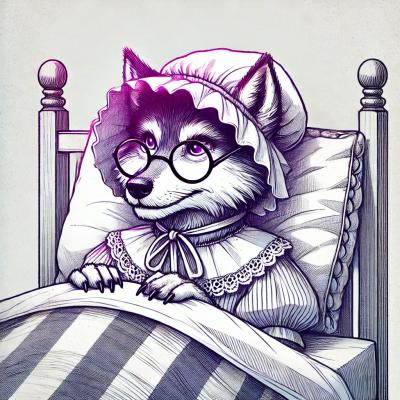
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The xhr npm package is a simple and lightweight library for making XMLHttpRequests in a browser environment. It provides a straightforward API for performing HTTP requests, handling responses, and managing errors.
Basic GET Request
This feature allows you to perform a basic GET request to a specified URL. The callback function handles the response or any errors that occur.
const xhr = require('xhr');
xhr({ url: 'https://api.example.com/data' }, function (err, resp, body) {
if (err) {
console.error(err);
} else {
console.log(body);
}
});
POST Request with Data
This feature allows you to perform a POST request with a JSON payload. The request includes headers to specify the content type, and the callback function handles the response or any errors.
const xhr = require('xhr');
xhr({
url: 'https://api.example.com/data',
method: 'POST',
body: JSON.stringify({ key: 'value' }),
headers: { 'Content-Type': 'application/json' }
}, function (err, resp, body) {
if (err) {
console.error(err);
} else {
console.log(body);
}
});
Handling Response Status Codes
This feature demonstrates how to handle different HTTP response status codes. The callback function checks the status code and logs the appropriate message.
const xhr = require('xhr');
xhr({ url: 'https://api.example.com/data' }, function (err, resp, body) {
if (err) {
console.error(err);
} else if (resp.statusCode === 200) {
console.log('Success:', body);
} else {
console.log('Error:', resp.statusCode);
}
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides a more powerful and flexible API compared to xhr, including support for interceptors, automatic JSON transformation, and request cancellation.
Fetch is a built-in web API for making HTTP requests in the browser. It is a modern alternative to XMLHttpRequest and provides a more powerful and flexible API. Unlike xhr, fetch is built into modern browsers and does not require an external library.
Superagent is a small, progressive client-side HTTP request library. It provides a more feature-rich API compared to xhr, including support for promises, request retries, and plugins.
A small xhr wrapper
var xhr = require("xhr")
xhr({
method: "GET",
data: someJSON,
uri: "/foo",
headers: {
"Content-Type": "application/json"
}
}, function (err, result) {
// this === xhr
})
var req = xhr(options, callback)
the returned object is either an XMLHttpRequest
instance
or an XDomainRequest
instance (if on IE8/IE9)
Your callback will be called once with either an Error
or a valid result
. The result will be either
xhr.response
, xhr.responseText
or
xhr.responseXML
depending on the request type.
Your callback will be called with an Error
if the
resulting status of the request is either 0
, 4xx
or 5xx
options.method
Specify the method the XMLHttpRequest
should be opened
with. Passed to xhr.open
options.data
Pass in data to be send across the XMLHttpRequest
.
Generally should be a string. But anything that's valid as
a parameter to xhr.send
should work
options.status
Set this to false
if you do not want this module to turn
a status code of 4xx, 5xx or 0 into an error.
options.uri
The uri to send a request too. Passed to
xhr.open
options.headers
An object of headers that should be set on the request. The
key, value pair is passed to xhr.setRequestHeader
FAQs
small xhr abstraction
The npm package xhr receives a total of 1,112,860 weekly downloads. As such, xhr popularity was classified as popular.
We found that xhr demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.