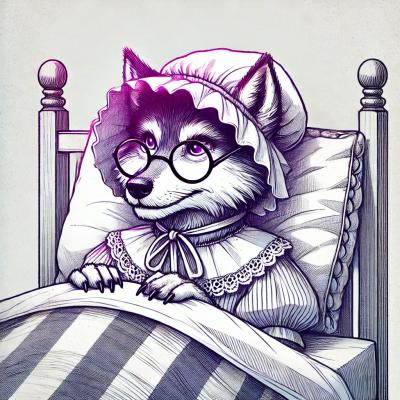
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
xml-encryption
Advanced tools
[](https://travis-ci.org/auth0/node-xml-encryption)
The xml-encryption npm package provides tools for encrypting and decrypting XML data. It supports various encryption algorithms and allows for the secure handling of XML documents.
Encrypting XML Data
This feature allows you to encrypt XML data using a specified encryption algorithm and keys. The code sample demonstrates how to encrypt an XML string using RSA and AES256-CBC.
const xmlenc = require('xml-encryption');
const xml = '<root><name>John Doe</name></root>';
const options = { rsa_pub: 'public_key.pem', pem: 'private_key.pem', encryptionAlgorithm: 'http://www.w3.org/2001/04/xmlenc#aes256-cbc' };
xmlenc.encrypt(xml, options, function(err, result) {
if (err) console.error(err);
console.log('Encrypted XML:', result);
});
Decrypting XML Data
This feature allows you to decrypt previously encrypted XML data using the appropriate private key. The code sample demonstrates how to decrypt an encrypted XML string.
const xmlenc = require('xml-encryption');
const encryptedXml = '...'; // Encrypted XML string
const options = { key: 'private_key.pem' };
xmlenc.decrypt(encryptedXml, options, function(err, result) {
if (err) console.error(err);
console.log('Decrypted XML:', result);
});
The xml2js package is used for parsing XML to JavaScript objects and vice versa. While it does not provide encryption functionalities, it is useful for handling XML data in JavaScript applications.
The xml-crypto package provides tools for XML digital signatures and encryption. It offers similar functionalities to xml-encryption but also includes support for XML signatures, making it a more comprehensive solution for XML security.
The node-forge package is a comprehensive library for cryptographic operations in JavaScript, including encryption, decryption, and key generation. While it is not specific to XML, it can be used to implement custom XML encryption and decryption solutions.
W3C XML Encryption implementation for node.js (http://www.w3.org/TR/xmlenc-core/)
Supports node >= 12
npm install xml-encryption
var xmlenc = require('xml-encryption');
var options = {
rsa_pub: fs.readFileSync(__dirname + '/your_rsa.pub'),
pem: fs.readFileSync(__dirname + '/your_public_cert.pem'),
encryptionAlgorithm: 'http://www.w3.org/2001/04/xmlenc#aes256-cbc',
keyEncryptionAlgorithm: 'http://www.w3.org/2001/04/xmlenc#rsa-oaep-mgf1p',
disallowEncryptionWithInsecureAlgorithm: true,
warnInsecureAlgorithm: true
};
xmlenc.encrypt('content to encrypt', options, function(err, result) {
console.log(result);
}
Result:
<xenc:EncryptedData Type="http://www.w3.org/2001/04/xmlenc#Element" xmlns:xenc="http://www.w3.org/2001/04/xmlenc#">
<xenc:EncryptionMethod Algorithm="http://www.w3.org/2001/04/xmlenc#aes-256-cbc" />
<KeyInfo xmlns="http://www.w3.org/2000/09/xmldsig#">
<e:EncryptedKey xmlns:e="http://www.w3.org/2001/04/xmlenc#">
<e:EncryptionMethod Algorithm="http://www.w3.org/2001/04/xmlenc#rsa-oaep-mgf1p">
<DigestMethod Algorithm="http://www.w3.org/2000/09/xmldsig#sha1" />
</e:EncryptionMethod>
<KeyInfo>
<X509Data><X509Certificate>MIIEDzCCAveg... base64 cert... q3uaLvlAUo=</X509Certificate></X509Data>
</KeyInfo>
<e:CipherData>
<e:CipherValue>sGH0hhzkjmLWYYY0gyQMampDM... encrypted symmetric key ...gewHMbtZafk1MHh9A==</e:CipherValue>
</e:CipherData>
</e:EncryptedKey>
</KeyInfo>
<xenc:CipherData>
<xenc:CipherValue>V3Vb1vDl055Lp92zvK..... encrypted content.... kNzP6xTu7/L9EMAeU</xenc:CipherValue>
</xenc:CipherData>
</xenc:EncryptedData>
var options = {
key: fs.readFileSync(__dirname + '/your_private_key.key'),
disallowDecryptionWithInsecureAlgorithm: true,
warnInsecureAlgorithm: true
};
xmlenc.decrypt('<xenc:EncryptedData ..... </xenc:EncryptedData>', options, function(err, result) {
console.log(result);
}
// result
decrypted content
Currently the library supports:
EncryptedKey to transport symmetric key using:
EncryptedData using:
Insecure Algorithms can be disabled via disallowEncryptionWithInsecureAlgorithm
/disallowDecryptionWithInsecureAlgorithm
flags when encrypting/decrypting. This flag is off by default in 0.x versions.
A warning will be piped to stderr
using console.warn() by default when the aforementioned algorithms are used. This can be disabled via the warnInsecureAlgorithm
flag.
If you have found a bug or if you have a feature request, please report them at this repository issues section. Please do not report security vulnerabilities on the public GitHub issue tracker. The Responsible Disclosure Program details the procedure for disclosing security issues.
This project is licensed under the MIT license. See the LICENSE file for more info.
Release notes may be found under github release page: https://github.com/auth0/node-xml-encryption/releases
FAQs
[](https://travis-ci.org/auth0/node-xml-encryption)
We found that xml-encryption demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 51 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.