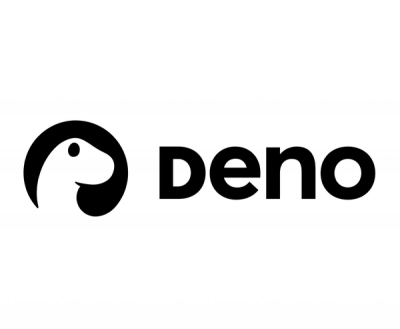
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
yaml-front-matter
Advanced tools
yaml front matter for JS. Parse yaml or JSON from the beginning of files.
Parses yaml or json at the front of a string. Places the parsed content, plus the rest of the string content, into an object literal.
This readme is for the 4.x branch which introduces breaking changes. View the changelog for more information.
This
---
name: Derek Worthen
age: young
contact:
email: email@domain.com
address: some location
pets:
- cat
- dog
- bat
match: !!js/regexp /pattern/gim
run: !!js/function function() { }
---
Some Other content
becomes
{
name: 'Derek Worthen',
age: 'young',
contact: { email: 'email@domain.com', address: 'some location' },
pets: [ 'cat', 'dog', 'bat' ],
match: /pattern/gim,
run: [Function],
__content: '\nSome Other Content'
}
May also use JSON
---
{
"name": "Derek Worthen",
"age": "young",
"anArray": ["one","two"],
"subObj":{"field1": "one"}
}
---
Some content
NOTE: The
---
are required to denote the start and end of front matter. There must be a newline after the opening---
and a newline preceding the closing---
.
$ npm install yaml-front-matter@next
Use the -g
flag if you plan on using the command line tool.
$ npm install yaml-front-matter -g
Include the client script from dist/yamlFront.js. The library will be exposed as a global, yamlFront
. The client script for js-yaml is also required. May need to load espirma for some use cases. See js-yaml for more information.
<script src="https://unpkg.com/js-yaml@3.10.0/dist/js-yaml.js"></script>
<script src="js-yaml-front-client.min.js"></script>
<script>
// parse front matter with yamlFront.loadFront(String);
</script>
$ npm install --dev && npm start
Then visit localhost:8080
.
Outputs client files in dist/
.
$ npm install --dev && npm run build
npm install --dev && npm test
Usage: yaml-front-matter [options] <file>
Options:
-h, --help output usage information
-V, --version output the version number
-c, --content [name] set the property name for the files contents [__content]
Yaml front matter wraps js-yaml to support parsing yaml front-matter.
var yamlFront = require('yaml-front-matter')
, input = [
'---\npost: title one\n',
'anArray:\n - one\n - two\n',
'subObject:\n prop1: cool\n prop2: two',
'\nreg: !!js/regexp /pattern/gim',
'\nfun: !!js/function function() { }\n---\n',
'content\nmore'
].join('');
var results = yamlFront.loadFront(input);
console.log(results);
the above will produce the following in the console.
{ post: 'title one',
anArray: [ 'one', 'two' ],
subObject: { obj1: 'cool', obj2: 'two' },
reg: /pattern/gim,
fun: [Function],
__content: '\ncontent\nmore' }
The front-matter is optional.
frontMatter.loadFront('Hello World');
Will produce
{ __content: "Hello World!" }
Content all together is optional
frontMatter.loadFront('');
// will produce { __content: '' }
Same api as loadFront except it does not support regexps, functions and undefined. See js-yaml for more information.
The options object supports the same options available to js-yaml except adds support for an additional key.
options.contentKeyName
: Specify the object key where the remaining string content after parsing the yaml front-matter will be stored. defaults to __content
.yamlFront.loadFront('Hello World', {
contentKeyName: 'fileContents'
});
// => { fileContents: "Hello World" }
FAQs
yaml front matter for JS. Parse yaml or JSON from the beginning of files.
The npm package yaml-front-matter receives a total of 90,252 weekly downloads. As such, yaml-front-matter popularity was classified as popular.
We found that yaml-front-matter demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.