful for injecting dynamic content into a static template.
import {strategy, concatenate, concatenateToResponse} from 'workbox-streams';
self.addEventListener('fetch', (event) => {
event.respondWith((async () => {
const parts = [
new Response('<html><body>'),
fetch('/dynamic-content'),
new Response('</body></html>')
];
return concatenateToResponse(parts);
})());
});
sw-toolbox is a predecessor to Workbox, offering similar functionalities for service worker management and response strategies. However, Workbox, including workbox-streams, provides a more modern and comprehensive approach with additional features and improvements.
sw-precache is another tool that predates Workbox and focuses on precaching resources to make them available offline. While it offers some similar capabilities in terms of caching, workbox-streams specifically allows for more dynamic content generation and manipulation using streams, which is not a focus of sw-precache.
This module's documentation can be found at https://developers.google.com/web/tools/workbox/reference-docs/latest/module-workbox-streams
FAQs
A library that makes it easier to work with Streams in the browser.
We found that workbox-streams demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.