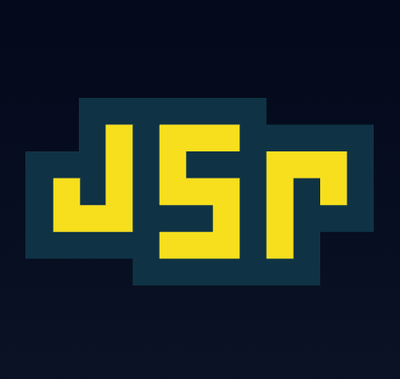
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The xmlcreate npm package is a lightweight library for creating XML documents in a programmatic way. It provides a simple API to build XML structures, making it easy to generate XML content dynamically.
Creating XML Documents
This feature allows you to create a new XML document with a specified version and encoding. You can then add elements to the document, including nested elements.
const { document } = require('xmlcreate');
const doc = document({ version: '1.0', encoding: 'UTF-8' });
const root = doc.element({ name: 'root' });
root.element({ name: 'child', text: 'Hello, World!' });
console.log(doc.toString());
Adding Attributes to Elements
This feature allows you to add attributes to XML elements. In this example, an 'id' attribute is added to the 'child' element.
const { document } = require('xmlcreate');
const doc = document({ version: '1.0', encoding: 'UTF-8' });
const root = doc.element({ name: 'root' });
const child = root.element({ name: 'child', text: 'Hello, World!' });
child.attribute({ name: 'id', value: '1' });
console.log(doc.toString());
Pretty Printing XML
This feature allows you to output the XML document in a pretty-printed format, making it more readable.
const { document } = require('xmlcreate');
const doc = document({ version: '1.0', encoding: 'UTF-8' });
const root = doc.element({ name: 'root' });
root.element({ name: 'child', text: 'Hello, World!' });
console.log(doc.toString({ pretty: true }));
xmlbuilder is another popular library for building XML documents in a programmatic way. It offers a more extensive API and additional features like DTD support and namespace handling. Compared to xmlcreate, xmlbuilder is more feature-rich but also slightly more complex to use.
xml2js is primarily used for converting XML to JavaScript objects and vice versa. While it can also create XML documents, its main strength lies in parsing XML. It is more versatile in terms of data manipulation but less focused on XML creation compared to xmlcreate.
fast-xml-parser is known for its high performance in parsing XML to JSON and vice versa. It also supports XML building, but its primary focus is on fast and efficient parsing. It is a good choice if performance is a critical factor.
xmlcreate is a Node.js module that can be used to build XML using a simple API.
xmlcreate allows you to use a series of chained function calls to build an XML tree.
Once the tree is built, it can be serialized to text. The formatting of the text is customizable.
xmlcreate can perform some basic validation to check that the resulting XML is well-formed.
The easiest way to install xmlcreate is using npm:
npm install xmlcreate
You can also build xmlcreate from source using npm and gulp:
git clone https://github.com/michaelkourlas/node-xmlcreate.git
npm install
./node_modules/.bin/gulp
The default
target will build the production variant of xmlcreate, run all
tests, and build the documentation.
You can build the production variant without running tests using the target
prod
. You can also build the development version using the target dev
.
The only difference between the two is that the development version includes
source maps.
The documentation for the current version is available here.
You can also build the documentation using gulp:
gulp docs
The following TypeScript example illustrates the basic usage of xmlcreate:
import {document} from "xmlcreate";
const tree = document();
tree
.decl({encoding: "UTF-8"})
.up()
.dtd({
name: "html",
pubId: "-//W3C//DTD XHTML 1.0 Strict//EN",
sysId: "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"
})
.up()
.element({name: "html"})
.attribute({name: "xmlns"})
.text({charData: "http://www.w3.org/1999/xhtml"})
.up()
.up()
.attribute({name: "xml:lang"})
.text({charData: "en"})
.up()
.up()
.element({name: "head"})
.element({name: "title"})
.charData({charData: "My page title"})
.up()
.up()
.up()
.element({name: "body"})
.element({name: "h1"})
.charData({charData: "Welcome!"})
.up()
.up()
.element({name: "p"})
.charData({charData: "This is some text on my website."})
.up()
.up()
.element({name: "div"})
.element({name: "img"})
.attribute({name: "src"})
.text({charData: "picture.png"})
.up()
.up()
.attribute({name: "alt"})
.text({charData: "picture"}).up().up().up().up().up();
console.log(tree.toString({doubleQuotes: true}));
This example produces the following XML:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en">
<head>
<title>My page title</title>
</head>
<body>
<h1>Welcome!</h1>
<p>This is some text on my website.</p>
</body>
</html>
A JavaScript version of this example can be found in the examples directory.
xmlcreate includes a set of tests to verify core functionality. You can run the tests using gulp:
gulp test
The test
target builds the production variant of xmlcreate before running
the tests. The test-prod
target does the same thing, while the test-dev
target builds the development variant first instead.
xmlcreate is licensed under the Apache License 2.0. Please see the LICENSE.md file for more information.
2.0.0
FAQs
Simple XML builder for Node.js
The npm package xmlcreate receives a total of 1,868,610 weekly downloads. As such, xmlcreate popularity was classified as popular.
We found that xmlcreate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.