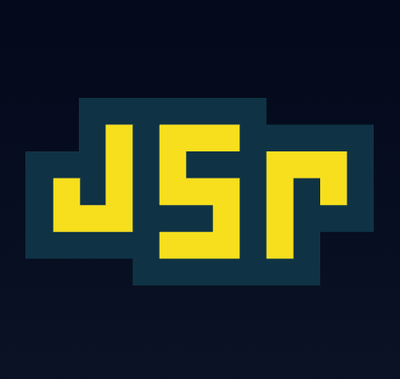
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The xhr2 npm package is a server-side implementation of the XMLHttpRequest API, which is commonly used in web browsers for making HTTP requests. This package allows Node.js applications to make HTTP requests using the familiar XMLHttpRequest interface.
Basic GET Request
This feature allows you to make a basic GET request to a specified URL. The code sample demonstrates how to open a connection, send the request, and handle the response.
const XMLHttpRequest = require('xhr2');
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://jsonplaceholder.typicode.com/posts/1', true);
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
console.log(xhr.responseText);
} else {
console.error('Request failed with status:', xhr.status);
}
};
xhr.send();
POST Request with Data
This feature allows you to make a POST request with data. The code sample demonstrates how to set the request method to POST, set the appropriate headers, and send JSON data in the request body.
const XMLHttpRequest = require('xhr2');
const xhr = new XMLHttpRequest();
xhr.open('POST', 'https://jsonplaceholder.typicode.com/posts', true);
xhr.setRequestHeader('Content-Type', 'application/json;charset=UTF-8');
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
console.log(xhr.responseText);
} else {
console.error('Request failed with status:', xhr.status);
}
};
xhr.send(JSON.stringify({ title: 'foo', body: 'bar', userId: 1 }));
Handling Errors
This feature allows you to handle errors that may occur during the request. The code sample demonstrates how to handle both HTTP status errors and network errors.
const XMLHttpRequest = require('xhr2');
const xhr = new XMLHttpRequest();
xhr.open('GET', 'https://jsonplaceholder.typicode.com/invalid-url', true);
xhr.onload = function () {
if (xhr.status >= 200 && xhr.status < 300) {
console.log(xhr.responseText);
} else {
console.error('Request failed with status:', xhr.status);
}
};
xhr.onerror = function () {
console.error('Network error occurred');
};
xhr.send();
Axios is a promise-based HTTP client for the browser and Node.js. It provides a more modern and flexible API compared to xhr2, including support for promises and async/await, which makes it easier to handle asynchronous operations.
Node-fetch is a lightweight module that brings the Fetch API to Node.js. It is similar to xhr2 in that it allows you to make HTTP requests, but it uses the modern Fetch API, which is more widely used in modern web development.
Request is a simplified HTTP client for Node.js. It provides a more user-friendly API compared to xhr2 and supports features like cookies, redirects, and multipart form data. However, it has been deprecated in favor of more modern alternatives like axios and node-fetch.
This is an npm package that implements the W3C XMLHttpRequest specification on top of the node.js APIs.
This library is tested against the following platforms.
Keep in mind that the versions above are not hard requirements.
The preferred installation method is to add the library to the dependencies
section in your package.json
.
{
"dependencies": {
"xhr2": "*"
}
}
Alternatively, npm
can be used to install the library directly.
npm install xhr2
Once the library is installed, require
-ing it returns the XMLHttpRequest
constructor.
var XMLHttpRequest = require('xhr2');
The other objects that are usually defined in an XHR environment are hanging
off of XMLHttpRequest
.
var XMLHttpRequestUpload = XMLHttpRequest.XMLHttpRequestUpload;
MDN (the Mozilla Developer Network) has a great intro to XMLHttpRequest.
This library's CoffeeDocs can be used as quick reference to the XMLHttpRequest specification parts that were implemented.
The following standard features are implemented.
http
and https
URI protocolssend()
accepts the following data types: String, ArrayBufferView,
ArrayBuffer (deprecated in the standard)responseType
values: text
, json
, arraybuffer
readystatechange
and download progress eventsoverrideMimeType()
abort()
timeout
The following node.js extensions are implemented.
send()
accepts a node.js BufferresponseType
to buffer
produces a node.js BuffernodejsSet
does XHR network configuration that is not exposed in browsers,
for security reasonsThe following standard features are not implemented.
file://
URIsdata:
URIsThe library aims to implement the W3C XMLHttpRequest specification, so the library's API will always be a (hopefully growing) subset of the API in the specification.
The following commands will get the source tree in a node-xhr2/
directory and
build the library.
git clone git://github.com/pwnall/node-xhr2.git
cd node-xhr2
npm install
npm pack
Installing CoffeeScript globally will let you type cake
instead of
node_modules/.bin/cake
npm install -g coffee-script
The library comes with unit tests that exercise the XMLHttpRequest API.
cake test
The tests themselves can be tested by running them in a browser environment, where a different XMLHttpRequest implementation is available. Both Google Chrome and Firefox deviate from the specification in small ways, so it's best to run the tests in both browsers and mentally compute an intersection of the failing tests.
cake webtest
BROWSER=firefox cake webtest
The library is Copyright (c) 2013 Victor Costan, and distributed under the MIT License.
FAQs
XMLHttpRequest emulation for node.js
The npm package xhr2 receives a total of 671,981 weekly downloads. As such, xhr2 popularity was classified as popular.
We found that xhr2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.