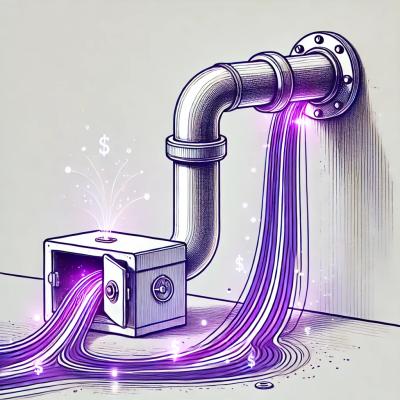
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
world-information
Advanced tools
world-information is a comprehensive npm package designed to provide detailed geographical information. It includes data on continents, countries, states, and cities, making it an essential tool for applications that require location-based data.
npm install world-information
Continent array
Country array
State array
getCountryByContinentName(continentName);
getStateByCountryName(countryName);
Cities
getCitiesByStateName(stateName);
const worldInformation = require('world-information');
// Get a list of continents
const continents = worldInformation.continentList;
console.log(continents);
// Output:
[
{ name: 'Africa', code: 'AF' },
{ name: 'Antarctica', code: 'AN' },
{ name: 'Asia', code: 'AS' },
{ name: 'Europe', code: 'EU' },
{ name: 'North America', code: 'NA' },
{ name: 'Oceania', code: 'OC' },
{ name: 'South America', code: 'SA' }
]
const worldInformation = require('world-information');
// Get a list of countries
const countries = worldInformation.countryList;
console.log(countries);
// Output (sample):
[
{ "name": "Afghanistan", "alpha-2": "AF", "alpha-3": "AFG", "numeric": "004", "continent": "Asia", "phoneCode": "+93" },
{ "name": "Albania", "alpha-2": "AL", "alpha-3": "ALB", "numeric": "008", "continent": "Europe", "phoneCode": "+355" },
...
{ "name": "Zimbabwe", "alpha-2": "ZW", "alpha-3": "ZWE", "numeric": "716", "continent": "Africa", "phoneCode": "+263" }
]
const {getCountryByContinentName} = require("world-information");
// Get a list of countries in asia continent
/*
Make sure the argument name is one of the below continent.
I have also configured the JSDoc Annotations, So you will get the suggestions too.
The argument value(continent name) is case insensitive.
'Africa' | 'Antarctica' | 'Asia' | 'Europe' | 'North America' | 'Oceania' | 'South America'
*/
const asianCountries = getCountryByContinentName("Asia");
console.log(asianCountries);
// Output (sample):
[
{ "name": "Afghanistan", "alpha-2": "AF", "alpha-3": "AFG", "numeric": "004", "continent": "Asia", "phoneCode": "+93" },
...
{ "name": "Yemen", "alpha-2": "YE", "alpha-3": "YEM", "numeric": "887", "continent": "Asia", "phoneCode": "+967" },
]
const {getStateByCountryName} = require("world-information");
/*
Make sure the argument name is one of the below country.
I have also configured the JSDoc Annotations, So you will get the suggestions too.
The argument value(country name) is case insensitive.
'Afghanistan' | 'Albania' | 'Algeria' | 'Andorra' | 'Angola' | 'Antigua and Barbuda' | 'Argentina' | 'Armenia' | 'Australia' | 'Austria' | 'Azerbaijan' | 'Bahamas' | 'Bahrain' | 'Bangladesh' | 'Barbados' | 'Belarus' | 'Belgium' | 'Belize' | 'Benin' | 'Bhutan' | 'Bolivia' | 'Bosnia and Herzegovina' | 'Botswana' | 'Brazil' | 'Brunei' | 'Bulgaria' | 'Burkina Faso' | 'Burundi' | 'Cabo Verde' | 'Cambodia' | 'Cameroon' | 'Canada' | 'Central African Republic' | 'Chad' | 'Chile' | 'China' | 'Colombia' | 'Comoros' | 'Congo' | 'Costa Rica' | 'Croatia' | 'Cuba' | 'Cyprus' | 'Czech Republic' | 'Denmark' | 'Djibouti' | 'Dominica' | 'Dominican Republic' | 'Ecuador' | 'Egypt' | 'El Salvador' | 'Equatorial Guinea' | 'Eritrea' | 'Estonia' | 'Eswatini' | 'Ethiopia' | 'Fiji' | 'Finland' | 'France' | 'Gabon' | 'Gambia' | 'Georgia' | 'Germany' | 'Ghana' | 'Greece' | 'Grenada' | 'Guatemala' | 'Guinea' | 'Guinea-Bissau' | 'Guyana' | 'Haiti' | 'Honduras' | 'Hungary' | 'Iceland' | 'India' | 'Indonesia' | 'Iran' | 'Iraq' | 'Ireland' | 'Israel' | 'Italy' | 'Jamaica' | 'Japan' | 'Jordan' | 'Kazakhstan' | 'Kenya' | 'Kiribati' | 'Korea, North' | 'Korea, South' | 'Kuwait' | 'Kyrgyzstan' | 'Laos' | 'Latvia' | 'Lebanon' | 'Lesotho' | 'Liberia' | 'Libya' | 'Liechtenstein' | 'Lithuania' | 'Luxembourg' | 'Madagascar' | 'Malawi' | 'Malaysia' | 'Maldives' | 'Mali' | 'Malta' | 'Marshall Islands' | 'Mauritania' | 'Mauritius' | 'Mexico' | 'Micronesia' | 'Moldova' | 'Monaco' | 'Mongolia' | 'Montenegro' | 'Morocco' | 'Mozambique' | 'Myanmar' | 'Namibia' | 'Nauru' | 'Nepal' | 'Netherlands' | 'New Zealand' | 'Nicaragua' | 'Niger' | 'Nigeria' | 'North Macedonia' | 'Norway' | 'Oman' | 'Pakistan' | 'Palau' | 'Panama' | 'Papua New Guinea' | 'Paraguay' | 'Peru' | 'Philippines' | 'Poland' | 'Portugal' | 'Qatar' | 'Romania' | 'Russia' | 'Rwanda' | 'Saint Kitts and Nevis' | 'Saint Lucia' | 'Saint Vincent and the Grenadines' | 'Samoa' | 'San Marino' | 'Sao Tome and Principe' | 'Saudi Arabia' | 'Senegal' | 'Serbia' | 'Seychelles' | 'Sierra Leone' | 'Singapore' | 'Slovakia' | 'Slovenia' | 'Solomon Islands' | 'Somalia' | 'South Africa' | 'South Sudan' | 'Spain' | 'Sri Lanka' | 'Sudan' | 'Suriname' | 'Sweden' | 'Switzerland' | 'Syria' | 'Taiwan' | 'Tajikistan' | 'Tanzania' | 'Thailand' | 'Timor-Leste' | 'Togo' | 'Tonga' | 'Trinidad and Tobago' | 'Tunisia' | 'Turkey' | 'Turkmenistan' | 'Tuvalu' | 'Uganda' | 'Ukraine' | 'United Arab Emirates' | 'United Kingdom' | 'United States' | 'Uruguay' | 'Uzbekistan' | 'Vanuatu' | 'Vatican City' | 'Venezuela' | 'Vietnam' | 'Yemen' | 'Zambia' | 'Zimbabwe'
*/
// Get a list of states in india
const indianStates = getStateByCountryName("India");
console.log(indianStates);
// Output (sample)
[
{name: 'Andhra Pradesh', code: 'IN-AP', country: 'India'},
{name: 'Arunachal Pradesh', code: 'IN-AR', country: 'India'},
...
{name: 'Puducherry', code: 'IN-PY', country: 'India'}
]
Contributions are welcome! Please open an issue or submit a pull request on GitHub to help improve this package. ♥♥♥
FAQs
world-information is a comprehensive npm package designed to provide detailed geographical information. It includes data on continents, countries, states, and cities, making it an essential tool for applications that require location-based data.
The npm package world-information receives a total of 0 weekly downloads. As such, world-information popularity was classified as not popular.
We found that world-information demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.