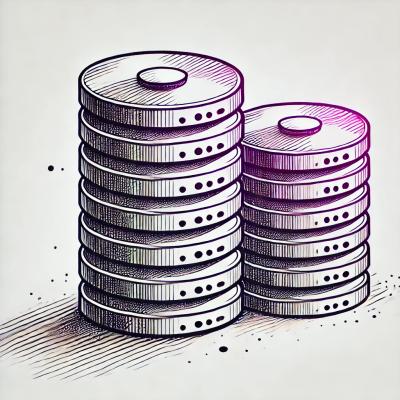
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
xterm is a terminal emulator library that can be used to create terminal interfaces in web applications. It provides a way to embed a terminal in a web page, allowing users to interact with a command-line interface directly from their browser.
Basic Terminal Initialization
This code initializes a basic terminal instance and attaches it to a DOM element with the ID 'terminal-container'.
const { Terminal } = require('xterm');
const terminal = new Terminal();
terminal.open(document.getElementById('terminal-container'));
Customizing Terminal Appearance
This code demonstrates how to customize the appearance of the terminal, including the number of columns and rows, as well as the theme colors for the background and foreground.
const { Terminal } = require('xterm');
const terminal = new Terminal({
cols: 80,
rows: 24,
theme: {
background: '#1e1e1e',
foreground: '#ffffff'
}
});
terminal.open(document.getElementById('terminal-container'));
Handling Terminal Input
This code sets up an event listener to handle user input in the terminal. The input data is logged to the console.
const { Terminal } = require('xterm');
const terminal = new Terminal();
terminal.open(document.getElementById('terminal-container'));
terminal.onData(data => {
console.log('User input:', data);
});
Writing Data to Terminal
This code demonstrates how to write data to the terminal. The text 'Hello, World!' is written to the terminal, followed by a carriage return and newline.
const { Terminal } = require('xterm');
const terminal = new Terminal();
terminal.open(document.getElementById('terminal-container'));
terminal.write('Hello, World!\r\n');
Blessed is a curses-like library for creating terminal user interfaces in Node.js. It provides a higher-level abstraction for building complex terminal applications, including support for widgets like buttons, text boxes, and more. Compared to xterm, blessed is more focused on creating full-fledged terminal applications rather than embedding a terminal emulator in a web page.
Terminal-kit is a comprehensive library for creating terminal applications in Node.js. It offers a wide range of features, including support for colors, styles, input handling, and more. Terminal-kit is similar to blessed in that it is designed for building terminal applications, but it provides more low-level control and flexibility. Unlike xterm, terminal-kit is not focused on web-based terminal emulation.
Node-pty is a library that provides bindings to pseudo terminals (PTYs) in Node.js. It allows you to spawn and interact with terminal processes programmatically. While node-pty does not provide a terminal emulator like xterm, it can be used in conjunction with xterm to handle the backend process management for a web-based terminal interface.
Xterm.js is a front-end component written in TypeScript that lets applications bring fully-featured terminals to their users in the browser. It's used by popular projects such as VS Code, Hyper and Theia.
bash
, vim
, and tmux
, including support for curses-based apps and mouse events.bash
. Xterm.js can be connected to processes like bash
and let you interact with them (provide input, receive output).First, you need to install the module, we ship exclusively through npm, so you need that installed and then add xterm.js as a dependency by running:
npm install xterm
To start using xterm.js on your browser, add the xterm.js
and xterm.css
to the head of your HTML page. Then create a <div id="terminal"></div>
onto which xterm can attach itself. Finally, instantiate the Terminal
object and then call the open
function with the DOM object of the div
.
<!doctype html>
<html>
<head>
<link rel="stylesheet" href="node_modules/xterm/css/xterm.css" />
<script src="node_modules/xterm/lib/xterm.js"></script>
</head>
<body>
<div id="terminal"></div>
<script>
var term = new Terminal();
term.open(document.getElementById('terminal'));
term.write('Hello from \x1B[1;3;31mxterm.js\x1B[0m $ ')
</script>
</body>
</html>
The recommended way to load xterm.js is via the ES6 module syntax:
import { Terminal } from 'xterm';
ā ļø This section describes the new addon format introduced in v3.14.0, see here for the instructions on the old format
Addons are separate modules that extend the Terminal
by building on the xterm.js API. To use an addon, you first need to install it in your project:
npm i -S xterm-addon-web-links
Then import the addon, instantiate it and call Terminal.loadAddon
:
import { Terminal } from 'xterm';
import { WebLinksAddon } from 'xterm-addon-web-links';
const terminal = new Terminal();
// Load WebLinksAddon on terminal, this is all that's needed to get web links
// working in the terminal.
terminal.loadAddon(new WebLinksAddon());
The xterm.js team maintains the following addons, but anyone can build them:
xterm-addon-attach
: Attaches to a server running a process via a websocketxterm-addon-fit
: Fits the terminal to the containing elementxterm-addon-search
: Adds search functionalityxterm-addon-web-links
: Adds web link detection and interactionSince xterm.js is typically implemented as a developer tool, only modern browsers are supported officially. Specifically the latest versions of Chrome, Edge, Firefox, and Safari.
Xterm.js works seamlessly in Electron apps and may even work on earlier versions of the browsers. These are the versions we strive to keep working.
We also publish xterm-headless
which is a stripped down version of xterm.js that runs in Node.js. An example use case for this is to keep track of a terminal's state where the process is running and using the serialize addon so it can get all state restored upon reconnection.
The full API for xterm.js is contained within the TypeScript declaration file, use the branch/tag picker in GitHub (w
) to navigate to the correct version of the API.
Note that some APIs are marked experimental, these are added to enable experimentation with new ideas without committing to support it like a normal semver API. Note that these APIs can change radically between versions, so be sure to read release notes if you plan on using experimental APIs.
Xterm.js follows a monthly release cycle roughly.
All current and past releases are available on this repo's Releases page, you can view the high-level roadmap on the wiki and see what we're working on now by looking through Milestones.
Our CI releases beta builds to npm for every change that goes into master. Install the latest beta build with:
npm install -S xterm@beta
These should generally be stable, but some bugs may slip in. We recommend using the beta build primarily to test out new features and to verify bug fixes.
You can read the guide on the wiki to learn how to contribute and set up xterm.js for development.
Xterm.js is used in several world-class applications to provide great terminal experiences.
<ng-terminal></ng-terminal>
into your component.Do you use xterm.js in your application as well? Please open a Pull Request to include it here. We would love to have it on our list. Note: Please add any new contributions to the end of the list only.
If you contribute code to this project, you implicitly allow your code to be distributed under the MIT license. You are also implicitly verifying that all code is your original work.
Copyright (c) 2017-2022, The xterm.js authors (MIT License)
Copyright (c) 2014-2017, SourceLair, Private Company (www.sourcelair.com) (MIT License)
Copyright (c) 2012-2013, Christopher Jeffrey (MIT License)
FAQs
Full xterm terminal, in your browser
The npm package xterm receives a total of 355,137 weekly downloads. As such, xterm popularity was classified as popular.
We found that xterm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Ā It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.